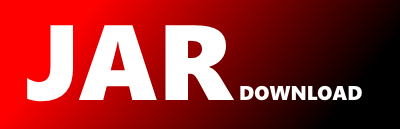
com.pulumi.aws.imagebuilder.ImageArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.imagebuilder;
import com.pulumi.aws.imagebuilder.inputs.ImageImageScanningConfigurationArgs;
import com.pulumi.aws.imagebuilder.inputs.ImageImageTestsConfigurationArgs;
import com.pulumi.aws.imagebuilder.inputs.ImageWorkflowArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ImageArgs extends com.pulumi.resources.ResourceArgs {
public static final ImageArgs Empty = new ImageArgs();
/**
* Amazon Resource Name (ARN) of the container recipe.
*
*/
@Import(name="containerRecipeArn")
private @Nullable Output containerRecipeArn;
/**
* @return Amazon Resource Name (ARN) of the container recipe.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy