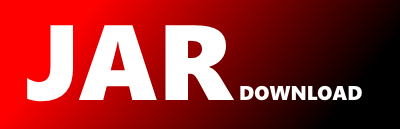
com.pulumi.aws.s3.outputs.GetObjectsResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetObjectsResult {
private String bucket;
/**
* @return List of any keys between `prefix` and the next occurrence of `delimiter` (i.e., similar to subdirectories of the `prefix` "directory"); the list is only returned when you specify `delimiter`
*
*/
private List commonPrefixes;
private @Nullable String delimiter;
private @Nullable String encodingType;
private @Nullable Boolean fetchOwner;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return List of strings representing object keys
*
*/
private List keys;
private @Nullable Integer maxKeys;
/**
* @return List of strings representing object owner IDs (see `fetch_owner` above)
*
*/
private List owners;
private @Nullable String prefix;
/**
* @return If present, indicates that the requester was successfully charged for the request.
*
*/
private String requestCharged;
private @Nullable String requestPayer;
private @Nullable String startAfter;
private GetObjectsResult() {}
public String bucket() {
return this.bucket;
}
/**
* @return List of any keys between `prefix` and the next occurrence of `delimiter` (i.e., similar to subdirectories of the `prefix` "directory"); the list is only returned when you specify `delimiter`
*
*/
public List commonPrefixes() {
return this.commonPrefixes;
}
public Optional delimiter() {
return Optional.ofNullable(this.delimiter);
}
public Optional encodingType() {
return Optional.ofNullable(this.encodingType);
}
public Optional fetchOwner() {
return Optional.ofNullable(this.fetchOwner);
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return List of strings representing object keys
*
*/
public List keys() {
return this.keys;
}
public Optional maxKeys() {
return Optional.ofNullable(this.maxKeys);
}
/**
* @return List of strings representing object owner IDs (see `fetch_owner` above)
*
*/
public List owners() {
return this.owners;
}
public Optional prefix() {
return Optional.ofNullable(this.prefix);
}
/**
* @return If present, indicates that the requester was successfully charged for the request.
*
*/
public String requestCharged() {
return this.requestCharged;
}
public Optional requestPayer() {
return Optional.ofNullable(this.requestPayer);
}
public Optional startAfter() {
return Optional.ofNullable(this.startAfter);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetObjectsResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String bucket;
private List commonPrefixes;
private @Nullable String delimiter;
private @Nullable String encodingType;
private @Nullable Boolean fetchOwner;
private String id;
private List keys;
private @Nullable Integer maxKeys;
private List owners;
private @Nullable String prefix;
private String requestCharged;
private @Nullable String requestPayer;
private @Nullable String startAfter;
public Builder() {}
public Builder(GetObjectsResult defaults) {
Objects.requireNonNull(defaults);
this.bucket = defaults.bucket;
this.commonPrefixes = defaults.commonPrefixes;
this.delimiter = defaults.delimiter;
this.encodingType = defaults.encodingType;
this.fetchOwner = defaults.fetchOwner;
this.id = defaults.id;
this.keys = defaults.keys;
this.maxKeys = defaults.maxKeys;
this.owners = defaults.owners;
this.prefix = defaults.prefix;
this.requestCharged = defaults.requestCharged;
this.requestPayer = defaults.requestPayer;
this.startAfter = defaults.startAfter;
}
@CustomType.Setter
public Builder bucket(String bucket) {
if (bucket == null) {
throw new MissingRequiredPropertyException("GetObjectsResult", "bucket");
}
this.bucket = bucket;
return this;
}
@CustomType.Setter
public Builder commonPrefixes(List commonPrefixes) {
if (commonPrefixes == null) {
throw new MissingRequiredPropertyException("GetObjectsResult", "commonPrefixes");
}
this.commonPrefixes = commonPrefixes;
return this;
}
public Builder commonPrefixes(String... commonPrefixes) {
return commonPrefixes(List.of(commonPrefixes));
}
@CustomType.Setter
public Builder delimiter(@Nullable String delimiter) {
this.delimiter = delimiter;
return this;
}
@CustomType.Setter
public Builder encodingType(@Nullable String encodingType) {
this.encodingType = encodingType;
return this;
}
@CustomType.Setter
public Builder fetchOwner(@Nullable Boolean fetchOwner) {
this.fetchOwner = fetchOwner;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetObjectsResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder keys(List keys) {
if (keys == null) {
throw new MissingRequiredPropertyException("GetObjectsResult", "keys");
}
this.keys = keys;
return this;
}
public Builder keys(String... keys) {
return keys(List.of(keys));
}
@CustomType.Setter
public Builder maxKeys(@Nullable Integer maxKeys) {
this.maxKeys = maxKeys;
return this;
}
@CustomType.Setter
public Builder owners(List owners) {
if (owners == null) {
throw new MissingRequiredPropertyException("GetObjectsResult", "owners");
}
this.owners = owners;
return this;
}
public Builder owners(String... owners) {
return owners(List.of(owners));
}
@CustomType.Setter
public Builder prefix(@Nullable String prefix) {
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder requestCharged(String requestCharged) {
if (requestCharged == null) {
throw new MissingRequiredPropertyException("GetObjectsResult", "requestCharged");
}
this.requestCharged = requestCharged;
return this;
}
@CustomType.Setter
public Builder requestPayer(@Nullable String requestPayer) {
this.requestPayer = requestPayer;
return this;
}
@CustomType.Setter
public Builder startAfter(@Nullable String startAfter) {
this.startAfter = startAfter;
return this;
}
public GetObjectsResult build() {
final var _resultValue = new GetObjectsResult();
_resultValue.bucket = bucket;
_resultValue.commonPrefixes = commonPrefixes;
_resultValue.delimiter = delimiter;
_resultValue.encodingType = encodingType;
_resultValue.fetchOwner = fetchOwner;
_resultValue.id = id;
_resultValue.keys = keys;
_resultValue.maxKeys = maxKeys;
_resultValue.owners = owners;
_resultValue.prefix = prefix;
_resultValue.requestCharged = requestCharged;
_resultValue.requestPayer = requestPayer;
_resultValue.startAfter = startAfter;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy