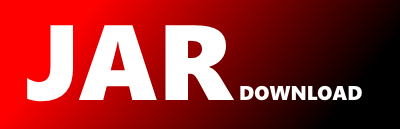
com.pulumi.aws.imagebuilder.ContainerRecipe Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.imagebuilder;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.imagebuilder.ContainerRecipeArgs;
import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeState;
import com.pulumi.aws.imagebuilder.outputs.ContainerRecipeComponent;
import com.pulumi.aws.imagebuilder.outputs.ContainerRecipeInstanceConfiguration;
import com.pulumi.aws.imagebuilder.outputs.ContainerRecipeTargetRepository;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an Image Builder Container Recipe.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.imagebuilder.ContainerRecipe;
* import com.pulumi.aws.imagebuilder.ContainerRecipeArgs;
* import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeTargetRepositoryArgs;
* import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeComponentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ContainerRecipe("example", ContainerRecipeArgs.builder()
* .name("example")
* .version("1.0.0")
* .containerType("DOCKER")
* .parentImage("arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x")
* .targetRepository(ContainerRecipeTargetRepositoryArgs.builder()
* .repositoryName(exampleAwsEcrRepository.name())
* .service("ECR")
* .build())
* .components(ContainerRecipeComponentArgs.builder()
* .componentArn(exampleAwsImagebuilderComponent.arn())
* .parameters(
* ContainerRecipeComponentParameterArgs.builder()
* .name("Parameter1")
* .value("Value1")
* .build(),
* ContainerRecipeComponentParameterArgs.builder()
* .name("Parameter2")
* .value("Value2")
* .build())
* .build())
* .dockerfileTemplateData("""
* FROM {{{ imagebuilder:parentImage }}}
* {{{ imagebuilder:environments }}}
* {{{ imagebuilder:components }}}
* """)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_imagebuilder_container_recipe` resources using the Amazon Resource Name (ARN). For example:
*
* ```sh
* $ pulumi import aws:imagebuilder/containerRecipe:ContainerRecipe example arn:aws:imagebuilder:us-east-1:123456789012:container-recipe/example/1.0.0
* ```
*
*/
@ResourceType(type="aws:imagebuilder/containerRecipe:ContainerRecipe")
public class ContainerRecipe extends com.pulumi.resources.CustomResource {
/**
* (Required) Amazon Resource Name (ARN) of the container recipe.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return (Required) Amazon Resource Name (ARN) of the container recipe.
*
*/
public Output arn() {
return this.arn;
}
/**
* Ordered configuration block(s) with components for the container recipe. Detailed below.
*
*/
@Export(name="components", refs={List.class,ContainerRecipeComponent.class}, tree="[0,1]")
private Output> components;
/**
* @return Ordered configuration block(s) with components for the container recipe. Detailed below.
*
*/
public Output> components() {
return this.components;
}
/**
* The type of the container to create. Valid values: `DOCKER`.
*
*/
@Export(name="containerType", refs={String.class}, tree="[0]")
private Output containerType;
/**
* @return The type of the container to create. Valid values: `DOCKER`.
*
*/
public Output containerType() {
return this.containerType;
}
/**
* Date the container recipe was created.
*
*/
@Export(name="dateCreated", refs={String.class}, tree="[0]")
private Output dateCreated;
/**
* @return Date the container recipe was created.
*
*/
public Output dateCreated() {
return this.dateCreated;
}
/**
* The description of the container recipe.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description of the container recipe.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The Dockerfile template used to build the image as an inline data blob.
*
*/
@Export(name="dockerfileTemplateData", refs={String.class}, tree="[0]")
private Output dockerfileTemplateData;
/**
* @return The Dockerfile template used to build the image as an inline data blob.
*
*/
public Output dockerfileTemplateData() {
return this.dockerfileTemplateData;
}
/**
* The Amazon S3 URI for the Dockerfile that will be used to build the container image.
*
*/
@Export(name="dockerfileTemplateUri", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dockerfileTemplateUri;
/**
* @return The Amazon S3 URI for the Dockerfile that will be used to build the container image.
*
*/
public Output> dockerfileTemplateUri() {
return Codegen.optional(this.dockerfileTemplateUri);
}
/**
* A flag that indicates if the target container is encrypted.
*
*/
@Export(name="encrypted", refs={Boolean.class}, tree="[0]")
private Output encrypted;
/**
* @return A flag that indicates if the target container is encrypted.
*
*/
public Output encrypted() {
return this.encrypted;
}
/**
* Configuration block used to configure an instance for building and testing container images. Detailed below.
*
*/
@Export(name="instanceConfiguration", refs={ContainerRecipeInstanceConfiguration.class}, tree="[0]")
private Output* @Nullable */ ContainerRecipeInstanceConfiguration> instanceConfiguration;
/**
* @return Configuration block used to configure an instance for building and testing container images. Detailed below.
*
*/
public Output> instanceConfiguration() {
return Codegen.optional(this.instanceConfiguration);
}
/**
* The KMS key used to encrypt the container image.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kmsKeyId;
/**
* @return The KMS key used to encrypt the container image.
*
*/
public Output> kmsKeyId() {
return Codegen.optional(this.kmsKeyId);
}
/**
* The name of the container recipe.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the container recipe.
*
*/
public Output name() {
return this.name;
}
/**
* Owner of the container recipe.
*
*/
@Export(name="owner", refs={String.class}, tree="[0]")
private Output owner;
/**
* @return Owner of the container recipe.
*
*/
public Output owner() {
return this.owner;
}
/**
* The base image for the container recipe.
*
*/
@Export(name="parentImage", refs={String.class}, tree="[0]")
private Output parentImage;
/**
* @return The base image for the container recipe.
*
*/
public Output parentImage() {
return this.parentImage;
}
/**
* Platform of the container recipe.
*
*/
@Export(name="platform", refs={String.class}, tree="[0]")
private Output platform;
/**
* @return Platform of the container recipe.
*
*/
public Output platform() {
return this.platform;
}
/**
* Specifies the operating system platform when you use a custom base image.
*
*/
@Export(name="platformOverride", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> platformOverride;
/**
* @return Specifies the operating system platform when you use a custom base image.
*
*/
public Output> platformOverride() {
return Codegen.optional(this.platformOverride);
}
/**
* Key-value map of resource tags for the container recipe. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags for the container recipe. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy