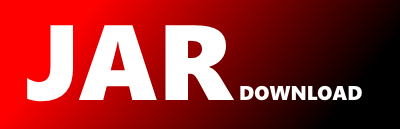
com.pulumi.aws.rds.outputs.GetClusterSnapshotResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetClusterSnapshotResult {
/**
* @return Allocated storage size in gigabytes (GB).
*
*/
private Integer allocatedStorage;
/**
* @return List of EC2 Availability Zones that instances in the DB cluster snapshot can be restored in.
*
*/
private List availabilityZones;
/**
* @return Specifies the DB cluster identifier of the DB cluster that this DB cluster snapshot was created from.
*
*/
private @Nullable String dbClusterIdentifier;
/**
* @return The ARN for the DB Cluster Snapshot.
*
*/
private String dbClusterSnapshotArn;
private @Nullable String dbClusterSnapshotIdentifier;
/**
* @return Name of the database engine.
*
*/
private String engine;
/**
* @return Version of the database engine for this DB cluster snapshot.
*
*/
private String engineVersion;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private @Nullable Boolean includePublic;
private @Nullable Boolean includeShared;
/**
* @return If storage_encrypted is true, the AWS KMS key identifier for the encrypted DB cluster snapshot.
*
*/
private String kmsKeyId;
/**
* @return License model information for the restored DB cluster.
*
*/
private String licenseModel;
private @Nullable Boolean mostRecent;
/**
* @return Port that the DB cluster was listening on at the time of the snapshot.
*
*/
private Integer port;
/**
* @return Time when the snapshot was taken, in Universal Coordinated Time (UTC).
*
*/
private String snapshotCreateTime;
private @Nullable String snapshotType;
private String sourceDbClusterSnapshotArn;
/**
* @return Status of this DB Cluster Snapshot.
*
*/
private String status;
/**
* @return Whether the DB cluster snapshot is encrypted.
*
*/
private Boolean storageEncrypted;
/**
* @return Map of tags for the resource.
*
*/
private Map tags;
/**
* @return VPC ID associated with the DB cluster snapshot.
*
*/
private String vpcId;
private GetClusterSnapshotResult() {}
/**
* @return Allocated storage size in gigabytes (GB).
*
*/
public Integer allocatedStorage() {
return this.allocatedStorage;
}
/**
* @return List of EC2 Availability Zones that instances in the DB cluster snapshot can be restored in.
*
*/
public List availabilityZones() {
return this.availabilityZones;
}
/**
* @return Specifies the DB cluster identifier of the DB cluster that this DB cluster snapshot was created from.
*
*/
public Optional dbClusterIdentifier() {
return Optional.ofNullable(this.dbClusterIdentifier);
}
/**
* @return The ARN for the DB Cluster Snapshot.
*
*/
public String dbClusterSnapshotArn() {
return this.dbClusterSnapshotArn;
}
public Optional dbClusterSnapshotIdentifier() {
return Optional.ofNullable(this.dbClusterSnapshotIdentifier);
}
/**
* @return Name of the database engine.
*
*/
public String engine() {
return this.engine;
}
/**
* @return Version of the database engine for this DB cluster snapshot.
*
*/
public String engineVersion() {
return this.engineVersion;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public Optional includePublic() {
return Optional.ofNullable(this.includePublic);
}
public Optional includeShared() {
return Optional.ofNullable(this.includeShared);
}
/**
* @return If storage_encrypted is true, the AWS KMS key identifier for the encrypted DB cluster snapshot.
*
*/
public String kmsKeyId() {
return this.kmsKeyId;
}
/**
* @return License model information for the restored DB cluster.
*
*/
public String licenseModel() {
return this.licenseModel;
}
public Optional mostRecent() {
return Optional.ofNullable(this.mostRecent);
}
/**
* @return Port that the DB cluster was listening on at the time of the snapshot.
*
*/
public Integer port() {
return this.port;
}
/**
* @return Time when the snapshot was taken, in Universal Coordinated Time (UTC).
*
*/
public String snapshotCreateTime() {
return this.snapshotCreateTime;
}
public Optional snapshotType() {
return Optional.ofNullable(this.snapshotType);
}
public String sourceDbClusterSnapshotArn() {
return this.sourceDbClusterSnapshotArn;
}
/**
* @return Status of this DB Cluster Snapshot.
*
*/
public String status() {
return this.status;
}
/**
* @return Whether the DB cluster snapshot is encrypted.
*
*/
public Boolean storageEncrypted() {
return this.storageEncrypted;
}
/**
* @return Map of tags for the resource.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return VPC ID associated with the DB cluster snapshot.
*
*/
public String vpcId() {
return this.vpcId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetClusterSnapshotResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer allocatedStorage;
private List availabilityZones;
private @Nullable String dbClusterIdentifier;
private String dbClusterSnapshotArn;
private @Nullable String dbClusterSnapshotIdentifier;
private String engine;
private String engineVersion;
private String id;
private @Nullable Boolean includePublic;
private @Nullable Boolean includeShared;
private String kmsKeyId;
private String licenseModel;
private @Nullable Boolean mostRecent;
private Integer port;
private String snapshotCreateTime;
private @Nullable String snapshotType;
private String sourceDbClusterSnapshotArn;
private String status;
private Boolean storageEncrypted;
private Map tags;
private String vpcId;
public Builder() {}
public Builder(GetClusterSnapshotResult defaults) {
Objects.requireNonNull(defaults);
this.allocatedStorage = defaults.allocatedStorage;
this.availabilityZones = defaults.availabilityZones;
this.dbClusterIdentifier = defaults.dbClusterIdentifier;
this.dbClusterSnapshotArn = defaults.dbClusterSnapshotArn;
this.dbClusterSnapshotIdentifier = defaults.dbClusterSnapshotIdentifier;
this.engine = defaults.engine;
this.engineVersion = defaults.engineVersion;
this.id = defaults.id;
this.includePublic = defaults.includePublic;
this.includeShared = defaults.includeShared;
this.kmsKeyId = defaults.kmsKeyId;
this.licenseModel = defaults.licenseModel;
this.mostRecent = defaults.mostRecent;
this.port = defaults.port;
this.snapshotCreateTime = defaults.snapshotCreateTime;
this.snapshotType = defaults.snapshotType;
this.sourceDbClusterSnapshotArn = defaults.sourceDbClusterSnapshotArn;
this.status = defaults.status;
this.storageEncrypted = defaults.storageEncrypted;
this.tags = defaults.tags;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder allocatedStorage(Integer allocatedStorage) {
if (allocatedStorage == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "allocatedStorage");
}
this.allocatedStorage = allocatedStorage;
return this;
}
@CustomType.Setter
public Builder availabilityZones(List availabilityZones) {
if (availabilityZones == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "availabilityZones");
}
this.availabilityZones = availabilityZones;
return this;
}
public Builder availabilityZones(String... availabilityZones) {
return availabilityZones(List.of(availabilityZones));
}
@CustomType.Setter
public Builder dbClusterIdentifier(@Nullable String dbClusterIdentifier) {
this.dbClusterIdentifier = dbClusterIdentifier;
return this;
}
@CustomType.Setter
public Builder dbClusterSnapshotArn(String dbClusterSnapshotArn) {
if (dbClusterSnapshotArn == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "dbClusterSnapshotArn");
}
this.dbClusterSnapshotArn = dbClusterSnapshotArn;
return this;
}
@CustomType.Setter
public Builder dbClusterSnapshotIdentifier(@Nullable String dbClusterSnapshotIdentifier) {
this.dbClusterSnapshotIdentifier = dbClusterSnapshotIdentifier;
return this;
}
@CustomType.Setter
public Builder engine(String engine) {
if (engine == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "engine");
}
this.engine = engine;
return this;
}
@CustomType.Setter
public Builder engineVersion(String engineVersion) {
if (engineVersion == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "engineVersion");
}
this.engineVersion = engineVersion;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder includePublic(@Nullable Boolean includePublic) {
this.includePublic = includePublic;
return this;
}
@CustomType.Setter
public Builder includeShared(@Nullable Boolean includeShared) {
this.includeShared = includeShared;
return this;
}
@CustomType.Setter
public Builder kmsKeyId(String kmsKeyId) {
if (kmsKeyId == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "kmsKeyId");
}
this.kmsKeyId = kmsKeyId;
return this;
}
@CustomType.Setter
public Builder licenseModel(String licenseModel) {
if (licenseModel == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "licenseModel");
}
this.licenseModel = licenseModel;
return this;
}
@CustomType.Setter
public Builder mostRecent(@Nullable Boolean mostRecent) {
this.mostRecent = mostRecent;
return this;
}
@CustomType.Setter
public Builder port(Integer port) {
if (port == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder snapshotCreateTime(String snapshotCreateTime) {
if (snapshotCreateTime == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "snapshotCreateTime");
}
this.snapshotCreateTime = snapshotCreateTime;
return this;
}
@CustomType.Setter
public Builder snapshotType(@Nullable String snapshotType) {
this.snapshotType = snapshotType;
return this;
}
@CustomType.Setter
public Builder sourceDbClusterSnapshotArn(String sourceDbClusterSnapshotArn) {
if (sourceDbClusterSnapshotArn == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "sourceDbClusterSnapshotArn");
}
this.sourceDbClusterSnapshotArn = sourceDbClusterSnapshotArn;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder storageEncrypted(Boolean storageEncrypted) {
if (storageEncrypted == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "storageEncrypted");
}
this.storageEncrypted = storageEncrypted;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder vpcId(String vpcId) {
if (vpcId == null) {
throw new MissingRequiredPropertyException("GetClusterSnapshotResult", "vpcId");
}
this.vpcId = vpcId;
return this;
}
public GetClusterSnapshotResult build() {
final var _resultValue = new GetClusterSnapshotResult();
_resultValue.allocatedStorage = allocatedStorage;
_resultValue.availabilityZones = availabilityZones;
_resultValue.dbClusterIdentifier = dbClusterIdentifier;
_resultValue.dbClusterSnapshotArn = dbClusterSnapshotArn;
_resultValue.dbClusterSnapshotIdentifier = dbClusterSnapshotIdentifier;
_resultValue.engine = engine;
_resultValue.engineVersion = engineVersion;
_resultValue.id = id;
_resultValue.includePublic = includePublic;
_resultValue.includeShared = includeShared;
_resultValue.kmsKeyId = kmsKeyId;
_resultValue.licenseModel = licenseModel;
_resultValue.mostRecent = mostRecent;
_resultValue.port = port;
_resultValue.snapshotCreateTime = snapshotCreateTime;
_resultValue.snapshotType = snapshotType;
_resultValue.sourceDbClusterSnapshotArn = sourceDbClusterSnapshotArn;
_resultValue.status = status;
_resultValue.storageEncrypted = storageEncrypted;
_resultValue.tags = tags;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy