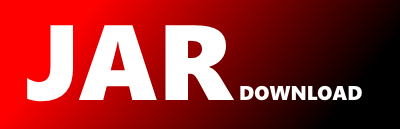
com.pulumi.aws.route53.outputs.GetResolverFirewallRulesFirewallRule Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.route53.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetResolverFirewallRulesFirewallRule {
/**
* @return The action that DNS Firewall should take on a DNS query when it matches one of the domains in the rule's domain list.
*
*/
private String action;
/**
* @return The DNS record's type.
*
*/
private String blockOverrideDnsType;
/**
* @return The custom DNS record to send back in response to the query.
*
*/
private String blockOverrideDomain;
/**
* @return The recommended amount of time, in seconds, for the DNS resolver or web browser to cache the provided override record.
*
*/
private Integer blockOverrideTtl;
/**
* @return The way that you want DNS Firewall to block the request.
*
*/
private String blockResponse;
/**
* @return The date and time that the rule was created, in Unix time format and Coordinated Universal Time (UTC).
*
*/
private String creationTime;
/**
* @return A unique string defined by you to identify the request.
*
*/
private String creatorRequestId;
/**
* @return The ID of the domain list that's used in the rule.
*
*/
private String firewallDomainListId;
/**
* @return The unique identifier of the firewall rule group that you want to retrieve the rules for.
*
*/
private String firewallRuleGroupId;
/**
* @return The date and time that the rule was last modified, in Unix time format and Coordinated Universal Time (UTC).
*
*/
private String modificationTime;
/**
* @return The name of the rule.
*
*/
private String name;
/**
* @return The setting that determines the processing order of the rules in a rule group.
*
*/
private Integer priority;
private GetResolverFirewallRulesFirewallRule() {}
/**
* @return The action that DNS Firewall should take on a DNS query when it matches one of the domains in the rule's domain list.
*
*/
public String action() {
return this.action;
}
/**
* @return The DNS record's type.
*
*/
public String blockOverrideDnsType() {
return this.blockOverrideDnsType;
}
/**
* @return The custom DNS record to send back in response to the query.
*
*/
public String blockOverrideDomain() {
return this.blockOverrideDomain;
}
/**
* @return The recommended amount of time, in seconds, for the DNS resolver or web browser to cache the provided override record.
*
*/
public Integer blockOverrideTtl() {
return this.blockOverrideTtl;
}
/**
* @return The way that you want DNS Firewall to block the request.
*
*/
public String blockResponse() {
return this.blockResponse;
}
/**
* @return The date and time that the rule was created, in Unix time format and Coordinated Universal Time (UTC).
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return A unique string defined by you to identify the request.
*
*/
public String creatorRequestId() {
return this.creatorRequestId;
}
/**
* @return The ID of the domain list that's used in the rule.
*
*/
public String firewallDomainListId() {
return this.firewallDomainListId;
}
/**
* @return The unique identifier of the firewall rule group that you want to retrieve the rules for.
*
*/
public String firewallRuleGroupId() {
return this.firewallRuleGroupId;
}
/**
* @return The date and time that the rule was last modified, in Unix time format and Coordinated Universal Time (UTC).
*
*/
public String modificationTime() {
return this.modificationTime;
}
/**
* @return The name of the rule.
*
*/
public String name() {
return this.name;
}
/**
* @return The setting that determines the processing order of the rules in a rule group.
*
*/
public Integer priority() {
return this.priority;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetResolverFirewallRulesFirewallRule defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String action;
private String blockOverrideDnsType;
private String blockOverrideDomain;
private Integer blockOverrideTtl;
private String blockResponse;
private String creationTime;
private String creatorRequestId;
private String firewallDomainListId;
private String firewallRuleGroupId;
private String modificationTime;
private String name;
private Integer priority;
public Builder() {}
public Builder(GetResolverFirewallRulesFirewallRule defaults) {
Objects.requireNonNull(defaults);
this.action = defaults.action;
this.blockOverrideDnsType = defaults.blockOverrideDnsType;
this.blockOverrideDomain = defaults.blockOverrideDomain;
this.blockOverrideTtl = defaults.blockOverrideTtl;
this.blockResponse = defaults.blockResponse;
this.creationTime = defaults.creationTime;
this.creatorRequestId = defaults.creatorRequestId;
this.firewallDomainListId = defaults.firewallDomainListId;
this.firewallRuleGroupId = defaults.firewallRuleGroupId;
this.modificationTime = defaults.modificationTime;
this.name = defaults.name;
this.priority = defaults.priority;
}
@CustomType.Setter
public Builder action(String action) {
if (action == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "action");
}
this.action = action;
return this;
}
@CustomType.Setter
public Builder blockOverrideDnsType(String blockOverrideDnsType) {
if (blockOverrideDnsType == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "blockOverrideDnsType");
}
this.blockOverrideDnsType = blockOverrideDnsType;
return this;
}
@CustomType.Setter
public Builder blockOverrideDomain(String blockOverrideDomain) {
if (blockOverrideDomain == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "blockOverrideDomain");
}
this.blockOverrideDomain = blockOverrideDomain;
return this;
}
@CustomType.Setter
public Builder blockOverrideTtl(Integer blockOverrideTtl) {
if (blockOverrideTtl == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "blockOverrideTtl");
}
this.blockOverrideTtl = blockOverrideTtl;
return this;
}
@CustomType.Setter
public Builder blockResponse(String blockResponse) {
if (blockResponse == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "blockResponse");
}
this.blockResponse = blockResponse;
return this;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder creatorRequestId(String creatorRequestId) {
if (creatorRequestId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "creatorRequestId");
}
this.creatorRequestId = creatorRequestId;
return this;
}
@CustomType.Setter
public Builder firewallDomainListId(String firewallDomainListId) {
if (firewallDomainListId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "firewallDomainListId");
}
this.firewallDomainListId = firewallDomainListId;
return this;
}
@CustomType.Setter
public Builder firewallRuleGroupId(String firewallRuleGroupId) {
if (firewallRuleGroupId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "firewallRuleGroupId");
}
this.firewallRuleGroupId = firewallRuleGroupId;
return this;
}
@CustomType.Setter
public Builder modificationTime(String modificationTime) {
if (modificationTime == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "modificationTime");
}
this.modificationTime = modificationTime;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(Integer priority) {
if (priority == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRulesFirewallRule", "priority");
}
this.priority = priority;
return this;
}
public GetResolverFirewallRulesFirewallRule build() {
final var _resultValue = new GetResolverFirewallRulesFirewallRule();
_resultValue.action = action;
_resultValue.blockOverrideDnsType = blockOverrideDnsType;
_resultValue.blockOverrideDomain = blockOverrideDomain;
_resultValue.blockOverrideTtl = blockOverrideTtl;
_resultValue.blockResponse = blockResponse;
_resultValue.creationTime = creationTime;
_resultValue.creatorRequestId = creatorRequestId;
_resultValue.firewallDomainListId = firewallDomainListId;
_resultValue.firewallRuleGroupId = firewallRuleGroupId;
_resultValue.modificationTime = modificationTime;
_resultValue.name = name;
_resultValue.priority = priority;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy