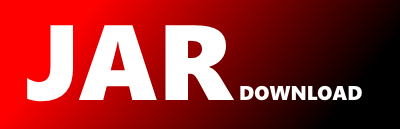
com.pulumi.aws.servicequotas.TemplateArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.servicequotas;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
public final class TemplateArgs extends com.pulumi.resources.ResourceArgs {
public static final TemplateArgs Empty = new TemplateArgs();
/**
* Quota identifier. To find the quota code for a specific quota, use the aws.servicequotas.ServiceQuota data source.
*
*/
@Import(name="quotaCode", required=true)
private Output quotaCode;
/**
* @return Quota identifier. To find the quota code for a specific quota, use the aws.servicequotas.ServiceQuota data source.
*
*/
public Output quotaCode() {
return this.quotaCode;
}
/**
* AWS Region to which the template applies.
*
*/
@Import(name="region", required=true)
private Output region;
/**
* @return AWS Region to which the template applies.
*
*/
public Output region() {
return this.region;
}
/**
* Service identifier. To find the service code value for an AWS service, use the aws.servicequotas.getService data source.
*
*/
@Import(name="serviceCode", required=true)
private Output serviceCode;
/**
* @return Service identifier. To find the service code value for an AWS service, use the aws.servicequotas.getService data source.
*
*/
public Output serviceCode() {
return this.serviceCode;
}
/**
* The new, increased value for the quota.
*
*/
@Import(name="value", required=true)
private Output value;
/**
* @return The new, increased value for the quota.
*
*/
public Output value() {
return this.value;
}
private TemplateArgs() {}
private TemplateArgs(TemplateArgs $) {
this.quotaCode = $.quotaCode;
this.region = $.region;
this.serviceCode = $.serviceCode;
this.value = $.value;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TemplateArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private TemplateArgs $;
public Builder() {
$ = new TemplateArgs();
}
public Builder(TemplateArgs defaults) {
$ = new TemplateArgs(Objects.requireNonNull(defaults));
}
/**
* @param quotaCode Quota identifier. To find the quota code for a specific quota, use the aws.servicequotas.ServiceQuota data source.
*
* @return builder
*
*/
public Builder quotaCode(Output quotaCode) {
$.quotaCode = quotaCode;
return this;
}
/**
* @param quotaCode Quota identifier. To find the quota code for a specific quota, use the aws.servicequotas.ServiceQuota data source.
*
* @return builder
*
*/
public Builder quotaCode(String quotaCode) {
return quotaCode(Output.of(quotaCode));
}
/**
* @param region AWS Region to which the template applies.
*
* @return builder
*
*/
public Builder region(Output region) {
$.region = region;
return this;
}
/**
* @param region AWS Region to which the template applies.
*
* @return builder
*
*/
public Builder region(String region) {
return region(Output.of(region));
}
/**
* @param serviceCode Service identifier. To find the service code value for an AWS service, use the aws.servicequotas.getService data source.
*
* @return builder
*
*/
public Builder serviceCode(Output serviceCode) {
$.serviceCode = serviceCode;
return this;
}
/**
* @param serviceCode Service identifier. To find the service code value for an AWS service, use the aws.servicequotas.getService data source.
*
* @return builder
*
*/
public Builder serviceCode(String serviceCode) {
return serviceCode(Output.of(serviceCode));
}
/**
* @param value The new, increased value for the quota.
*
* @return builder
*
*/
public Builder value(Output value) {
$.value = value;
return this;
}
/**
* @param value The new, increased value for the quota.
*
* @return builder
*
*/
public Builder value(Double value) {
return value(Output.of(value));
}
public TemplateArgs build() {
if ($.quotaCode == null) {
throw new MissingRequiredPropertyException("TemplateArgs", "quotaCode");
}
if ($.region == null) {
throw new MissingRequiredPropertyException("TemplateArgs", "region");
}
if ($.serviceCode == null) {
throw new MissingRequiredPropertyException("TemplateArgs", "serviceCode");
}
if ($.value == null) {
throw new MissingRequiredPropertyException("TemplateArgs", "value");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy