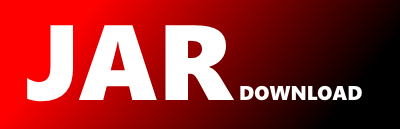
com.pulumi.aws.securityhub.Account Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.securityhub;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.securityhub.AccountArgs;
import com.pulumi.aws.securityhub.inputs.AccountState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Enables Security Hub for this AWS account.
*
* > **NOTE:** Destroying this resource will disable Security Hub for this AWS account.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.securityhub.Account;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Account("example");
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import an existing Security Hub enabled account using the AWS account ID. For example:
*
* ```sh
* $ pulumi import aws:securityhub/account:Account example 123456789012
* ```
*
*/
@ResourceType(type="aws:securityhub/account:Account")
public class Account extends com.pulumi.resources.CustomResource {
/**
* ARN of the SecurityHub Hub created in the account.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the SecurityHub Hub created in the account.
*
*/
public Output arn() {
return this.arn;
}
/**
* Whether to automatically enable new controls when they are added to standards that are enabled. By default, this is set to true, and new controls are enabled automatically. To not automatically enable new controls, set this to false.
*
*/
@Export(name="autoEnableControls", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoEnableControls;
/**
* @return Whether to automatically enable new controls when they are added to standards that are enabled. By default, this is set to true, and new controls are enabled automatically. To not automatically enable new controls, set this to false.
*
*/
public Output> autoEnableControls() {
return Codegen.optional(this.autoEnableControls);
}
/**
* Updates whether the calling account has consolidated control findings turned on. If the value for this field is set to `SECURITY_CONTROL`, Security Hub generates a single finding for a control check even when the check applies to multiple enabled standards. If the value for this field is set to `STANDARD_CONTROL`, Security Hub generates separate findings for a control check when the check applies to multiple enabled standards. For accounts that are part of an organization, this value can only be updated in the administrator account.
*
*/
@Export(name="controlFindingGenerator", refs={String.class}, tree="[0]")
private Output controlFindingGenerator;
/**
* @return Updates whether the calling account has consolidated control findings turned on. If the value for this field is set to `SECURITY_CONTROL`, Security Hub generates a single finding for a control check even when the check applies to multiple enabled standards. If the value for this field is set to `STANDARD_CONTROL`, Security Hub generates separate findings for a control check when the check applies to multiple enabled standards. For accounts that are part of an organization, this value can only be updated in the administrator account.
*
*/
public Output controlFindingGenerator() {
return this.controlFindingGenerator;
}
/**
* Whether to enable the security standards that Security Hub has designated as automatically enabled including: ` AWS Foundational Security Best Practices v1.0.0 ` and `CIS AWS Foundations Benchmark v1.2.0`. Defaults to `true`.
*
*/
@Export(name="enableDefaultStandards", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableDefaultStandards;
/**
* @return Whether to enable the security standards that Security Hub has designated as automatically enabled including: ` AWS Foundational Security Best Practices v1.0.0 ` and `CIS AWS Foundations Benchmark v1.2.0`. Defaults to `true`.
*
*/
public Output> enableDefaultStandards() {
return Codegen.optional(this.enableDefaultStandards);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Account(java.lang.String name) {
this(name, AccountArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Account(java.lang.String name, @Nullable AccountArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Account(java.lang.String name, @Nullable AccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:securityhub/account:Account", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Account(java.lang.String name, Output id, @Nullable AccountState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:securityhub/account:Account", name, state, makeResourceOptions(options, id), false);
}
private static AccountArgs makeArgs(@Nullable AccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccountArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Account get(java.lang.String name, Output id, @Nullable AccountState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Account(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy