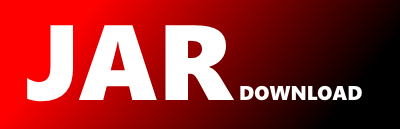
com.pulumi.aws.s3.outputs.BucketReplicationConfigRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3.outputs;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDeleteMarkerReplication;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDestination;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleExistingObjectReplication;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleFilter;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleSourceSelectionCriteria;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BucketReplicationConfigRule {
/**
* @return Whether delete markers are replicated. This argument is only valid with V2 replication configurations (i.e., when `filter` is used)documented below.
*
*/
private @Nullable BucketReplicationConfigRuleDeleteMarkerReplication deleteMarkerReplication;
/**
* @return Specifies the destination for the rule. See below.
*
*/
private BucketReplicationConfigRuleDestination destination;
/**
* @return Replicate existing objects in the source bucket according to the rule configurations. See below.
*
*/
private @Nullable BucketReplicationConfigRuleExistingObjectReplication existingObjectReplication;
/**
* @return Filter that identifies subset of objects to which the replication rule applies. See below. If not specified, the `rule` will default to using `prefix`.
*
*/
private @Nullable BucketReplicationConfigRuleFilter filter;
/**
* @return Unique identifier for the rule. Must be less than or equal to 255 characters in length.
*
*/
private @Nullable String id;
/**
* @return Object key name prefix identifying one or more objects to which the rule applies. Must be less than or equal to 1024 characters in length. Defaults to an empty string (`""`) if `filter` is not specified.
*
* @deprecated
* Use filter instead
*
*/
@Deprecated /* Use filter instead */
private @Nullable String prefix;
/**
* @return Priority associated with the rule. Priority should only be set if `filter` is configured. If not provided, defaults to `0`. Priority must be unique between multiple rules.
*
*/
private @Nullable Integer priority;
/**
* @return Specifies special object selection criteria. See below.
*
*/
private @Nullable BucketReplicationConfigRuleSourceSelectionCriteria sourceSelectionCriteria;
/**
* @return Status of the rule. Either `"Enabled"` or `"Disabled"`. The rule is ignored if status is not "Enabled".
*
*/
private String status;
private BucketReplicationConfigRule() {}
/**
* @return Whether delete markers are replicated. This argument is only valid with V2 replication configurations (i.e., when `filter` is used)documented below.
*
*/
public Optional deleteMarkerReplication() {
return Optional.ofNullable(this.deleteMarkerReplication);
}
/**
* @return Specifies the destination for the rule. See below.
*
*/
public BucketReplicationConfigRuleDestination destination() {
return this.destination;
}
/**
* @return Replicate existing objects in the source bucket according to the rule configurations. See below.
*
*/
public Optional existingObjectReplication() {
return Optional.ofNullable(this.existingObjectReplication);
}
/**
* @return Filter that identifies subset of objects to which the replication rule applies. See below. If not specified, the `rule` will default to using `prefix`.
*
*/
public Optional filter() {
return Optional.ofNullable(this.filter);
}
/**
* @return Unique identifier for the rule. Must be less than or equal to 255 characters in length.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Object key name prefix identifying one or more objects to which the rule applies. Must be less than or equal to 1024 characters in length. Defaults to an empty string (`""`) if `filter` is not specified.
*
* @deprecated
* Use filter instead
*
*/
@Deprecated /* Use filter instead */
public Optional prefix() {
return Optional.ofNullable(this.prefix);
}
/**
* @return Priority associated with the rule. Priority should only be set if `filter` is configured. If not provided, defaults to `0`. Priority must be unique between multiple rules.
*
*/
public Optional priority() {
return Optional.ofNullable(this.priority);
}
/**
* @return Specifies special object selection criteria. See below.
*
*/
public Optional sourceSelectionCriteria() {
return Optional.ofNullable(this.sourceSelectionCriteria);
}
/**
* @return Status of the rule. Either `"Enabled"` or `"Disabled"`. The rule is ignored if status is not "Enabled".
*
*/
public String status() {
return this.status;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BucketReplicationConfigRule defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable BucketReplicationConfigRuleDeleteMarkerReplication deleteMarkerReplication;
private BucketReplicationConfigRuleDestination destination;
private @Nullable BucketReplicationConfigRuleExistingObjectReplication existingObjectReplication;
private @Nullable BucketReplicationConfigRuleFilter filter;
private @Nullable String id;
private @Nullable String prefix;
private @Nullable Integer priority;
private @Nullable BucketReplicationConfigRuleSourceSelectionCriteria sourceSelectionCriteria;
private String status;
public Builder() {}
public Builder(BucketReplicationConfigRule defaults) {
Objects.requireNonNull(defaults);
this.deleteMarkerReplication = defaults.deleteMarkerReplication;
this.destination = defaults.destination;
this.existingObjectReplication = defaults.existingObjectReplication;
this.filter = defaults.filter;
this.id = defaults.id;
this.prefix = defaults.prefix;
this.priority = defaults.priority;
this.sourceSelectionCriteria = defaults.sourceSelectionCriteria;
this.status = defaults.status;
}
@CustomType.Setter
public Builder deleteMarkerReplication(@Nullable BucketReplicationConfigRuleDeleteMarkerReplication deleteMarkerReplication) {
this.deleteMarkerReplication = deleteMarkerReplication;
return this;
}
@CustomType.Setter
public Builder destination(BucketReplicationConfigRuleDestination destination) {
if (destination == null) {
throw new MissingRequiredPropertyException("BucketReplicationConfigRule", "destination");
}
this.destination = destination;
return this;
}
@CustomType.Setter
public Builder existingObjectReplication(@Nullable BucketReplicationConfigRuleExistingObjectReplication existingObjectReplication) {
this.existingObjectReplication = existingObjectReplication;
return this;
}
@CustomType.Setter
public Builder filter(@Nullable BucketReplicationConfigRuleFilter filter) {
this.filter = filter;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder prefix(@Nullable String prefix) {
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder priority(@Nullable Integer priority) {
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder sourceSelectionCriteria(@Nullable BucketReplicationConfigRuleSourceSelectionCriteria sourceSelectionCriteria) {
this.sourceSelectionCriteria = sourceSelectionCriteria;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("BucketReplicationConfigRule", "status");
}
this.status = status;
return this;
}
public BucketReplicationConfigRule build() {
final var _resultValue = new BucketReplicationConfigRule();
_resultValue.deleteMarkerReplication = deleteMarkerReplication;
_resultValue.destination = destination;
_resultValue.existingObjectReplication = existingObjectReplication;
_resultValue.filter = filter;
_resultValue.id = id;
_resultValue.prefix = prefix;
_resultValue.priority = priority;
_resultValue.sourceSelectionCriteria = sourceSelectionCriteria;
_resultValue.status = status;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy