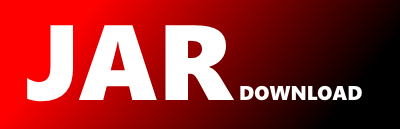
com.pulumi.aws.quicksight.Analysis Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.quicksight;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.quicksight.AnalysisArgs;
import com.pulumi.aws.quicksight.inputs.AnalysisState;
import com.pulumi.aws.quicksight.outputs.AnalysisParameters;
import com.pulumi.aws.quicksight.outputs.AnalysisPermission;
import com.pulumi.aws.quicksight.outputs.AnalysisSourceEntity;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing a QuickSight Analysis.
*
* ## Example Usage
*
* ### From Source Template
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.quicksight.Analysis;
* import com.pulumi.aws.quicksight.AnalysisArgs;
* import com.pulumi.aws.quicksight.inputs.AnalysisSourceEntityArgs;
* import com.pulumi.aws.quicksight.inputs.AnalysisSourceEntitySourceTemplateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Analysis("example", AnalysisArgs.builder()
* .analysisId("example-id")
* .name("example-name")
* .sourceEntity(AnalysisSourceEntityArgs.builder()
* .sourceTemplate(AnalysisSourceEntitySourceTemplateArgs.builder()
* .arn(source.arn())
* .dataSetReferences(AnalysisSourceEntitySourceTemplateDataSetReferenceArgs.builder()
* .dataSetArn(dataset.arn())
* .dataSetPlaceholder("1")
* .build())
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With Definition
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import a QuickSight Analysis using the AWS account ID and analysis ID separated by a comma (`,`). For example:
*
* ```sh
* $ pulumi import aws:quicksight/analysis:Analysis example 123456789012,example-id
* ```
*
*/
@ResourceType(type="aws:quicksight/analysis:Analysis")
public class Analysis extends com.pulumi.resources.CustomResource {
/**
* Identifier for the analysis.
*
*/
@Export(name="analysisId", refs={String.class}, tree="[0]")
private Output analysisId;
/**
* @return Identifier for the analysis.
*
*/
public Output analysisId() {
return this.analysisId;
}
/**
* ARN of the analysis.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the analysis.
*
*/
public Output arn() {
return this.arn;
}
/**
* AWS account ID.
*
*/
@Export(name="awsAccountId", refs={String.class}, tree="[0]")
private Output awsAccountId;
/**
* @return AWS account ID.
*
*/
public Output awsAccountId() {
return this.awsAccountId;
}
/**
* The time that the analysis was created.
*
*/
@Export(name="createdTime", refs={String.class}, tree="[0]")
private Output createdTime;
/**
* @return The time that the analysis was created.
*
*/
public Output createdTime() {
return this.createdTime;
}
@Export(name="lastPublishedTime", refs={String.class}, tree="[0]")
private Output lastPublishedTime;
public Output lastPublishedTime() {
return this.lastPublishedTime;
}
/**
* The time that the analysis was last updated.
*
*/
@Export(name="lastUpdatedTime", refs={String.class}, tree="[0]")
private Output lastUpdatedTime;
/**
* @return The time that the analysis was last updated.
*
*/
public Output lastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
* Display name for the analysis.
*
* The following arguments are optional:
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Display name for the analysis.
*
* The following arguments are optional:
*
*/
public Output name() {
return this.name;
}
/**
* The parameters for the creation of the analysis, which you want to use to override the default settings. An analysis can have any type of parameters, and some parameters might accept multiple values. See parameters.
*
*/
@Export(name="parameters", refs={AnalysisParameters.class}, tree="[0]")
private Output parameters;
/**
* @return The parameters for the creation of the analysis, which you want to use to override the default settings. An analysis can have any type of parameters, and some parameters might accept multiple values. See parameters.
*
*/
public Output parameters() {
return this.parameters;
}
/**
* A set of resource permissions on the analysis. Maximum of 64 items. See permissions.
*
*/
@Export(name="permissions", refs={List.class,AnalysisPermission.class}, tree="[0,1]")
private Output* @Nullable */ List> permissions;
/**
* @return A set of resource permissions on the analysis. Maximum of 64 items. See permissions.
*
*/
public Output>> permissions() {
return Codegen.optional(this.permissions);
}
/**
* A value that specifies the number of days that Amazon QuickSight waits before it deletes the analysis. Use `0` to force deletion without recovery. Minimum value of `7`. Maximum value of `30`. Default to `30`.
*
*/
@Export(name="recoveryWindowInDays", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> recoveryWindowInDays;
/**
* @return A value that specifies the number of days that Amazon QuickSight waits before it deletes the analysis. Use `0` to force deletion without recovery. Minimum value of `7`. Maximum value of `30`. Default to `30`.
*
*/
public Output> recoveryWindowInDays() {
return Codegen.optional(this.recoveryWindowInDays);
}
/**
* The entity that you are using as a source when you create the analysis (template). Only one of `definition` or `source_entity` should be configured. See source_entity.
*
*/
@Export(name="sourceEntity", refs={AnalysisSourceEntity.class}, tree="[0]")
private Output* @Nullable */ AnalysisSourceEntity> sourceEntity;
/**
* @return The entity that you are using as a source when you create the analysis (template). Only one of `definition` or `source_entity` should be configured. See source_entity.
*
*/
public Output> sourceEntity() {
return Codegen.optional(this.sourceEntity);
}
/**
* The analysis creation status.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The analysis creation status.
*
*/
public Output status() {
return this.status;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy