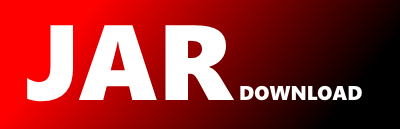
com.pulumi.aws.sesv2.outputs.ConfigurationSetEventDestinationEventDestination Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sesv2.outputs;
import com.pulumi.aws.sesv2.outputs.ConfigurationSetEventDestinationEventDestinationCloudWatchDestination;
import com.pulumi.aws.sesv2.outputs.ConfigurationSetEventDestinationEventDestinationEventBridgeDestination;
import com.pulumi.aws.sesv2.outputs.ConfigurationSetEventDestinationEventDestinationKinesisFirehoseDestination;
import com.pulumi.aws.sesv2.outputs.ConfigurationSetEventDestinationEventDestinationPinpointDestination;
import com.pulumi.aws.sesv2.outputs.ConfigurationSetEventDestinationEventDestinationSnsDestination;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ConfigurationSetEventDestinationEventDestination {
/**
* @return An object that defines an Amazon CloudWatch destination for email events. See `cloud_watch_destination` Block for details.
*
*/
private @Nullable ConfigurationSetEventDestinationEventDestinationCloudWatchDestination cloudWatchDestination;
/**
* @return When the event destination is enabled, the specified event types are sent to the destinations. Default: `false`.
*
*/
private @Nullable Boolean enabled;
private @Nullable ConfigurationSetEventDestinationEventDestinationEventBridgeDestination eventBridgeDestination;
/**
* @return An object that defines an Amazon Kinesis Data Firehose destination for email events. See `kinesis_firehose_destination` Block for details.
*
*/
private @Nullable ConfigurationSetEventDestinationEventDestinationKinesisFirehoseDestination kinesisFirehoseDestination;
/**
* @return An array that specifies which events the Amazon SES API v2 should send to the destinations. Valid values: `SEND`, `REJECT`, `BOUNCE`, `COMPLAINT`, `DELIVERY`, `OPEN`, `CLICK`, `RENDERING_FAILURE`, `DELIVERY_DELAY`, `SUBSCRIPTION`.
*
*/
private List matchingEventTypes;
/**
* @return An object that defines an Amazon Pinpoint project destination for email events. See `pinpoint_destination` Block for details.
*
*/
private @Nullable ConfigurationSetEventDestinationEventDestinationPinpointDestination pinpointDestination;
/**
* @return An object that defines an Amazon SNS destination for email events. See `sns_destination` Block for details.
*
*/
private @Nullable ConfigurationSetEventDestinationEventDestinationSnsDestination snsDestination;
private ConfigurationSetEventDestinationEventDestination() {}
/**
* @return An object that defines an Amazon CloudWatch destination for email events. See `cloud_watch_destination` Block for details.
*
*/
public Optional cloudWatchDestination() {
return Optional.ofNullable(this.cloudWatchDestination);
}
/**
* @return When the event destination is enabled, the specified event types are sent to the destinations. Default: `false`.
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
public Optional eventBridgeDestination() {
return Optional.ofNullable(this.eventBridgeDestination);
}
/**
* @return An object that defines an Amazon Kinesis Data Firehose destination for email events. See `kinesis_firehose_destination` Block for details.
*
*/
public Optional kinesisFirehoseDestination() {
return Optional.ofNullable(this.kinesisFirehoseDestination);
}
/**
* @return An array that specifies which events the Amazon SES API v2 should send to the destinations. Valid values: `SEND`, `REJECT`, `BOUNCE`, `COMPLAINT`, `DELIVERY`, `OPEN`, `CLICK`, `RENDERING_FAILURE`, `DELIVERY_DELAY`, `SUBSCRIPTION`.
*
*/
public List matchingEventTypes() {
return this.matchingEventTypes;
}
/**
* @return An object that defines an Amazon Pinpoint project destination for email events. See `pinpoint_destination` Block for details.
*
*/
public Optional pinpointDestination() {
return Optional.ofNullable(this.pinpointDestination);
}
/**
* @return An object that defines an Amazon SNS destination for email events. See `sns_destination` Block for details.
*
*/
public Optional snsDestination() {
return Optional.ofNullable(this.snsDestination);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ConfigurationSetEventDestinationEventDestination defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ConfigurationSetEventDestinationEventDestinationCloudWatchDestination cloudWatchDestination;
private @Nullable Boolean enabled;
private @Nullable ConfigurationSetEventDestinationEventDestinationEventBridgeDestination eventBridgeDestination;
private @Nullable ConfigurationSetEventDestinationEventDestinationKinesisFirehoseDestination kinesisFirehoseDestination;
private List matchingEventTypes;
private @Nullable ConfigurationSetEventDestinationEventDestinationPinpointDestination pinpointDestination;
private @Nullable ConfigurationSetEventDestinationEventDestinationSnsDestination snsDestination;
public Builder() {}
public Builder(ConfigurationSetEventDestinationEventDestination defaults) {
Objects.requireNonNull(defaults);
this.cloudWatchDestination = defaults.cloudWatchDestination;
this.enabled = defaults.enabled;
this.eventBridgeDestination = defaults.eventBridgeDestination;
this.kinesisFirehoseDestination = defaults.kinesisFirehoseDestination;
this.matchingEventTypes = defaults.matchingEventTypes;
this.pinpointDestination = defaults.pinpointDestination;
this.snsDestination = defaults.snsDestination;
}
@CustomType.Setter
public Builder cloudWatchDestination(@Nullable ConfigurationSetEventDestinationEventDestinationCloudWatchDestination cloudWatchDestination) {
this.cloudWatchDestination = cloudWatchDestination;
return this;
}
@CustomType.Setter
public Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder eventBridgeDestination(@Nullable ConfigurationSetEventDestinationEventDestinationEventBridgeDestination eventBridgeDestination) {
this.eventBridgeDestination = eventBridgeDestination;
return this;
}
@CustomType.Setter
public Builder kinesisFirehoseDestination(@Nullable ConfigurationSetEventDestinationEventDestinationKinesisFirehoseDestination kinesisFirehoseDestination) {
this.kinesisFirehoseDestination = kinesisFirehoseDestination;
return this;
}
@CustomType.Setter
public Builder matchingEventTypes(List matchingEventTypes) {
if (matchingEventTypes == null) {
throw new MissingRequiredPropertyException("ConfigurationSetEventDestinationEventDestination", "matchingEventTypes");
}
this.matchingEventTypes = matchingEventTypes;
return this;
}
public Builder matchingEventTypes(String... matchingEventTypes) {
return matchingEventTypes(List.of(matchingEventTypes));
}
@CustomType.Setter
public Builder pinpointDestination(@Nullable ConfigurationSetEventDestinationEventDestinationPinpointDestination pinpointDestination) {
this.pinpointDestination = pinpointDestination;
return this;
}
@CustomType.Setter
public Builder snsDestination(@Nullable ConfigurationSetEventDestinationEventDestinationSnsDestination snsDestination) {
this.snsDestination = snsDestination;
return this;
}
public ConfigurationSetEventDestinationEventDestination build() {
final var _resultValue = new ConfigurationSetEventDestinationEventDestination();
_resultValue.cloudWatchDestination = cloudWatchDestination;
_resultValue.enabled = enabled;
_resultValue.eventBridgeDestination = eventBridgeDestination;
_resultValue.kinesisFirehoseDestination = kinesisFirehoseDestination;
_resultValue.matchingEventTypes = matchingEventTypes;
_resultValue.pinpointDestination = pinpointDestination;
_resultValue.snsDestination = snsDestination;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy