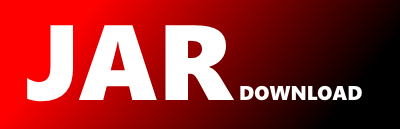
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH264SettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.medialive.inputs;
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH264SettingsFilterSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ChannelEncoderSettingsVideoDescriptionCodecSettingsH264SettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final ChannelEncoderSettingsVideoDescriptionCodecSettingsH264SettingsArgs Empty = new ChannelEncoderSettingsVideoDescriptionCodecSettingsH264SettingsArgs();
/**
* Enables or disables adaptive quantization.
*
*/
@Import(name="adaptiveQuantization")
private @Nullable Output adaptiveQuantization;
/**
* @return Enables or disables adaptive quantization.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy