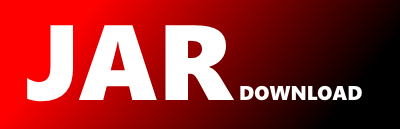
com.pulumi.aws.sagemaker.WorkteamArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker;
import com.pulumi.aws.sagemaker.inputs.WorkteamMemberDefinitionArgs;
import com.pulumi.aws.sagemaker.inputs.WorkteamNotificationConfigurationArgs;
import com.pulumi.aws.sagemaker.inputs.WorkteamWorkerAccessConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkteamArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkteamArgs Empty = new WorkteamArgs();
/**
* A description of the work team.
*
*/
@Import(name="description", required=true)
private Output description;
/**
* @return A description of the work team.
*
*/
public Output description() {
return this.description;
}
/**
* A list of Member Definitions that contains objects that identify the workers that make up the work team. Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces created using Amazon Cognito use `cognito_member_definition`. For workforces created using your own OIDC identity provider (IdP) use `oidc_member_definition`. Do not provide input for both of these parameters in a single request. see Member Definition details below.
*
*/
@Import(name="memberDefinitions", required=true)
private Output> memberDefinitions;
/**
* @return A list of Member Definitions that contains objects that identify the workers that make up the work team. Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces created using Amazon Cognito use `cognito_member_definition`. For workforces created using your own OIDC identity provider (IdP) use `oidc_member_definition`. Do not provide input for both of these parameters in a single request. see Member Definition details below.
*
*/
public Output> memberDefinitions() {
return this.memberDefinitions;
}
/**
* Configures notification of workers regarding available or expiring work items. see Notification Configuration details below.
*
*/
@Import(name="notificationConfiguration")
private @Nullable Output notificationConfiguration;
/**
* @return Configures notification of workers regarding available or expiring work items. see Notification Configuration details below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy