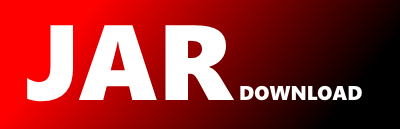
com.pulumi.aws.iam.outputs.GetServerCertificateResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iam.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetServerCertificateResult {
/**
* @return is set to the ARN of the IAM Server Certificate
*
*/
private String arn;
/**
* @return is the public key certificate (PEM-encoded). This is useful when [configuring back-end instance authentication](http://docs.aws.amazon.com/elasticloadbalancing/latest/classic/elb-create-https-ssl-load-balancer.html) policy for load balancer
*
*/
private String certificateBody;
/**
* @return is the public key certificate chain (PEM-encoded) if exists, empty otherwise
*
*/
private String certificateChain;
/**
* @return is set to the expiration date of the IAM Server Certificate
*
*/
private String expirationDate;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private @Nullable Boolean latest;
private String name;
private @Nullable String namePrefix;
/**
* @return is set to the path of the IAM Server Certificate
*
*/
private String path;
private @Nullable String pathPrefix;
/**
* @return is the date when the server certificate was uploaded
*
*/
private String uploadDate;
private GetServerCertificateResult() {}
/**
* @return is set to the ARN of the IAM Server Certificate
*
*/
public String arn() {
return this.arn;
}
/**
* @return is the public key certificate (PEM-encoded). This is useful when [configuring back-end instance authentication](http://docs.aws.amazon.com/elasticloadbalancing/latest/classic/elb-create-https-ssl-load-balancer.html) policy for load balancer
*
*/
public String certificateBody() {
return this.certificateBody;
}
/**
* @return is the public key certificate chain (PEM-encoded) if exists, empty otherwise
*
*/
public String certificateChain() {
return this.certificateChain;
}
/**
* @return is set to the expiration date of the IAM Server Certificate
*
*/
public String expirationDate() {
return this.expirationDate;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public Optional latest() {
return Optional.ofNullable(this.latest);
}
public String name() {
return this.name;
}
public Optional namePrefix() {
return Optional.ofNullable(this.namePrefix);
}
/**
* @return is set to the path of the IAM Server Certificate
*
*/
public String path() {
return this.path;
}
public Optional pathPrefix() {
return Optional.ofNullable(this.pathPrefix);
}
/**
* @return is the date when the server certificate was uploaded
*
*/
public String uploadDate() {
return this.uploadDate;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerCertificateResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String certificateBody;
private String certificateChain;
private String expirationDate;
private String id;
private @Nullable Boolean latest;
private String name;
private @Nullable String namePrefix;
private String path;
private @Nullable String pathPrefix;
private String uploadDate;
public Builder() {}
public Builder(GetServerCertificateResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.certificateBody = defaults.certificateBody;
this.certificateChain = defaults.certificateChain;
this.expirationDate = defaults.expirationDate;
this.id = defaults.id;
this.latest = defaults.latest;
this.name = defaults.name;
this.namePrefix = defaults.namePrefix;
this.path = defaults.path;
this.pathPrefix = defaults.pathPrefix;
this.uploadDate = defaults.uploadDate;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder certificateBody(String certificateBody) {
if (certificateBody == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "certificateBody");
}
this.certificateBody = certificateBody;
return this;
}
@CustomType.Setter
public Builder certificateChain(String certificateChain) {
if (certificateChain == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "certificateChain");
}
this.certificateChain = certificateChain;
return this;
}
@CustomType.Setter
public Builder expirationDate(String expirationDate) {
if (expirationDate == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "expirationDate");
}
this.expirationDate = expirationDate;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder latest(@Nullable Boolean latest) {
this.latest = latest;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder namePrefix(@Nullable String namePrefix) {
this.namePrefix = namePrefix;
return this;
}
@CustomType.Setter
public Builder path(String path) {
if (path == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "path");
}
this.path = path;
return this;
}
@CustomType.Setter
public Builder pathPrefix(@Nullable String pathPrefix) {
this.pathPrefix = pathPrefix;
return this;
}
@CustomType.Setter
public Builder uploadDate(String uploadDate) {
if (uploadDate == null) {
throw new MissingRequiredPropertyException("GetServerCertificateResult", "uploadDate");
}
this.uploadDate = uploadDate;
return this;
}
public GetServerCertificateResult build() {
final var _resultValue = new GetServerCertificateResult();
_resultValue.arn = arn;
_resultValue.certificateBody = certificateBody;
_resultValue.certificateChain = certificateChain;
_resultValue.expirationDate = expirationDate;
_resultValue.id = id;
_resultValue.latest = latest;
_resultValue.name = name;
_resultValue.namePrefix = namePrefix;
_resultValue.path = path;
_resultValue.pathPrefix = pathPrefix;
_resultValue.uploadDate = uploadDate;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy