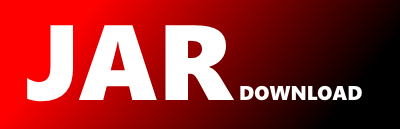
com.pulumi.aws.neptune.ClusterInstance Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.neptune;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.neptune.ClusterInstanceArgs;
import com.pulumi.aws.neptune.inputs.ClusterInstanceState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A Cluster Instance Resource defines attributes that are specific to a single instance in a Neptune Cluster.
*
* You can simply add neptune instances and Neptune manages the replication. You can use the count
* meta-parameter to make multiple instances and join them all to the same Neptune Cluster, or you may specify different Cluster Instance resources with various `instance_class` sizes.
*
* ## Example Usage
*
* The following example will create a neptune cluster with two neptune instances(one writer and one reader).
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.neptune.Cluster;
* import com.pulumi.aws.neptune.ClusterArgs;
* import com.pulumi.aws.neptune.ClusterInstance;
* import com.pulumi.aws.neptune.ClusterInstanceArgs;
* import com.pulumi.codegen.internal.KeyedValue;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("neptune-cluster-demo")
* .engine("neptune")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .skipFinalSnapshot(true)
* .iamDatabaseAuthenticationEnabled(true)
* .applyImmediately(true)
* .build());
*
* for (var i = 0; i < 2; i++) {
* new ClusterInstance("example-" + i, ClusterInstanceArgs.builder()
* .clusterIdentifier(default_.id())
* .engine("neptune")
* .instanceClass("db.r4.large")
* .applyImmediately(true)
* .build());
*
*
* }
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_neptune_cluster_instance` using the instance identifier. For example:
*
* ```sh
* $ pulumi import aws:neptune/clusterInstance:ClusterInstance example my-instance
* ```
*
*/
@ResourceType(type="aws:neptune/clusterInstance:ClusterInstance")
public class ClusterInstance extends com.pulumi.resources.CustomResource {
/**
* The hostname of the instance. See also `endpoint` and `port`.
*
*/
@Export(name="address", refs={String.class}, tree="[0]")
private Output address;
/**
* @return The hostname of the instance. See also `endpoint` and `port`.
*
*/
public Output address() {
return this.address;
}
/**
* Specifies whether any instance modifications
* are applied immediately, or during the next maintenance window. Default is`false`.
*
*/
@Export(name="applyImmediately", refs={Boolean.class}, tree="[0]")
private Output applyImmediately;
/**
* @return Specifies whether any instance modifications
* are applied immediately, or during the next maintenance window. Default is`false`.
*
*/
public Output applyImmediately() {
return this.applyImmediately;
}
/**
* Amazon Resource Name (ARN) of neptune instance
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) of neptune instance
*
*/
public Output arn() {
return this.arn;
}
/**
* Indicates that minor engine upgrades will be applied automatically to the instance during the maintenance window. Default is `true`.
*
*/
@Export(name="autoMinorVersionUpgrade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoMinorVersionUpgrade;
/**
* @return Indicates that minor engine upgrades will be applied automatically to the instance during the maintenance window. Default is `true`.
*
*/
public Output> autoMinorVersionUpgrade() {
return Codegen.optional(this.autoMinorVersionUpgrade);
}
/**
* The EC2 Availability Zone that the neptune instance is created in.
*
*/
@Export(name="availabilityZone", refs={String.class}, tree="[0]")
private Output availabilityZone;
/**
* @return The EC2 Availability Zone that the neptune instance is created in.
*
*/
public Output availabilityZone() {
return this.availabilityZone;
}
/**
* The identifier of the `aws.neptune.Cluster` in which to launch this instance.
*
*/
@Export(name="clusterIdentifier", refs={String.class}, tree="[0]")
private Output clusterIdentifier;
/**
* @return The identifier of the `aws.neptune.Cluster` in which to launch this instance.
*
*/
public Output clusterIdentifier() {
return this.clusterIdentifier;
}
/**
* The region-unique, immutable identifier for the neptune instance.
*
*/
@Export(name="dbiResourceId", refs={String.class}, tree="[0]")
private Output dbiResourceId;
/**
* @return The region-unique, immutable identifier for the neptune instance.
*
*/
public Output dbiResourceId() {
return this.dbiResourceId;
}
/**
* The connection endpoint in `address:port` format.
*
*/
@Export(name="endpoint", refs={String.class}, tree="[0]")
private Output endpoint;
/**
* @return The connection endpoint in `address:port` format.
*
*/
public Output endpoint() {
return this.endpoint;
}
/**
* The name of the database engine to be used for the neptune instance. Defaults to `neptune`. Valid Values: `neptune`.
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> engine;
/**
* @return The name of the database engine to be used for the neptune instance. Defaults to `neptune`. Valid Values: `neptune`.
*
*/
public Output> engine() {
return Codegen.optional(this.engine);
}
/**
* The neptune engine version. Currently configuring this argumnet has no effect.
*
*/
@Export(name="engineVersion", refs={String.class}, tree="[0]")
private Output engineVersion;
/**
* @return The neptune engine version. Currently configuring this argumnet has no effect.
*
*/
public Output engineVersion() {
return this.engineVersion;
}
/**
* The identifier for the neptune instance, if omitted, this provider will assign a random, unique identifier.
*
*/
@Export(name="identifier", refs={String.class}, tree="[0]")
private Output identifier;
/**
* @return The identifier for the neptune instance, if omitted, this provider will assign a random, unique identifier.
*
*/
public Output identifier() {
return this.identifier;
}
/**
* Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*
*/
@Export(name="identifierPrefix", refs={String.class}, tree="[0]")
private Output identifierPrefix;
/**
* @return Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*
*/
public Output identifierPrefix() {
return this.identifierPrefix;
}
/**
* The instance class to use.
*
*/
@Export(name="instanceClass", refs={String.class}, tree="[0]")
private Output instanceClass;
/**
* @return The instance class to use.
*
*/
public Output instanceClass() {
return this.instanceClass;
}
/**
* The ARN for the KMS encryption key if one is set to the neptune cluster.
*
*/
@Export(name="kmsKeyArn", refs={String.class}, tree="[0]")
private Output kmsKeyArn;
/**
* @return The ARN for the KMS encryption key if one is set to the neptune cluster.
*
*/
public Output kmsKeyArn() {
return this.kmsKeyArn;
}
/**
* The name of the neptune parameter group to associate with this instance.
*
*/
@Export(name="neptuneParameterGroupName", refs={String.class}, tree="[0]")
private Output neptuneParameterGroupName;
/**
* @return The name of the neptune parameter group to associate with this instance.
*
*/
public Output neptuneParameterGroupName() {
return this.neptuneParameterGroupName;
}
/**
* A subnet group to associate with this neptune instance. **NOTE:** This must match the `neptune_subnet_group_name` of the attached `aws.neptune.Cluster`.
*
*/
@Export(name="neptuneSubnetGroupName", refs={String.class}, tree="[0]")
private Output neptuneSubnetGroupName;
/**
* @return A subnet group to associate with this neptune instance. **NOTE:** This must match the `neptune_subnet_group_name` of the attached `aws.neptune.Cluster`.
*
*/
public Output neptuneSubnetGroupName() {
return this.neptuneSubnetGroupName;
}
/**
* The port on which the DB accepts connections. Defaults to `8182`.
*
*/
@Export(name="port", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> port;
/**
* @return The port on which the DB accepts connections. Defaults to `8182`.
*
*/
public Output> port() {
return Codegen.optional(this.port);
}
/**
* The daily time range during which automated backups are created if automated backups are enabled. Eg: "04:00-09:00"
*
*/
@Export(name="preferredBackupWindow", refs={String.class}, tree="[0]")
private Output preferredBackupWindow;
/**
* @return The daily time range during which automated backups are created if automated backups are enabled. Eg: "04:00-09:00"
*
*/
public Output preferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
* The window to perform maintenance in.
* Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00".
*
*/
@Export(name="preferredMaintenanceWindow", refs={String.class}, tree="[0]")
private Output preferredMaintenanceWindow;
/**
* @return The window to perform maintenance in.
* Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00".
*
*/
public Output preferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
* Default 0. Failover Priority setting on instance level. The reader who has lower tier has higher priority to get promoter to writer.
*
*/
@Export(name="promotionTier", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> promotionTier;
/**
* @return Default 0. Failover Priority setting on instance level. The reader who has lower tier has higher priority to get promoter to writer.
*
*/
public Output> promotionTier() {
return Codegen.optional(this.promotionTier);
}
/**
* Bool to control if instance is publicly accessible. Default is `false`.
*
*/
@Export(name="publiclyAccessible", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> publiclyAccessible;
/**
* @return Bool to control if instance is publicly accessible. Default is `false`.
*
*/
public Output> publiclyAccessible() {
return Codegen.optional(this.publiclyAccessible);
}
/**
* Determines whether a final DB snapshot is created before the DB instance is deleted.
*
*/
@Export(name="skipFinalSnapshot", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipFinalSnapshot;
/**
* @return Determines whether a final DB snapshot is created before the DB instance is deleted.
*
*/
public Output> skipFinalSnapshot() {
return Codegen.optional(this.skipFinalSnapshot);
}
/**
* Specifies whether the neptune cluster is encrypted.
*
*/
@Export(name="storageEncrypted", refs={Boolean.class}, tree="[0]")
private Output storageEncrypted;
/**
* @return Specifies whether the neptune cluster is encrypted.
*
*/
public Output storageEncrypted() {
return this.storageEncrypted;
}
/**
* Storage type associated with the cluster `standard/iopt1`.
*
*/
@Export(name="storageType", refs={String.class}, tree="[0]")
private Output storageType;
/**
* @return Storage type associated with the cluster `standard/iopt1`.
*
*/
public Output storageType() {
return this.storageType;
}
/**
* A map of tags to assign to the instance. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the instance. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy