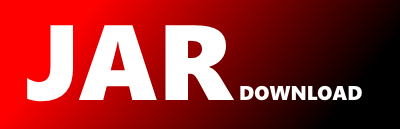
com.pulumi.aws.s3.outputs.GetBucketResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetBucketResult {
/**
* @return ARN of the bucket. Will be of format `arn:aws:s3:::bucketname`.
*
*/
private String arn;
private String bucket;
/**
* @return Bucket domain name. Will be of format `bucketname.s3.amazonaws.com`.
*
*/
private String bucketDomainName;
/**
* @return The bucket region-specific domain name. The bucket domain name including the region name. Please refer to the [S3 endpoints reference](https://docs.aws.amazon.com/general/latest/gr/s3.html#s3_region) for format. Note: AWS CloudFront allows specifying an S3 region-specific endpoint when creating an S3 origin. This will prevent redirect issues from CloudFront to the S3 Origin URL. For more information, see the [Virtual Hosted-Style Requests for Other Regions](https://docs.aws.amazon.com/AmazonS3/latest/userguide/VirtualHosting.html#deprecated-global-endpoint) section in the AWS S3 User Guide.
*
*/
private String bucketRegionalDomainName;
/**
* @return The [Route 53 Hosted Zone ID](https://docs.aws.amazon.com/general/latest/gr/rande.html#s3_website_region_endpoints) for this bucket's region.
*
*/
private String hostedZoneId;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return AWS region this bucket resides in.
*
*/
private String region;
/**
* @return Domain of the website endpoint, if the bucket is configured with a website. If not, this will be an empty string. This is used to create Route 53 alias records.
*
*/
private String websiteDomain;
/**
* @return Website endpoint, if the bucket is configured with a website. If not, this will be an empty string.
*
*/
private String websiteEndpoint;
private GetBucketResult() {}
/**
* @return ARN of the bucket. Will be of format `arn:aws:s3:::bucketname`.
*
*/
public String arn() {
return this.arn;
}
public String bucket() {
return this.bucket;
}
/**
* @return Bucket domain name. Will be of format `bucketname.s3.amazonaws.com`.
*
*/
public String bucketDomainName() {
return this.bucketDomainName;
}
/**
* @return The bucket region-specific domain name. The bucket domain name including the region name. Please refer to the [S3 endpoints reference](https://docs.aws.amazon.com/general/latest/gr/s3.html#s3_region) for format. Note: AWS CloudFront allows specifying an S3 region-specific endpoint when creating an S3 origin. This will prevent redirect issues from CloudFront to the S3 Origin URL. For more information, see the [Virtual Hosted-Style Requests for Other Regions](https://docs.aws.amazon.com/AmazonS3/latest/userguide/VirtualHosting.html#deprecated-global-endpoint) section in the AWS S3 User Guide.
*
*/
public String bucketRegionalDomainName() {
return this.bucketRegionalDomainName;
}
/**
* @return The [Route 53 Hosted Zone ID](https://docs.aws.amazon.com/general/latest/gr/rande.html#s3_website_region_endpoints) for this bucket's region.
*
*/
public String hostedZoneId() {
return this.hostedZoneId;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return AWS region this bucket resides in.
*
*/
public String region() {
return this.region;
}
/**
* @return Domain of the website endpoint, if the bucket is configured with a website. If not, this will be an empty string. This is used to create Route 53 alias records.
*
*/
public String websiteDomain() {
return this.websiteDomain;
}
/**
* @return Website endpoint, if the bucket is configured with a website. If not, this will be an empty string.
*
*/
public String websiteEndpoint() {
return this.websiteEndpoint;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBucketResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String bucket;
private String bucketDomainName;
private String bucketRegionalDomainName;
private String hostedZoneId;
private String id;
private String region;
private String websiteDomain;
private String websiteEndpoint;
public Builder() {}
public Builder(GetBucketResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.bucket = defaults.bucket;
this.bucketDomainName = defaults.bucketDomainName;
this.bucketRegionalDomainName = defaults.bucketRegionalDomainName;
this.hostedZoneId = defaults.hostedZoneId;
this.id = defaults.id;
this.region = defaults.region;
this.websiteDomain = defaults.websiteDomain;
this.websiteEndpoint = defaults.websiteEndpoint;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder bucket(String bucket) {
if (bucket == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "bucket");
}
this.bucket = bucket;
return this;
}
@CustomType.Setter
public Builder bucketDomainName(String bucketDomainName) {
if (bucketDomainName == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "bucketDomainName");
}
this.bucketDomainName = bucketDomainName;
return this;
}
@CustomType.Setter
public Builder bucketRegionalDomainName(String bucketRegionalDomainName) {
if (bucketRegionalDomainName == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "bucketRegionalDomainName");
}
this.bucketRegionalDomainName = bucketRegionalDomainName;
return this;
}
@CustomType.Setter
public Builder hostedZoneId(String hostedZoneId) {
if (hostedZoneId == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "hostedZoneId");
}
this.hostedZoneId = hostedZoneId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder region(String region) {
if (region == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "region");
}
this.region = region;
return this;
}
@CustomType.Setter
public Builder websiteDomain(String websiteDomain) {
if (websiteDomain == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "websiteDomain");
}
this.websiteDomain = websiteDomain;
return this;
}
@CustomType.Setter
public Builder websiteEndpoint(String websiteEndpoint) {
if (websiteEndpoint == null) {
throw new MissingRequiredPropertyException("GetBucketResult", "websiteEndpoint");
}
this.websiteEndpoint = websiteEndpoint;
return this;
}
public GetBucketResult build() {
final var _resultValue = new GetBucketResult();
_resultValue.arn = arn;
_resultValue.bucket = bucket;
_resultValue.bucketDomainName = bucketDomainName;
_resultValue.bucketRegionalDomainName = bucketRegionalDomainName;
_resultValue.hostedZoneId = hostedZoneId;
_resultValue.id = id;
_resultValue.region = region;
_resultValue.websiteDomain = websiteDomain;
_resultValue.websiteEndpoint = websiteEndpoint;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy