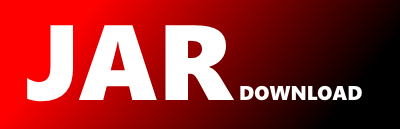
com.pulumi.aws.lambda.Invocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lambda;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.lambda.InvocationArgs;
import com.pulumi.aws.lambda.inputs.InvocationState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Use this resource to invoke a lambda function. The lambda function is invoked with the [RequestResponse](https://docs.aws.amazon.com/lambda/latest/dg/API_Invoke.html#API_Invoke_RequestSyntax) invocation type.
*
* > **NOTE:** By default this resource _only_ invokes the function when the arguments call for a create or replace. In other words, after an initial invocation on _apply_, if the arguments do not change, a subsequent _apply_ does not invoke the function again. To dynamically invoke the function, see the `triggers` example below. To always invoke a function on each _apply_, see the `aws.lambda.Invocation` data source. To invoke the lambda function when the Pulumi resource is updated and deleted, see the CRUD Lifecycle Scope example below.
*
* > **NOTE:** If you get a `KMSAccessDeniedException: Lambda was unable to decrypt the environment variables because KMS access was denied` error when invoking an `aws.lambda.Function` with environment variables, the IAM role associated with the function may have been deleted and recreated _after_ the function was created. You can fix the problem two ways: 1) updating the function's role to another role and then updating it back again to the recreated role, or 2) by using Pulumi to `taint` the function and `apply` your configuration again to recreate the function. (When you create a function, Lambda grants permissions on the KMS key to the function's IAM role. If the IAM role is recreated, the grant is no longer valid. Changing the function's role or recreating the function causes Lambda to update the grant.)
*
* ## Example Usage
*
* ### Dynamic Invocation Example Using Triggers
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Invocation;
* import com.pulumi.aws.lambda.InvocationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Invocation("example", InvocationArgs.builder()
* .functionName(lambdaFunctionTest.functionName())
* .triggers(Map.of("redeployment", StdFunctions.sha1(Sha1Args.builder()
* .input(serializeJson(
* jsonArray(exampleAwsLambdaFunction.environment())))
* .build()).result()))
* .input(serializeJson(
* jsonObject(
* jsonProperty("key1", "value1"),
* jsonProperty("key2", "value2")
* )))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### CRUD Lifecycle Scope
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Invocation;
* import com.pulumi.aws.lambda.InvocationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Invocation("example", InvocationArgs.builder()
* .functionName(lambdaFunctionTest.functionName())
* .input(serializeJson(
* jsonObject(
* jsonProperty("key1", "value1"),
* jsonProperty("key2", "value2")
* )))
* .lifecycleScope("CRUD")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* > **NOTE:** `lifecycle_scope = "CRUD"` will inject a key `tf` in the input event to pass lifecycle information! This allows the lambda function to handle different lifecycle transitions uniquely. If you need to use a key `tf` in your own input JSON, the default key name can be overridden with the `pulumi_key` argument.
*
* The key `tf` gets added with subkeys:
*
* * `action` - Action Pulumi performs on the resource. Values are `create`, `update`, or `delete`.
* * `prev_input` - Input JSON payload from the previous invocation. This can be used to handle update and delete events.
*
* When the resource from the example above is created, the Lambda will get following JSON payload:
*
* If the input value of `key1` changes to "valueB", then the lambda will be invoked again with the following JSON payload:
*
* When the invocation resource is removed, the final invocation will have the following JSON payload:
*
*/
@ResourceType(type="aws:lambda/invocation:Invocation")
public class Invocation extends com.pulumi.resources.CustomResource {
/**
* Name of the lambda function.
*
*/
@Export(name="functionName", refs={String.class}, tree="[0]")
private Output functionName;
/**
* @return Name of the lambda function.
*
*/
public Output functionName() {
return this.functionName;
}
/**
* JSON payload to the lambda function.
*
* The following arguments are optional:
*
*/
@Export(name="input", refs={String.class}, tree="[0]")
private Output input;
/**
* @return JSON payload to the lambda function.
*
* The following arguments are optional:
*
*/
public Output input() {
return this.input;
}
/**
* Lifecycle scope of the resource to manage. Valid values are `CREATE_ONLY` and `CRUD`. Defaults to `CREATE_ONLY`. `CREATE_ONLY` will invoke the function only on creation or replacement. `CRUD` will invoke the function on each lifecycle event, and augment the input JSON payload with additional lifecycle information.
*
*/
@Export(name="lifecycleScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lifecycleScope;
/**
* @return Lifecycle scope of the resource to manage. Valid values are `CREATE_ONLY` and `CRUD`. Defaults to `CREATE_ONLY`. `CREATE_ONLY` will invoke the function only on creation or replacement. `CRUD` will invoke the function on each lifecycle event, and augment the input JSON payload with additional lifecycle information.
*
*/
public Output> lifecycleScope() {
return Codegen.optional(this.lifecycleScope);
}
/**
* Qualifier (i.e., version) of the lambda function. Defaults to `$LATEST`.
*
*/
@Export(name="qualifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> qualifier;
/**
* @return Qualifier (i.e., version) of the lambda function. Defaults to `$LATEST`.
*
*/
public Output> qualifier() {
return Codegen.optional(this.qualifier);
}
/**
* String result of the lambda function invocation.
*
*/
@Export(name="result", refs={String.class}, tree="[0]")
private Output result;
/**
* @return String result of the lambda function invocation.
*
*/
public Output result() {
return this.result;
}
@Export(name="terraformKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> terraformKey;
public Output> terraformKey() {
return Codegen.optional(this.terraformKey);
}
/**
* Map of arbitrary keys and values that, when changed, will trigger a re-invocation.
*
*/
@Export(name="triggers", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> triggers;
/**
* @return Map of arbitrary keys and values that, when changed, will trigger a re-invocation.
*
*/
public Output>> triggers() {
return Codegen.optional(this.triggers);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Invocation(java.lang.String name) {
this(name, InvocationArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Invocation(java.lang.String name, InvocationArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Invocation(java.lang.String name, InvocationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:lambda/invocation:Invocation", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Invocation(java.lang.String name, Output id, @Nullable InvocationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:lambda/invocation:Invocation", name, state, makeResourceOptions(options, id), false);
}
private static InvocationArgs makeArgs(InvocationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? InvocationArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Invocation get(java.lang.String name, Output id, @Nullable InvocationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Invocation(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy