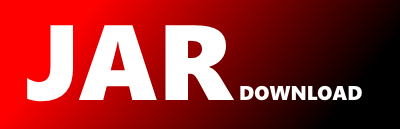
com.pulumi.aws.paymentcryptography.inputs.KeyState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.paymentcryptography.inputs;
import com.pulumi.aws.paymentcryptography.inputs.KeyKeyAttributesArgs;
import com.pulumi.aws.paymentcryptography.inputs.KeyTimeoutsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KeyState extends com.pulumi.resources.ResourceArgs {
public static final KeyState Empty = new KeyState();
/**
* ARN of the key.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return ARN of the key.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy