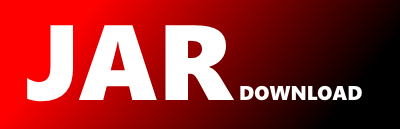
com.pulumi.aws.imagebuilder.outputs.ImageRecipeBlockDeviceMappingEbs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.imagebuilder.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ImageRecipeBlockDeviceMappingEbs {
/**
* @return Whether to delete the volume on termination. Defaults to unset, which is the value inherited from the parent image.
*
*/
private @Nullable String deleteOnTermination;
/**
* @return Whether to encrypt the volume. Defaults to unset, which is the value inherited from the parent image.
*
*/
private @Nullable String encrypted;
/**
* @return Number of Input/Output (I/O) operations per second to provision for an `io1` or `io2` volume.
*
*/
private @Nullable Integer iops;
/**
* @return Amazon Resource Name (ARN) of the Key Management Service (KMS) Key for encryption.
*
*/
private @Nullable String kmsKeyId;
/**
* @return Identifier of the EC2 Volume Snapshot.
*
*/
private @Nullable String snapshotId;
/**
* @return For GP3 volumes only. The throughput in MiB/s that the volume supports.
*
*/
private @Nullable Integer throughput;
/**
* @return Size of the volume, in GiB.
*
*/
private @Nullable Integer volumeSize;
/**
* @return Type of the volume. For example, `gp2` or `io2`.
*
*/
private @Nullable String volumeType;
private ImageRecipeBlockDeviceMappingEbs() {}
/**
* @return Whether to delete the volume on termination. Defaults to unset, which is the value inherited from the parent image.
*
*/
public Optional deleteOnTermination() {
return Optional.ofNullable(this.deleteOnTermination);
}
/**
* @return Whether to encrypt the volume. Defaults to unset, which is the value inherited from the parent image.
*
*/
public Optional encrypted() {
return Optional.ofNullable(this.encrypted);
}
/**
* @return Number of Input/Output (I/O) operations per second to provision for an `io1` or `io2` volume.
*
*/
public Optional iops() {
return Optional.ofNullable(this.iops);
}
/**
* @return Amazon Resource Name (ARN) of the Key Management Service (KMS) Key for encryption.
*
*/
public Optional kmsKeyId() {
return Optional.ofNullable(this.kmsKeyId);
}
/**
* @return Identifier of the EC2 Volume Snapshot.
*
*/
public Optional snapshotId() {
return Optional.ofNullable(this.snapshotId);
}
/**
* @return For GP3 volumes only. The throughput in MiB/s that the volume supports.
*
*/
public Optional throughput() {
return Optional.ofNullable(this.throughput);
}
/**
* @return Size of the volume, in GiB.
*
*/
public Optional volumeSize() {
return Optional.ofNullable(this.volumeSize);
}
/**
* @return Type of the volume. For example, `gp2` or `io2`.
*
*/
public Optional volumeType() {
return Optional.ofNullable(this.volumeType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ImageRecipeBlockDeviceMappingEbs defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String deleteOnTermination;
private @Nullable String encrypted;
private @Nullable Integer iops;
private @Nullable String kmsKeyId;
private @Nullable String snapshotId;
private @Nullable Integer throughput;
private @Nullable Integer volumeSize;
private @Nullable String volumeType;
public Builder() {}
public Builder(ImageRecipeBlockDeviceMappingEbs defaults) {
Objects.requireNonNull(defaults);
this.deleteOnTermination = defaults.deleteOnTermination;
this.encrypted = defaults.encrypted;
this.iops = defaults.iops;
this.kmsKeyId = defaults.kmsKeyId;
this.snapshotId = defaults.snapshotId;
this.throughput = defaults.throughput;
this.volumeSize = defaults.volumeSize;
this.volumeType = defaults.volumeType;
}
@CustomType.Setter
public Builder deleteOnTermination(@Nullable String deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
return this;
}
@CustomType.Setter
public Builder encrypted(@Nullable String encrypted) {
this.encrypted = encrypted;
return this;
}
@CustomType.Setter
public Builder iops(@Nullable Integer iops) {
this.iops = iops;
return this;
}
@CustomType.Setter
public Builder kmsKeyId(@Nullable String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
return this;
}
@CustomType.Setter
public Builder snapshotId(@Nullable String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
@CustomType.Setter
public Builder throughput(@Nullable Integer throughput) {
this.throughput = throughput;
return this;
}
@CustomType.Setter
public Builder volumeSize(@Nullable Integer volumeSize) {
this.volumeSize = volumeSize;
return this;
}
@CustomType.Setter
public Builder volumeType(@Nullable String volumeType) {
this.volumeType = volumeType;
return this;
}
public ImageRecipeBlockDeviceMappingEbs build() {
final var _resultValue = new ImageRecipeBlockDeviceMappingEbs();
_resultValue.deleteOnTermination = deleteOnTermination;
_resultValue.encrypted = encrypted;
_resultValue.iops = iops;
_resultValue.kmsKeyId = kmsKeyId;
_resultValue.snapshotId = snapshotId;
_resultValue.throughput = throughput;
_resultValue.volumeSize = volumeSize;
_resultValue.volumeType = volumeType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy