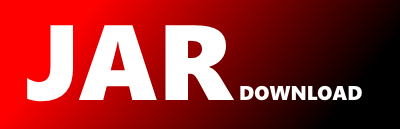
com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker.inputs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsCanvasAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsCodeEditorAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsCustomFileSystemConfigArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsCustomPosixUserConfigArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsJupyterLabAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsJupyterServerAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsKernelGatewayAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsRSessionAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsRStudioServerProAppSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsSharingSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsSpaceStorageSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsStudioWebPortalSettingsArgs;
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsTensorBoardAppSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserProfileUserSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final UserProfileUserSettingsArgs Empty = new UserProfileUserSettingsArgs();
/**
* Indicates whether auto-mounting of an EFS volume is supported for the user profile. The `DefaultAsDomain` value is only supported for user profiles. Do not use the `DefaultAsDomain` value when setting this parameter for a domain. Valid values are: `Enabled`, `Disabled`, and `DefaultAsDomain`.
*
*/
@Import(name="autoMountHomeEfs")
private @Nullable Output autoMountHomeEfs;
/**
* @return Indicates whether auto-mounting of an EFS volume is supported for the user profile. The `DefaultAsDomain` value is only supported for user profiles. Do not use the `DefaultAsDomain` value when setting this parameter for a domain. Valid values are: `Enabled`, `Disabled`, and `DefaultAsDomain`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy