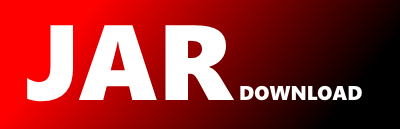
com.pulumi.aws.rds.outputs.InstanceS3Import Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InstanceS3Import {
/**
* @return The bucket name where your backup is stored
*
*/
private String bucketName;
/**
* @return Can be blank, but is the path to your backup
*
*/
private @Nullable String bucketPrefix;
/**
* @return Role applied to load the data.
*
*/
private String ingestionRole;
/**
* @return Source engine for the backup
*
*/
private String sourceEngine;
/**
* @return Version of the source engine used to make the backup
*
* This will not recreate the resource if the S3 object changes in some way. It's only used to initialize the database.
*
*/
private String sourceEngineVersion;
private InstanceS3Import() {}
/**
* @return The bucket name where your backup is stored
*
*/
public String bucketName() {
return this.bucketName;
}
/**
* @return Can be blank, but is the path to your backup
*
*/
public Optional bucketPrefix() {
return Optional.ofNullable(this.bucketPrefix);
}
/**
* @return Role applied to load the data.
*
*/
public String ingestionRole() {
return this.ingestionRole;
}
/**
* @return Source engine for the backup
*
*/
public String sourceEngine() {
return this.sourceEngine;
}
/**
* @return Version of the source engine used to make the backup
*
* This will not recreate the resource if the S3 object changes in some way. It's only used to initialize the database.
*
*/
public String sourceEngineVersion() {
return this.sourceEngineVersion;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InstanceS3Import defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String bucketName;
private @Nullable String bucketPrefix;
private String ingestionRole;
private String sourceEngine;
private String sourceEngineVersion;
public Builder() {}
public Builder(InstanceS3Import defaults) {
Objects.requireNonNull(defaults);
this.bucketName = defaults.bucketName;
this.bucketPrefix = defaults.bucketPrefix;
this.ingestionRole = defaults.ingestionRole;
this.sourceEngine = defaults.sourceEngine;
this.sourceEngineVersion = defaults.sourceEngineVersion;
}
@CustomType.Setter
public Builder bucketName(String bucketName) {
if (bucketName == null) {
throw new MissingRequiredPropertyException("InstanceS3Import", "bucketName");
}
this.bucketName = bucketName;
return this;
}
@CustomType.Setter
public Builder bucketPrefix(@Nullable String bucketPrefix) {
this.bucketPrefix = bucketPrefix;
return this;
}
@CustomType.Setter
public Builder ingestionRole(String ingestionRole) {
if (ingestionRole == null) {
throw new MissingRequiredPropertyException("InstanceS3Import", "ingestionRole");
}
this.ingestionRole = ingestionRole;
return this;
}
@CustomType.Setter
public Builder sourceEngine(String sourceEngine) {
if (sourceEngine == null) {
throw new MissingRequiredPropertyException("InstanceS3Import", "sourceEngine");
}
this.sourceEngine = sourceEngine;
return this;
}
@CustomType.Setter
public Builder sourceEngineVersion(String sourceEngineVersion) {
if (sourceEngineVersion == null) {
throw new MissingRequiredPropertyException("InstanceS3Import", "sourceEngineVersion");
}
this.sourceEngineVersion = sourceEngineVersion;
return this;
}
public InstanceS3Import build() {
final var _resultValue = new InstanceS3Import();
_resultValue.bucketName = bucketName;
_resultValue.bucketPrefix = bucketPrefix;
_resultValue.ingestionRole = ingestionRole;
_resultValue.sourceEngine = sourceEngine;
_resultValue.sourceEngineVersion = sourceEngineVersion;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy