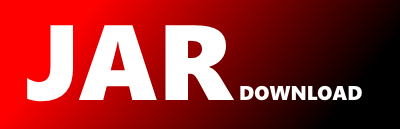
com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.medialive.outputs;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbNitSettings;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbSdtSettings;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbTdtSettings;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings {
private @Nullable String absentInputAudioBehavior;
private @Nullable String arib;
private @Nullable String aribCaptionsPid;
private @Nullable String aribCaptionsPidControl;
private @Nullable String audioBufferModel;
private @Nullable Integer audioFramesPerPes;
private @Nullable String audioPids;
private @Nullable String audioStreamType;
private @Nullable Integer bitrate;
private @Nullable String bufferModel;
private @Nullable String ccDescriptor;
private @Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbNitSettings dvbNitSettings;
private @Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbSdtSettings dvbSdtSettings;
private @Nullable String dvbSubPids;
private @Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbTdtSettings dvbTdtSettings;
private @Nullable String dvbTeletextPid;
private @Nullable String ebif;
private @Nullable String ebpAudioInterval;
private @Nullable Integer ebpLookaheadMs;
private @Nullable String ebpPlacement;
private @Nullable String ecmPid;
private @Nullable String esRateInPes;
private @Nullable String etvPlatformPid;
private @Nullable String etvSignalPid;
private @Nullable Double fragmentTime;
private @Nullable String klv;
private @Nullable String klvDataPids;
private @Nullable String nielsenId3Behavior;
private @Nullable Double nullPacketBitrate;
private @Nullable Integer patInterval;
private @Nullable String pcrControl;
private @Nullable Integer pcrPeriod;
private @Nullable String pcrPid;
private @Nullable Integer pmtInterval;
private @Nullable String pmtPid;
private @Nullable Integer programNum;
private @Nullable String rateMode;
private @Nullable String scte27Pids;
private @Nullable String scte35Control;
/**
* @return PID from which to read SCTE-35 messages.
*
*/
private @Nullable String scte35Pid;
private @Nullable String segmentationMarkers;
private @Nullable String segmentationStyle;
private @Nullable Double segmentationTime;
private @Nullable String timedMetadataBehavior;
private @Nullable String timedMetadataPid;
private @Nullable Integer transportStreamId;
private @Nullable String videoPid;
private ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings() {}
public Optional absentInputAudioBehavior() {
return Optional.ofNullable(this.absentInputAudioBehavior);
}
public Optional arib() {
return Optional.ofNullable(this.arib);
}
public Optional aribCaptionsPid() {
return Optional.ofNullable(this.aribCaptionsPid);
}
public Optional aribCaptionsPidControl() {
return Optional.ofNullable(this.aribCaptionsPidControl);
}
public Optional audioBufferModel() {
return Optional.ofNullable(this.audioBufferModel);
}
public Optional audioFramesPerPes() {
return Optional.ofNullable(this.audioFramesPerPes);
}
public Optional audioPids() {
return Optional.ofNullable(this.audioPids);
}
public Optional audioStreamType() {
return Optional.ofNullable(this.audioStreamType);
}
public Optional bitrate() {
return Optional.ofNullable(this.bitrate);
}
public Optional bufferModel() {
return Optional.ofNullable(this.bufferModel);
}
public Optional ccDescriptor() {
return Optional.ofNullable(this.ccDescriptor);
}
public Optional dvbNitSettings() {
return Optional.ofNullable(this.dvbNitSettings);
}
public Optional dvbSdtSettings() {
return Optional.ofNullable(this.dvbSdtSettings);
}
public Optional dvbSubPids() {
return Optional.ofNullable(this.dvbSubPids);
}
public Optional dvbTdtSettings() {
return Optional.ofNullable(this.dvbTdtSettings);
}
public Optional dvbTeletextPid() {
return Optional.ofNullable(this.dvbTeletextPid);
}
public Optional ebif() {
return Optional.ofNullable(this.ebif);
}
public Optional ebpAudioInterval() {
return Optional.ofNullable(this.ebpAudioInterval);
}
public Optional ebpLookaheadMs() {
return Optional.ofNullable(this.ebpLookaheadMs);
}
public Optional ebpPlacement() {
return Optional.ofNullable(this.ebpPlacement);
}
public Optional ecmPid() {
return Optional.ofNullable(this.ecmPid);
}
public Optional esRateInPes() {
return Optional.ofNullable(this.esRateInPes);
}
public Optional etvPlatformPid() {
return Optional.ofNullable(this.etvPlatformPid);
}
public Optional etvSignalPid() {
return Optional.ofNullable(this.etvSignalPid);
}
public Optional fragmentTime() {
return Optional.ofNullable(this.fragmentTime);
}
public Optional klv() {
return Optional.ofNullable(this.klv);
}
public Optional klvDataPids() {
return Optional.ofNullable(this.klvDataPids);
}
public Optional nielsenId3Behavior() {
return Optional.ofNullable(this.nielsenId3Behavior);
}
public Optional nullPacketBitrate() {
return Optional.ofNullable(this.nullPacketBitrate);
}
public Optional patInterval() {
return Optional.ofNullable(this.patInterval);
}
public Optional pcrControl() {
return Optional.ofNullable(this.pcrControl);
}
public Optional pcrPeriod() {
return Optional.ofNullable(this.pcrPeriod);
}
public Optional pcrPid() {
return Optional.ofNullable(this.pcrPid);
}
public Optional pmtInterval() {
return Optional.ofNullable(this.pmtInterval);
}
public Optional pmtPid() {
return Optional.ofNullable(this.pmtPid);
}
public Optional programNum() {
return Optional.ofNullable(this.programNum);
}
public Optional rateMode() {
return Optional.ofNullable(this.rateMode);
}
public Optional scte27Pids() {
return Optional.ofNullable(this.scte27Pids);
}
public Optional scte35Control() {
return Optional.ofNullable(this.scte35Control);
}
/**
* @return PID from which to read SCTE-35 messages.
*
*/
public Optional scte35Pid() {
return Optional.ofNullable(this.scte35Pid);
}
public Optional segmentationMarkers() {
return Optional.ofNullable(this.segmentationMarkers);
}
public Optional segmentationStyle() {
return Optional.ofNullable(this.segmentationStyle);
}
public Optional segmentationTime() {
return Optional.ofNullable(this.segmentationTime);
}
public Optional timedMetadataBehavior() {
return Optional.ofNullable(this.timedMetadataBehavior);
}
public Optional timedMetadataPid() {
return Optional.ofNullable(this.timedMetadataPid);
}
public Optional transportStreamId() {
return Optional.ofNullable(this.transportStreamId);
}
public Optional videoPid() {
return Optional.ofNullable(this.videoPid);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String absentInputAudioBehavior;
private @Nullable String arib;
private @Nullable String aribCaptionsPid;
private @Nullable String aribCaptionsPidControl;
private @Nullable String audioBufferModel;
private @Nullable Integer audioFramesPerPes;
private @Nullable String audioPids;
private @Nullable String audioStreamType;
private @Nullable Integer bitrate;
private @Nullable String bufferModel;
private @Nullable String ccDescriptor;
private @Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbNitSettings dvbNitSettings;
private @Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbSdtSettings dvbSdtSettings;
private @Nullable String dvbSubPids;
private @Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbTdtSettings dvbTdtSettings;
private @Nullable String dvbTeletextPid;
private @Nullable String ebif;
private @Nullable String ebpAudioInterval;
private @Nullable Integer ebpLookaheadMs;
private @Nullable String ebpPlacement;
private @Nullable String ecmPid;
private @Nullable String esRateInPes;
private @Nullable String etvPlatformPid;
private @Nullable String etvSignalPid;
private @Nullable Double fragmentTime;
private @Nullable String klv;
private @Nullable String klvDataPids;
private @Nullable String nielsenId3Behavior;
private @Nullable Double nullPacketBitrate;
private @Nullable Integer patInterval;
private @Nullable String pcrControl;
private @Nullable Integer pcrPeriod;
private @Nullable String pcrPid;
private @Nullable Integer pmtInterval;
private @Nullable String pmtPid;
private @Nullable Integer programNum;
private @Nullable String rateMode;
private @Nullable String scte27Pids;
private @Nullable String scte35Control;
private @Nullable String scte35Pid;
private @Nullable String segmentationMarkers;
private @Nullable String segmentationStyle;
private @Nullable Double segmentationTime;
private @Nullable String timedMetadataBehavior;
private @Nullable String timedMetadataPid;
private @Nullable Integer transportStreamId;
private @Nullable String videoPid;
public Builder() {}
public Builder(ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings defaults) {
Objects.requireNonNull(defaults);
this.absentInputAudioBehavior = defaults.absentInputAudioBehavior;
this.arib = defaults.arib;
this.aribCaptionsPid = defaults.aribCaptionsPid;
this.aribCaptionsPidControl = defaults.aribCaptionsPidControl;
this.audioBufferModel = defaults.audioBufferModel;
this.audioFramesPerPes = defaults.audioFramesPerPes;
this.audioPids = defaults.audioPids;
this.audioStreamType = defaults.audioStreamType;
this.bitrate = defaults.bitrate;
this.bufferModel = defaults.bufferModel;
this.ccDescriptor = defaults.ccDescriptor;
this.dvbNitSettings = defaults.dvbNitSettings;
this.dvbSdtSettings = defaults.dvbSdtSettings;
this.dvbSubPids = defaults.dvbSubPids;
this.dvbTdtSettings = defaults.dvbTdtSettings;
this.dvbTeletextPid = defaults.dvbTeletextPid;
this.ebif = defaults.ebif;
this.ebpAudioInterval = defaults.ebpAudioInterval;
this.ebpLookaheadMs = defaults.ebpLookaheadMs;
this.ebpPlacement = defaults.ebpPlacement;
this.ecmPid = defaults.ecmPid;
this.esRateInPes = defaults.esRateInPes;
this.etvPlatformPid = defaults.etvPlatformPid;
this.etvSignalPid = defaults.etvSignalPid;
this.fragmentTime = defaults.fragmentTime;
this.klv = defaults.klv;
this.klvDataPids = defaults.klvDataPids;
this.nielsenId3Behavior = defaults.nielsenId3Behavior;
this.nullPacketBitrate = defaults.nullPacketBitrate;
this.patInterval = defaults.patInterval;
this.pcrControl = defaults.pcrControl;
this.pcrPeriod = defaults.pcrPeriod;
this.pcrPid = defaults.pcrPid;
this.pmtInterval = defaults.pmtInterval;
this.pmtPid = defaults.pmtPid;
this.programNum = defaults.programNum;
this.rateMode = defaults.rateMode;
this.scte27Pids = defaults.scte27Pids;
this.scte35Control = defaults.scte35Control;
this.scte35Pid = defaults.scte35Pid;
this.segmentationMarkers = defaults.segmentationMarkers;
this.segmentationStyle = defaults.segmentationStyle;
this.segmentationTime = defaults.segmentationTime;
this.timedMetadataBehavior = defaults.timedMetadataBehavior;
this.timedMetadataPid = defaults.timedMetadataPid;
this.transportStreamId = defaults.transportStreamId;
this.videoPid = defaults.videoPid;
}
@CustomType.Setter
public Builder absentInputAudioBehavior(@Nullable String absentInputAudioBehavior) {
this.absentInputAudioBehavior = absentInputAudioBehavior;
return this;
}
@CustomType.Setter
public Builder arib(@Nullable String arib) {
this.arib = arib;
return this;
}
@CustomType.Setter
public Builder aribCaptionsPid(@Nullable String aribCaptionsPid) {
this.aribCaptionsPid = aribCaptionsPid;
return this;
}
@CustomType.Setter
public Builder aribCaptionsPidControl(@Nullable String aribCaptionsPidControl) {
this.aribCaptionsPidControl = aribCaptionsPidControl;
return this;
}
@CustomType.Setter
public Builder audioBufferModel(@Nullable String audioBufferModel) {
this.audioBufferModel = audioBufferModel;
return this;
}
@CustomType.Setter
public Builder audioFramesPerPes(@Nullable Integer audioFramesPerPes) {
this.audioFramesPerPes = audioFramesPerPes;
return this;
}
@CustomType.Setter
public Builder audioPids(@Nullable String audioPids) {
this.audioPids = audioPids;
return this;
}
@CustomType.Setter
public Builder audioStreamType(@Nullable String audioStreamType) {
this.audioStreamType = audioStreamType;
return this;
}
@CustomType.Setter
public Builder bitrate(@Nullable Integer bitrate) {
this.bitrate = bitrate;
return this;
}
@CustomType.Setter
public Builder bufferModel(@Nullable String bufferModel) {
this.bufferModel = bufferModel;
return this;
}
@CustomType.Setter
public Builder ccDescriptor(@Nullable String ccDescriptor) {
this.ccDescriptor = ccDescriptor;
return this;
}
@CustomType.Setter
public Builder dvbNitSettings(@Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbNitSettings dvbNitSettings) {
this.dvbNitSettings = dvbNitSettings;
return this;
}
@CustomType.Setter
public Builder dvbSdtSettings(@Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbSdtSettings dvbSdtSettings) {
this.dvbSdtSettings = dvbSdtSettings;
return this;
}
@CustomType.Setter
public Builder dvbSubPids(@Nullable String dvbSubPids) {
this.dvbSubPids = dvbSubPids;
return this;
}
@CustomType.Setter
public Builder dvbTdtSettings(@Nullable ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbTdtSettings dvbTdtSettings) {
this.dvbTdtSettings = dvbTdtSettings;
return this;
}
@CustomType.Setter
public Builder dvbTeletextPid(@Nullable String dvbTeletextPid) {
this.dvbTeletextPid = dvbTeletextPid;
return this;
}
@CustomType.Setter
public Builder ebif(@Nullable String ebif) {
this.ebif = ebif;
return this;
}
@CustomType.Setter
public Builder ebpAudioInterval(@Nullable String ebpAudioInterval) {
this.ebpAudioInterval = ebpAudioInterval;
return this;
}
@CustomType.Setter
public Builder ebpLookaheadMs(@Nullable Integer ebpLookaheadMs) {
this.ebpLookaheadMs = ebpLookaheadMs;
return this;
}
@CustomType.Setter
public Builder ebpPlacement(@Nullable String ebpPlacement) {
this.ebpPlacement = ebpPlacement;
return this;
}
@CustomType.Setter
public Builder ecmPid(@Nullable String ecmPid) {
this.ecmPid = ecmPid;
return this;
}
@CustomType.Setter
public Builder esRateInPes(@Nullable String esRateInPes) {
this.esRateInPes = esRateInPes;
return this;
}
@CustomType.Setter
public Builder etvPlatformPid(@Nullable String etvPlatformPid) {
this.etvPlatformPid = etvPlatformPid;
return this;
}
@CustomType.Setter
public Builder etvSignalPid(@Nullable String etvSignalPid) {
this.etvSignalPid = etvSignalPid;
return this;
}
@CustomType.Setter
public Builder fragmentTime(@Nullable Double fragmentTime) {
this.fragmentTime = fragmentTime;
return this;
}
@CustomType.Setter
public Builder klv(@Nullable String klv) {
this.klv = klv;
return this;
}
@CustomType.Setter
public Builder klvDataPids(@Nullable String klvDataPids) {
this.klvDataPids = klvDataPids;
return this;
}
@CustomType.Setter
public Builder nielsenId3Behavior(@Nullable String nielsenId3Behavior) {
this.nielsenId3Behavior = nielsenId3Behavior;
return this;
}
@CustomType.Setter
public Builder nullPacketBitrate(@Nullable Double nullPacketBitrate) {
this.nullPacketBitrate = nullPacketBitrate;
return this;
}
@CustomType.Setter
public Builder patInterval(@Nullable Integer patInterval) {
this.patInterval = patInterval;
return this;
}
@CustomType.Setter
public Builder pcrControl(@Nullable String pcrControl) {
this.pcrControl = pcrControl;
return this;
}
@CustomType.Setter
public Builder pcrPeriod(@Nullable Integer pcrPeriod) {
this.pcrPeriod = pcrPeriod;
return this;
}
@CustomType.Setter
public Builder pcrPid(@Nullable String pcrPid) {
this.pcrPid = pcrPid;
return this;
}
@CustomType.Setter
public Builder pmtInterval(@Nullable Integer pmtInterval) {
this.pmtInterval = pmtInterval;
return this;
}
@CustomType.Setter
public Builder pmtPid(@Nullable String pmtPid) {
this.pmtPid = pmtPid;
return this;
}
@CustomType.Setter
public Builder programNum(@Nullable Integer programNum) {
this.programNum = programNum;
return this;
}
@CustomType.Setter
public Builder rateMode(@Nullable String rateMode) {
this.rateMode = rateMode;
return this;
}
@CustomType.Setter
public Builder scte27Pids(@Nullable String scte27Pids) {
this.scte27Pids = scte27Pids;
return this;
}
@CustomType.Setter
public Builder scte35Control(@Nullable String scte35Control) {
this.scte35Control = scte35Control;
return this;
}
@CustomType.Setter
public Builder scte35Pid(@Nullable String scte35Pid) {
this.scte35Pid = scte35Pid;
return this;
}
@CustomType.Setter
public Builder segmentationMarkers(@Nullable String segmentationMarkers) {
this.segmentationMarkers = segmentationMarkers;
return this;
}
@CustomType.Setter
public Builder segmentationStyle(@Nullable String segmentationStyle) {
this.segmentationStyle = segmentationStyle;
return this;
}
@CustomType.Setter
public Builder segmentationTime(@Nullable Double segmentationTime) {
this.segmentationTime = segmentationTime;
return this;
}
@CustomType.Setter
public Builder timedMetadataBehavior(@Nullable String timedMetadataBehavior) {
this.timedMetadataBehavior = timedMetadataBehavior;
return this;
}
@CustomType.Setter
public Builder timedMetadataPid(@Nullable String timedMetadataPid) {
this.timedMetadataPid = timedMetadataPid;
return this;
}
@CustomType.Setter
public Builder transportStreamId(@Nullable Integer transportStreamId) {
this.transportStreamId = transportStreamId;
return this;
}
@CustomType.Setter
public Builder videoPid(@Nullable String videoPid) {
this.videoPid = videoPid;
return this;
}
public ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings build() {
final var _resultValue = new ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings();
_resultValue.absentInputAudioBehavior = absentInputAudioBehavior;
_resultValue.arib = arib;
_resultValue.aribCaptionsPid = aribCaptionsPid;
_resultValue.aribCaptionsPidControl = aribCaptionsPidControl;
_resultValue.audioBufferModel = audioBufferModel;
_resultValue.audioFramesPerPes = audioFramesPerPes;
_resultValue.audioPids = audioPids;
_resultValue.audioStreamType = audioStreamType;
_resultValue.bitrate = bitrate;
_resultValue.bufferModel = bufferModel;
_resultValue.ccDescriptor = ccDescriptor;
_resultValue.dvbNitSettings = dvbNitSettings;
_resultValue.dvbSdtSettings = dvbSdtSettings;
_resultValue.dvbSubPids = dvbSubPids;
_resultValue.dvbTdtSettings = dvbTdtSettings;
_resultValue.dvbTeletextPid = dvbTeletextPid;
_resultValue.ebif = ebif;
_resultValue.ebpAudioInterval = ebpAudioInterval;
_resultValue.ebpLookaheadMs = ebpLookaheadMs;
_resultValue.ebpPlacement = ebpPlacement;
_resultValue.ecmPid = ecmPid;
_resultValue.esRateInPes = esRateInPes;
_resultValue.etvPlatformPid = etvPlatformPid;
_resultValue.etvSignalPid = etvSignalPid;
_resultValue.fragmentTime = fragmentTime;
_resultValue.klv = klv;
_resultValue.klvDataPids = klvDataPids;
_resultValue.nielsenId3Behavior = nielsenId3Behavior;
_resultValue.nullPacketBitrate = nullPacketBitrate;
_resultValue.patInterval = patInterval;
_resultValue.pcrControl = pcrControl;
_resultValue.pcrPeriod = pcrPeriod;
_resultValue.pcrPid = pcrPid;
_resultValue.pmtInterval = pmtInterval;
_resultValue.pmtPid = pmtPid;
_resultValue.programNum = programNum;
_resultValue.rateMode = rateMode;
_resultValue.scte27Pids = scte27Pids;
_resultValue.scte35Control = scte35Control;
_resultValue.scte35Pid = scte35Pid;
_resultValue.segmentationMarkers = segmentationMarkers;
_resultValue.segmentationStyle = segmentationStyle;
_resultValue.segmentationTime = segmentationTime;
_resultValue.timedMetadataBehavior = timedMetadataBehavior;
_resultValue.timedMetadataPid = timedMetadataPid;
_resultValue.transportStreamId = transportStreamId;
_resultValue.videoPid = videoPid;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy