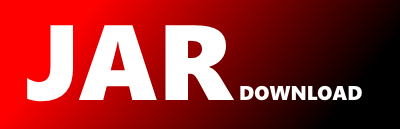
com.pulumi.aws.lex.outputs.V2modelsIntentConfirmationSettingPromptSpecification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.outputs;
import com.pulumi.aws.lex.outputs.V2modelsIntentConfirmationSettingPromptSpecificationMessageGroup;
import com.pulumi.aws.lex.outputs.V2modelsIntentConfirmationSettingPromptSpecificationPromptAttemptsSpecification;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class V2modelsIntentConfirmationSettingPromptSpecification {
/**
* @return Whether the user can interrupt a speech prompt from the bot.
*
*/
private @Nullable Boolean allowInterrupt;
/**
* @return Maximum number of times the bot tries to elicit a response from the user using this prompt.
*
*/
private Integer maxRetries;
/**
* @return Configuration block for messages that Amazon Lex can send to the user. Amazon Lex chooses the actual message to send at runtime. See `message_group`.
*
*/
private @Nullable List messageGroups;
/**
* @return How a message is selected from a message group among retries. Valid values are `Random` and `Ordered`.
*
*/
private @Nullable String messageSelectionStrategy;
/**
* @return Configuration block for advanced settings on each attempt of the prompt. See `prompt_attempts_specification`.
*
*/
private @Nullable List promptAttemptsSpecifications;
private V2modelsIntentConfirmationSettingPromptSpecification() {}
/**
* @return Whether the user can interrupt a speech prompt from the bot.
*
*/
public Optional allowInterrupt() {
return Optional.ofNullable(this.allowInterrupt);
}
/**
* @return Maximum number of times the bot tries to elicit a response from the user using this prompt.
*
*/
public Integer maxRetries() {
return this.maxRetries;
}
/**
* @return Configuration block for messages that Amazon Lex can send to the user. Amazon Lex chooses the actual message to send at runtime. See `message_group`.
*
*/
public List messageGroups() {
return this.messageGroups == null ? List.of() : this.messageGroups;
}
/**
* @return How a message is selected from a message group among retries. Valid values are `Random` and `Ordered`.
*
*/
public Optional messageSelectionStrategy() {
return Optional.ofNullable(this.messageSelectionStrategy);
}
/**
* @return Configuration block for advanced settings on each attempt of the prompt. See `prompt_attempts_specification`.
*
*/
public List promptAttemptsSpecifications() {
return this.promptAttemptsSpecifications == null ? List.of() : this.promptAttemptsSpecifications;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(V2modelsIntentConfirmationSettingPromptSpecification defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowInterrupt;
private Integer maxRetries;
private @Nullable List messageGroups;
private @Nullable String messageSelectionStrategy;
private @Nullable List promptAttemptsSpecifications;
public Builder() {}
public Builder(V2modelsIntentConfirmationSettingPromptSpecification defaults) {
Objects.requireNonNull(defaults);
this.allowInterrupt = defaults.allowInterrupt;
this.maxRetries = defaults.maxRetries;
this.messageGroups = defaults.messageGroups;
this.messageSelectionStrategy = defaults.messageSelectionStrategy;
this.promptAttemptsSpecifications = defaults.promptAttemptsSpecifications;
}
@CustomType.Setter
public Builder allowInterrupt(@Nullable Boolean allowInterrupt) {
this.allowInterrupt = allowInterrupt;
return this;
}
@CustomType.Setter
public Builder maxRetries(Integer maxRetries) {
if (maxRetries == null) {
throw new MissingRequiredPropertyException("V2modelsIntentConfirmationSettingPromptSpecification", "maxRetries");
}
this.maxRetries = maxRetries;
return this;
}
@CustomType.Setter
public Builder messageGroups(@Nullable List messageGroups) {
this.messageGroups = messageGroups;
return this;
}
public Builder messageGroups(V2modelsIntentConfirmationSettingPromptSpecificationMessageGroup... messageGroups) {
return messageGroups(List.of(messageGroups));
}
@CustomType.Setter
public Builder messageSelectionStrategy(@Nullable String messageSelectionStrategy) {
this.messageSelectionStrategy = messageSelectionStrategy;
return this;
}
@CustomType.Setter
public Builder promptAttemptsSpecifications(@Nullable List promptAttemptsSpecifications) {
this.promptAttemptsSpecifications = promptAttemptsSpecifications;
return this;
}
public Builder promptAttemptsSpecifications(V2modelsIntentConfirmationSettingPromptSpecificationPromptAttemptsSpecification... promptAttemptsSpecifications) {
return promptAttemptsSpecifications(List.of(promptAttemptsSpecifications));
}
public V2modelsIntentConfirmationSettingPromptSpecification build() {
final var _resultValue = new V2modelsIntentConfirmationSettingPromptSpecification();
_resultValue.allowInterrupt = allowInterrupt;
_resultValue.maxRetries = maxRetries;
_resultValue.messageGroups = messageGroups;
_resultValue.messageSelectionStrategy = messageSelectionStrategy;
_resultValue.promptAttemptsSpecifications = promptAttemptsSpecifications;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy