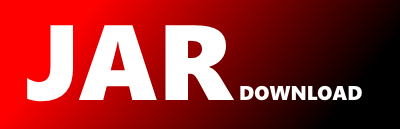
com.pulumi.aws.opensearch.OutboundConnectionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.opensearch;
import com.pulumi.aws.opensearch.inputs.OutboundConnectionConnectionPropertiesArgs;
import com.pulumi.aws.opensearch.inputs.OutboundConnectionLocalDomainInfoArgs;
import com.pulumi.aws.opensearch.inputs.OutboundConnectionRemoteDomainInfoArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OutboundConnectionArgs extends com.pulumi.resources.ResourceArgs {
public static final OutboundConnectionArgs Empty = new OutboundConnectionArgs();
/**
* Accepts the connection.
*
*/
@Import(name="acceptConnection")
private @Nullable Output acceptConnection;
/**
* @return Accepts the connection.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy