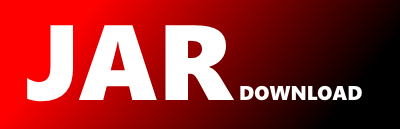
com.pulumi.aws.quicksight.Theme Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.quicksight;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.quicksight.ThemeArgs;
import com.pulumi.aws.quicksight.inputs.ThemeState;
import com.pulumi.aws.quicksight.outputs.ThemeConfiguration;
import com.pulumi.aws.quicksight.outputs.ThemePermission;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing a QuickSight Theme.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.quicksight.Theme;
* import com.pulumi.aws.quicksight.ThemeArgs;
* import com.pulumi.aws.quicksight.inputs.ThemeConfigurationArgs;
* import com.pulumi.aws.quicksight.inputs.ThemeConfigurationDataColorPaletteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Theme("example", ThemeArgs.builder()
* .themeId("example")
* .name("example")
* .baseThemeId("MIDNIGHT")
* .configuration(ThemeConfigurationArgs.builder()
* .dataColorPalette(ThemeConfigurationDataColorPaletteArgs.builder()
* .colors(
* "#FFFFFF",
* "#111111",
* "#222222",
* "#333333",
* "#444444",
* "#555555",
* "#666666",
* "#777777",
* "#888888",
* "#999999")
* .emptyFillColor("#FFFFFF")
* .minMaxGradients(
* "#FFFFFF",
* "#111111")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import a QuickSight Theme using the AWS account ID and theme ID separated by a comma (`,`). For example:
*
* ```sh
* $ pulumi import aws:quicksight/theme:Theme example 123456789012,example-id
* ```
*
*/
@ResourceType(type="aws:quicksight/theme:Theme")
public class Theme extends com.pulumi.resources.CustomResource {
/**
* ARN of the theme.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the theme.
*
*/
public Output arn() {
return this.arn;
}
/**
* AWS account ID.
*
*/
@Export(name="awsAccountId", refs={String.class}, tree="[0]")
private Output awsAccountId;
/**
* @return AWS account ID.
*
*/
public Output awsAccountId() {
return this.awsAccountId;
}
/**
* The ID of the theme that a custom theme will inherit from. All themes inherit from one of the starting themes defined by Amazon QuickSight. For a list of the starting themes, use ListThemes or choose Themes from within an analysis.
*
*/
@Export(name="baseThemeId", refs={String.class}, tree="[0]")
private Output baseThemeId;
/**
* @return The ID of the theme that a custom theme will inherit from. All themes inherit from one of the starting themes defined by Amazon QuickSight. For a list of the starting themes, use ListThemes or choose Themes from within an analysis.
*
*/
public Output baseThemeId() {
return this.baseThemeId;
}
/**
* The theme configuration, which contains the theme display properties. See configuration.
*
* The following arguments are optional:
*
*/
@Export(name="configuration", refs={ThemeConfiguration.class}, tree="[0]")
private Output* @Nullable */ ThemeConfiguration> configuration;
/**
* @return The theme configuration, which contains the theme display properties. See configuration.
*
* The following arguments are optional:
*
*/
public Output> configuration() {
return Codegen.optional(this.configuration);
}
/**
* The time that the theme was created.
*
*/
@Export(name="createdTime", refs={String.class}, tree="[0]")
private Output createdTime;
/**
* @return The time that the theme was created.
*
*/
public Output createdTime() {
return this.createdTime;
}
/**
* The time that the theme was last updated.
*
*/
@Export(name="lastUpdatedTime", refs={String.class}, tree="[0]")
private Output lastUpdatedTime;
/**
* @return The time that the theme was last updated.
*
*/
public Output lastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
* Display name of the theme.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Display name of the theme.
*
*/
public Output name() {
return this.name;
}
/**
* A set of resource permissions on the theme. Maximum of 64 items. See permissions.
*
*/
@Export(name="permissions", refs={List.class,ThemePermission.class}, tree="[0,1]")
private Output* @Nullable */ List> permissions;
/**
* @return A set of resource permissions on the theme. Maximum of 64 items. See permissions.
*
*/
public Output>> permissions() {
return Codegen.optional(this.permissions);
}
/**
* The theme creation status.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The theme creation status.
*
*/
public Output status() {
return this.status;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy