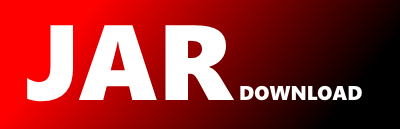
com.pulumi.aws.lex.outputs.IntentSlot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.outputs;
import com.pulumi.aws.lex.outputs.IntentSlotValueElicitationPrompt;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IntentSlot {
/**
* @return A description of the bot. Must be less than or equal to 200 characters in length.
*
*/
private @Nullable String description;
/**
* @return The name of the intent slot that you want to create. The name is case sensitive. Must be less than or equal to 100 characters in length.
*
*/
private String name;
/**
* @return Directs Lex the order in which to elicit this slot value from the user.
* For example, if the intent has two slots with priorities 1 and 2, AWS Lex first elicits a value for
* the slot with priority 1. If multiple slots share the same priority, the order in which Lex elicits
* values is arbitrary. Must be between 1 and 100.
*
*/
private @Nullable Integer priority;
/**
* @return The response card. Amazon Lex will substitute session attributes and
* slot values into the response card. For more information, see
* [Example: Using a Response Card](https://docs.aws.amazon.com/lex/latest/dg/ex-resp-card.html). Must be less than or equal to 50000 characters in length.
*
*/
private @Nullable String responseCard;
/**
* @return If you know a specific pattern with which users might respond to
* an Amazon Lex request for a slot value, you can provide those utterances to improve accuracy. This
* is optional. In most cases, Amazon Lex is capable of understanding user utterances. Must have between 1 and 10 items in the list, and each item must be less than or equal to 200 characters in length.
*
*/
private @Nullable List sampleUtterances;
/**
* @return Specifies whether the slot is required or optional.
*
*/
private String slotConstraint;
/**
* @return The type of the slot, either a custom slot type that you defined or one of
* the built-in slot types. Must be less than or equal to 100 characters in length.
*
*/
private String slotType;
/**
* @return The version of the slot type. Must be less than or equal to 64 characters in length.
*
*/
private @Nullable String slotTypeVersion;
/**
* @return The prompt that Amazon Lex uses to elicit the slot value
* from the user. Attributes are documented under prompt.
*
*/
private @Nullable IntentSlotValueElicitationPrompt valueElicitationPrompt;
private IntentSlot() {}
/**
* @return A description of the bot. Must be less than or equal to 200 characters in length.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The name of the intent slot that you want to create. The name is case sensitive. Must be less than or equal to 100 characters in length.
*
*/
public String name() {
return this.name;
}
/**
* @return Directs Lex the order in which to elicit this slot value from the user.
* For example, if the intent has two slots with priorities 1 and 2, AWS Lex first elicits a value for
* the slot with priority 1. If multiple slots share the same priority, the order in which Lex elicits
* values is arbitrary. Must be between 1 and 100.
*
*/
public Optional priority() {
return Optional.ofNullable(this.priority);
}
/**
* @return The response card. Amazon Lex will substitute session attributes and
* slot values into the response card. For more information, see
* [Example: Using a Response Card](https://docs.aws.amazon.com/lex/latest/dg/ex-resp-card.html). Must be less than or equal to 50000 characters in length.
*
*/
public Optional responseCard() {
return Optional.ofNullable(this.responseCard);
}
/**
* @return If you know a specific pattern with which users might respond to
* an Amazon Lex request for a slot value, you can provide those utterances to improve accuracy. This
* is optional. In most cases, Amazon Lex is capable of understanding user utterances. Must have between 1 and 10 items in the list, and each item must be less than or equal to 200 characters in length.
*
*/
public List sampleUtterances() {
return this.sampleUtterances == null ? List.of() : this.sampleUtterances;
}
/**
* @return Specifies whether the slot is required or optional.
*
*/
public String slotConstraint() {
return this.slotConstraint;
}
/**
* @return The type of the slot, either a custom slot type that you defined or one of
* the built-in slot types. Must be less than or equal to 100 characters in length.
*
*/
public String slotType() {
return this.slotType;
}
/**
* @return The version of the slot type. Must be less than or equal to 64 characters in length.
*
*/
public Optional slotTypeVersion() {
return Optional.ofNullable(this.slotTypeVersion);
}
/**
* @return The prompt that Amazon Lex uses to elicit the slot value
* from the user. Attributes are documented under prompt.
*
*/
public Optional valueElicitationPrompt() {
return Optional.ofNullable(this.valueElicitationPrompt);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IntentSlot defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String description;
private String name;
private @Nullable Integer priority;
private @Nullable String responseCard;
private @Nullable List sampleUtterances;
private String slotConstraint;
private String slotType;
private @Nullable String slotTypeVersion;
private @Nullable IntentSlotValueElicitationPrompt valueElicitationPrompt;
public Builder() {}
public Builder(IntentSlot defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.name = defaults.name;
this.priority = defaults.priority;
this.responseCard = defaults.responseCard;
this.sampleUtterances = defaults.sampleUtterances;
this.slotConstraint = defaults.slotConstraint;
this.slotType = defaults.slotType;
this.slotTypeVersion = defaults.slotTypeVersion;
this.valueElicitationPrompt = defaults.valueElicitationPrompt;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("IntentSlot", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(@Nullable Integer priority) {
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder responseCard(@Nullable String responseCard) {
this.responseCard = responseCard;
return this;
}
@CustomType.Setter
public Builder sampleUtterances(@Nullable List sampleUtterances) {
this.sampleUtterances = sampleUtterances;
return this;
}
public Builder sampleUtterances(String... sampleUtterances) {
return sampleUtterances(List.of(sampleUtterances));
}
@CustomType.Setter
public Builder slotConstraint(String slotConstraint) {
if (slotConstraint == null) {
throw new MissingRequiredPropertyException("IntentSlot", "slotConstraint");
}
this.slotConstraint = slotConstraint;
return this;
}
@CustomType.Setter
public Builder slotType(String slotType) {
if (slotType == null) {
throw new MissingRequiredPropertyException("IntentSlot", "slotType");
}
this.slotType = slotType;
return this;
}
@CustomType.Setter
public Builder slotTypeVersion(@Nullable String slotTypeVersion) {
this.slotTypeVersion = slotTypeVersion;
return this;
}
@CustomType.Setter
public Builder valueElicitationPrompt(@Nullable IntentSlotValueElicitationPrompt valueElicitationPrompt) {
this.valueElicitationPrompt = valueElicitationPrompt;
return this;
}
public IntentSlot build() {
final var _resultValue = new IntentSlot();
_resultValue.description = description;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.responseCard = responseCard;
_resultValue.sampleUtterances = sampleUtterances;
_resultValue.slotConstraint = slotConstraint;
_resultValue.slotType = slotType;
_resultValue.slotTypeVersion = slotTypeVersion;
_resultValue.valueElicitationPrompt = valueElicitationPrompt;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy