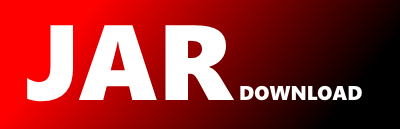
com.pulumi.aws.route53recoverycontrol.inputs.SafetyRuleState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.route53recoverycontrol.inputs;
import com.pulumi.aws.route53recoverycontrol.inputs.SafetyRuleRuleConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SafetyRuleState extends com.pulumi.resources.ResourceArgs {
public static final SafetyRuleState Empty = new SafetyRuleState();
/**
* ARN of the safety rule.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return ARN of the safety rule.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy