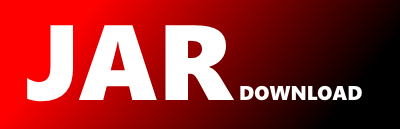
com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.licensemanager.outputs;
import com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseConsumptionConfiguration;
import com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseEntitlement;
import com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseIssuer;
import com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseLicenseMetadata;
import com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseReceivedMetadata;
import com.pulumi.aws.licensemanager.outputs.GetReceivedLicenseValidity;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetReceivedLicenseResult {
/**
* @return Granted license beneficiary. This is in the form of the ARN of the root user of the account.
*
*/
private String beneficiary;
/**
* @return Configuration for consumption of the license. Detailed below
*
*/
private List consumptionConfigurations;
/**
* @return Creation time of the granted license in RFC 3339 format.
*
*/
private String createTime;
/**
* @return License entitlements. Detailed below
*
*/
private List entitlements;
/**
* @return Home Region of the granted license.
*
*/
private String homeRegion;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Granted license issuer. Detailed below
*
*/
private List issuers;
/**
* @return Amazon Resource Name (ARN) of the license.
*
*/
private String licenseArn;
/**
* @return Granted license metadata. This is in the form of a set of all meta data. Detailed below
*
*/
private List licenseMetadatas;
/**
* @return License name.
*
*/
private String licenseName;
/**
* @return Product name.
* * ` product_sku ` - Product SKU.
*
*/
private String productName;
private String productSku;
/**
* @return Granted license received metadata. Detailed below
*
*/
private List receivedMetadatas;
/**
* @return Granted license status.
*
*/
private String status;
/**
* @return Date and time range during which the granted license is valid, in ISO8601-UTC format. Detailed below
*
*/
private List validities;
/**
* @return Version of the granted license.
*
*/
private String version;
private GetReceivedLicenseResult() {}
/**
* @return Granted license beneficiary. This is in the form of the ARN of the root user of the account.
*
*/
public String beneficiary() {
return this.beneficiary;
}
/**
* @return Configuration for consumption of the license. Detailed below
*
*/
public List consumptionConfigurations() {
return this.consumptionConfigurations;
}
/**
* @return Creation time of the granted license in RFC 3339 format.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return License entitlements. Detailed below
*
*/
public List entitlements() {
return this.entitlements;
}
/**
* @return Home Region of the granted license.
*
*/
public String homeRegion() {
return this.homeRegion;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Granted license issuer. Detailed below
*
*/
public List issuers() {
return this.issuers;
}
/**
* @return Amazon Resource Name (ARN) of the license.
*
*/
public String licenseArn() {
return this.licenseArn;
}
/**
* @return Granted license metadata. This is in the form of a set of all meta data. Detailed below
*
*/
public List licenseMetadatas() {
return this.licenseMetadatas;
}
/**
* @return License name.
*
*/
public String licenseName() {
return this.licenseName;
}
/**
* @return Product name.
* * ` product_sku ` - Product SKU.
*
*/
public String productName() {
return this.productName;
}
public String productSku() {
return this.productSku;
}
/**
* @return Granted license received metadata. Detailed below
*
*/
public List receivedMetadatas() {
return this.receivedMetadatas;
}
/**
* @return Granted license status.
*
*/
public String status() {
return this.status;
}
/**
* @return Date and time range during which the granted license is valid, in ISO8601-UTC format. Detailed below
*
*/
public List validities() {
return this.validities;
}
/**
* @return Version of the granted license.
*
*/
public String version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReceivedLicenseResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String beneficiary;
private List consumptionConfigurations;
private String createTime;
private List entitlements;
private String homeRegion;
private String id;
private List issuers;
private String licenseArn;
private List licenseMetadatas;
private String licenseName;
private String productName;
private String productSku;
private List receivedMetadatas;
private String status;
private List validities;
private String version;
public Builder() {}
public Builder(GetReceivedLicenseResult defaults) {
Objects.requireNonNull(defaults);
this.beneficiary = defaults.beneficiary;
this.consumptionConfigurations = defaults.consumptionConfigurations;
this.createTime = defaults.createTime;
this.entitlements = defaults.entitlements;
this.homeRegion = defaults.homeRegion;
this.id = defaults.id;
this.issuers = defaults.issuers;
this.licenseArn = defaults.licenseArn;
this.licenseMetadatas = defaults.licenseMetadatas;
this.licenseName = defaults.licenseName;
this.productName = defaults.productName;
this.productSku = defaults.productSku;
this.receivedMetadatas = defaults.receivedMetadatas;
this.status = defaults.status;
this.validities = defaults.validities;
this.version = defaults.version;
}
@CustomType.Setter
public Builder beneficiary(String beneficiary) {
if (beneficiary == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "beneficiary");
}
this.beneficiary = beneficiary;
return this;
}
@CustomType.Setter
public Builder consumptionConfigurations(List consumptionConfigurations) {
if (consumptionConfigurations == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "consumptionConfigurations");
}
this.consumptionConfigurations = consumptionConfigurations;
return this;
}
public Builder consumptionConfigurations(GetReceivedLicenseConsumptionConfiguration... consumptionConfigurations) {
return consumptionConfigurations(List.of(consumptionConfigurations));
}
@CustomType.Setter
public Builder createTime(String createTime) {
if (createTime == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "createTime");
}
this.createTime = createTime;
return this;
}
@CustomType.Setter
public Builder entitlements(List entitlements) {
if (entitlements == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "entitlements");
}
this.entitlements = entitlements;
return this;
}
public Builder entitlements(GetReceivedLicenseEntitlement... entitlements) {
return entitlements(List.of(entitlements));
}
@CustomType.Setter
public Builder homeRegion(String homeRegion) {
if (homeRegion == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "homeRegion");
}
this.homeRegion = homeRegion;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder issuers(List issuers) {
if (issuers == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "issuers");
}
this.issuers = issuers;
return this;
}
public Builder issuers(GetReceivedLicenseIssuer... issuers) {
return issuers(List.of(issuers));
}
@CustomType.Setter
public Builder licenseArn(String licenseArn) {
if (licenseArn == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "licenseArn");
}
this.licenseArn = licenseArn;
return this;
}
@CustomType.Setter
public Builder licenseMetadatas(List licenseMetadatas) {
if (licenseMetadatas == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "licenseMetadatas");
}
this.licenseMetadatas = licenseMetadatas;
return this;
}
public Builder licenseMetadatas(GetReceivedLicenseLicenseMetadata... licenseMetadatas) {
return licenseMetadatas(List.of(licenseMetadatas));
}
@CustomType.Setter
public Builder licenseName(String licenseName) {
if (licenseName == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "licenseName");
}
this.licenseName = licenseName;
return this;
}
@CustomType.Setter
public Builder productName(String productName) {
if (productName == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "productName");
}
this.productName = productName;
return this;
}
@CustomType.Setter
public Builder productSku(String productSku) {
if (productSku == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "productSku");
}
this.productSku = productSku;
return this;
}
@CustomType.Setter
public Builder receivedMetadatas(List receivedMetadatas) {
if (receivedMetadatas == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "receivedMetadatas");
}
this.receivedMetadatas = receivedMetadatas;
return this;
}
public Builder receivedMetadatas(GetReceivedLicenseReceivedMetadata... receivedMetadatas) {
return receivedMetadatas(List.of(receivedMetadatas));
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder validities(List validities) {
if (validities == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "validities");
}
this.validities = validities;
return this;
}
public Builder validities(GetReceivedLicenseValidity... validities) {
return validities(List.of(validities));
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("GetReceivedLicenseResult", "version");
}
this.version = version;
return this;
}
public GetReceivedLicenseResult build() {
final var _resultValue = new GetReceivedLicenseResult();
_resultValue.beneficiary = beneficiary;
_resultValue.consumptionConfigurations = consumptionConfigurations;
_resultValue.createTime = createTime;
_resultValue.entitlements = entitlements;
_resultValue.homeRegion = homeRegion;
_resultValue.id = id;
_resultValue.issuers = issuers;
_resultValue.licenseArn = licenseArn;
_resultValue.licenseMetadatas = licenseMetadatas;
_resultValue.licenseName = licenseName;
_resultValue.productName = productName;
_resultValue.productSku = productSku;
_resultValue.receivedMetadatas = receivedMetadatas;
_resultValue.status = status;
_resultValue.validities = validities;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy