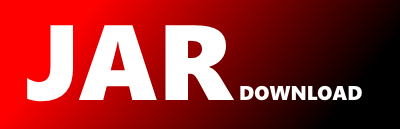
com.pulumi.aws.s3control.inputs.StorageLensConfigurationStorageLensConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3control.inputs;
import com.pulumi.aws.s3control.inputs.StorageLensConfigurationStorageLensConfigurationAccountLevelArgs;
import com.pulumi.aws.s3control.inputs.StorageLensConfigurationStorageLensConfigurationAwsOrgArgs;
import com.pulumi.aws.s3control.inputs.StorageLensConfigurationStorageLensConfigurationDataExportArgs;
import com.pulumi.aws.s3control.inputs.StorageLensConfigurationStorageLensConfigurationExcludeArgs;
import com.pulumi.aws.s3control.inputs.StorageLensConfigurationStorageLensConfigurationIncludeArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StorageLensConfigurationStorageLensConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final StorageLensConfigurationStorageLensConfigurationArgs Empty = new StorageLensConfigurationStorageLensConfigurationArgs();
/**
* The account-level configurations of the S3 Storage Lens configuration. See Account Level below for more details.
*
*/
@Import(name="accountLevel", required=true)
private Output accountLevel;
/**
* @return The account-level configurations of the S3 Storage Lens configuration. See Account Level below for more details.
*
*/
public Output accountLevel() {
return this.accountLevel;
}
/**
* The Amazon Web Services organization for the S3 Storage Lens configuration. See AWS Org below for more details.
*
*/
@Import(name="awsOrg")
private @Nullable Output awsOrg;
/**
* @return The Amazon Web Services organization for the S3 Storage Lens configuration. See AWS Org below for more details.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy