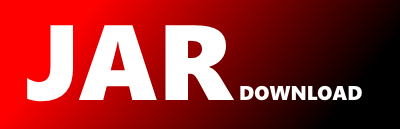
com.pulumi.aws.s3.inputs.GetBucketObjectsPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3.inputs;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetBucketObjectsPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetBucketObjectsPlainArgs Empty = new GetBucketObjectsPlainArgs();
/**
* Lists object keys in this S3 bucket. Alternatively, an [S3 access point](https://docs.aws.amazon.com/AmazonS3/latest/dev/using-access-points.html) ARN can be specified
*
* @deprecated
* Use the aws.s3.getObjects data source instead
*
*/
@Deprecated /* Use the aws.s3.getObjects data source instead */
@Import(name="bucket", required=true)
private String bucket;
/**
* @return Lists object keys in this S3 bucket. Alternatively, an [S3 access point](https://docs.aws.amazon.com/AmazonS3/latest/dev/using-access-points.html) ARN can be specified
*
* @deprecated
* Use the aws.s3.getObjects data source instead
*
*/
@Deprecated /* Use the aws.s3.getObjects data source instead */
public String bucket() {
return this.bucket;
}
/**
* Character used to group keys (Default: none)
*
*/
@Import(name="delimiter")
private @Nullable String delimiter;
/**
* @return Character used to group keys (Default: none)
*
*/
public Optional delimiter() {
return Optional.ofNullable(this.delimiter);
}
/**
* Encodes keys using this method (Default: none; besides none, only "url" can be used)
*
*/
@Import(name="encodingType")
private @Nullable String encodingType;
/**
* @return Encodes keys using this method (Default: none; besides none, only "url" can be used)
*
*/
public Optional encodingType() {
return Optional.ofNullable(this.encodingType);
}
/**
* Boolean specifying whether to populate the owner list (Default: false)
*
*/
@Import(name="fetchOwner")
private @Nullable Boolean fetchOwner;
/**
* @return Boolean specifying whether to populate the owner list (Default: false)
*
*/
public Optional fetchOwner() {
return Optional.ofNullable(this.fetchOwner);
}
/**
* Maximum object keys to return (Default: 1000)
*
*/
@Import(name="maxKeys")
private @Nullable Integer maxKeys;
/**
* @return Maximum object keys to return (Default: 1000)
*
*/
public Optional maxKeys() {
return Optional.ofNullable(this.maxKeys);
}
/**
* Limits results to object keys with this prefix (Default: none)
*
*/
@Import(name="prefix")
private @Nullable String prefix;
/**
* @return Limits results to object keys with this prefix (Default: none)
*
*/
public Optional prefix() {
return Optional.ofNullable(this.prefix);
}
/**
* Returns key names lexicographically after a specific object key in your bucket (Default: none; S3 lists object keys in UTF-8 character encoding in lexicographical order)
*
*/
@Import(name="startAfter")
private @Nullable String startAfter;
/**
* @return Returns key names lexicographically after a specific object key in your bucket (Default: none; S3 lists object keys in UTF-8 character encoding in lexicographical order)
*
*/
public Optional startAfter() {
return Optional.ofNullable(this.startAfter);
}
private GetBucketObjectsPlainArgs() {}
private GetBucketObjectsPlainArgs(GetBucketObjectsPlainArgs $) {
this.bucket = $.bucket;
this.delimiter = $.delimiter;
this.encodingType = $.encodingType;
this.fetchOwner = $.fetchOwner;
this.maxKeys = $.maxKeys;
this.prefix = $.prefix;
this.startAfter = $.startAfter;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBucketObjectsPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetBucketObjectsPlainArgs $;
public Builder() {
$ = new GetBucketObjectsPlainArgs();
}
public Builder(GetBucketObjectsPlainArgs defaults) {
$ = new GetBucketObjectsPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param bucket Lists object keys in this S3 bucket. Alternatively, an [S3 access point](https://docs.aws.amazon.com/AmazonS3/latest/dev/using-access-points.html) ARN can be specified
*
* @return builder
*
* @deprecated
* Use the aws.s3.getObjects data source instead
*
*/
@Deprecated /* Use the aws.s3.getObjects data source instead */
public Builder bucket(String bucket) {
$.bucket = bucket;
return this;
}
/**
* @param delimiter Character used to group keys (Default: none)
*
* @return builder
*
*/
public Builder delimiter(@Nullable String delimiter) {
$.delimiter = delimiter;
return this;
}
/**
* @param encodingType Encodes keys using this method (Default: none; besides none, only "url" can be used)
*
* @return builder
*
*/
public Builder encodingType(@Nullable String encodingType) {
$.encodingType = encodingType;
return this;
}
/**
* @param fetchOwner Boolean specifying whether to populate the owner list (Default: false)
*
* @return builder
*
*/
public Builder fetchOwner(@Nullable Boolean fetchOwner) {
$.fetchOwner = fetchOwner;
return this;
}
/**
* @param maxKeys Maximum object keys to return (Default: 1000)
*
* @return builder
*
*/
public Builder maxKeys(@Nullable Integer maxKeys) {
$.maxKeys = maxKeys;
return this;
}
/**
* @param prefix Limits results to object keys with this prefix (Default: none)
*
* @return builder
*
*/
public Builder prefix(@Nullable String prefix) {
$.prefix = prefix;
return this;
}
/**
* @param startAfter Returns key names lexicographically after a specific object key in your bucket (Default: none; S3 lists object keys in UTF-8 character encoding in lexicographical order)
*
* @return builder
*
*/
public Builder startAfter(@Nullable String startAfter) {
$.startAfter = startAfter;
return this;
}
public GetBucketObjectsPlainArgs build() {
if ($.bucket == null) {
throw new MissingRequiredPropertyException("GetBucketObjectsPlainArgs", "bucket");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy