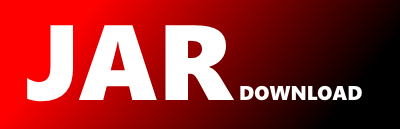
com.pulumi.aws.msk.ScramSecretAssociation Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.msk;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.msk.ScramSecretAssociationArgs;
import com.pulumi.aws.msk.inputs.ScramSecretAssociationState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* Associates SCRAM secrets stored in the Secrets Manager service with a Managed Streaming for Kafka (MSK) cluster.
*
* > **Note:** The following assumes the MSK cluster has SASL/SCRAM authentication enabled. See below for example usage or refer to the [Username/Password Authentication](https://docs.aws.amazon.com/msk/latest/developerguide/msk-password.html) section of the MSK Developer Guide for more details.
*
* To set up username and password authentication for a cluster, create an `aws.secretsmanager.Secret` resource and associate
* a username and password with the secret with an `aws.secretsmanager.SecretVersion` resource. When creating a secret for the cluster,
* the `name` must have the prefix `AmazonMSK_` and you must either use an existing custom AWS KMS key or create a new
* custom AWS KMS key for your secret with the `aws.kms.Key` resource. It is important to note that a policy is required for the `aws.secretsmanager.Secret`
* resource in order for Kafka to be able to read it. This policy is attached automatically when the `aws.msk.ScramSecretAssociation` is used,
* however, this policy will not be in the state and as such, will present a diff on plan/apply. For that reason, you must use the `aws.secretsmanager.SecretPolicy`
* resource](/docs/providers/aws/r/secretsmanager_secret_policy.html) as shown below in order to ensure that the state is in a clean state after the creation of secret and the association to the cluster.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.msk.Cluster;
* import com.pulumi.aws.msk.ClusterArgs;
* import com.pulumi.aws.msk.inputs.ClusterClientAuthenticationArgs;
* import com.pulumi.aws.msk.inputs.ClusterClientAuthenticationSaslArgs;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.secretsmanager.Secret;
* import com.pulumi.aws.secretsmanager.SecretArgs;
* import com.pulumi.aws.secretsmanager.SecretVersion;
* import com.pulumi.aws.secretsmanager.SecretVersionArgs;
* import com.pulumi.aws.msk.ScramSecretAssociation;
* import com.pulumi.aws.msk.ScramSecretAssociationArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.secretsmanager.SecretPolicy;
* import com.pulumi.aws.secretsmanager.SecretPolicyArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .clusterName("example")
* .clientAuthentication(ClusterClientAuthenticationArgs.builder()
* .sasl(ClusterClientAuthenticationSaslArgs.builder()
* .scram(true)
* .build())
* .build())
* .build());
*
* var exampleKey = new Key("exampleKey", KeyArgs.builder()
* .description("Example Key for MSK Cluster Scram Secret Association")
* .build());
*
* var exampleSecret = new Secret("exampleSecret", SecretArgs.builder()
* .name("AmazonMSK_example")
* .kmsKeyId(exampleKey.keyId())
* .build());
*
* var exampleSecretVersion = new SecretVersion("exampleSecretVersion", SecretVersionArgs.builder()
* .secretId(exampleSecret.id())
* .secretString(serializeJson(
* jsonObject(
* jsonProperty("username", "user"),
* jsonProperty("password", "pass")
* )))
* .build());
*
* var exampleScramSecretAssociation = new ScramSecretAssociation("exampleScramSecretAssociation", ScramSecretAssociationArgs.builder()
* .clusterArn(exampleCluster.arn())
* .secretArnLists(exampleSecret.arn())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleSecretVersion)
* .build());
*
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .sid("AWSKafkaResourcePolicy")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("kafka.amazonaws.com")
* .build())
* .actions("secretsmanager:getSecretValue")
* .resources(exampleSecret.arn())
* .build())
* .build());
*
* var exampleSecretPolicy = new SecretPolicy("exampleSecretPolicy", SecretPolicyArgs.builder()
* .secretArn(exampleSecret.arn())
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(example -> example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import MSK SCRAM Secret Associations using the `id`. For example:
*
* ```sh
* $ pulumi import aws:msk/scramSecretAssociation:ScramSecretAssociation example arn:aws:kafka:us-west-2:123456789012:cluster/example/279c0212-d057-4dba-9aa9-1c4e5a25bfc7-3
* ```
*
*/
@ResourceType(type="aws:msk/scramSecretAssociation:ScramSecretAssociation")
public class ScramSecretAssociation extends com.pulumi.resources.CustomResource {
/**
* Amazon Resource Name (ARN) of the MSK cluster.
*
*/
@Export(name="clusterArn", refs={String.class}, tree="[0]")
private Output clusterArn;
/**
* @return Amazon Resource Name (ARN) of the MSK cluster.
*
*/
public Output clusterArn() {
return this.clusterArn;
}
/**
* List of AWS Secrets Manager secret ARNs.
*
*/
@Export(name="secretArnLists", refs={List.class,String.class}, tree="[0,1]")
private Output> secretArnLists;
/**
* @return List of AWS Secrets Manager secret ARNs.
*
*/
public Output> secretArnLists() {
return this.secretArnLists;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ScramSecretAssociation(java.lang.String name) {
this(name, ScramSecretAssociationArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ScramSecretAssociation(java.lang.String name, ScramSecretAssociationArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ScramSecretAssociation(java.lang.String name, ScramSecretAssociationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:msk/scramSecretAssociation:ScramSecretAssociation", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ScramSecretAssociation(java.lang.String name, Output id, @Nullable ScramSecretAssociationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:msk/scramSecretAssociation:ScramSecretAssociation", name, state, makeResourceOptions(options, id), false);
}
private static ScramSecretAssociationArgs makeArgs(ScramSecretAssociationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ScramSecretAssociationArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ScramSecretAssociation get(java.lang.String name, Output id, @Nullable ScramSecretAssociationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ScramSecretAssociation(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy