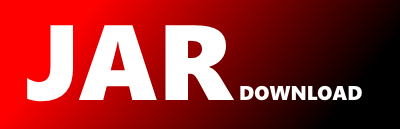
com.pulumi.aws.sns.Topic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sns;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.sns.TopicArgs;
import com.pulumi.aws.sns.inputs.TopicState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an SNS topic resource
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var userUpdates = new Topic("userUpdates", TopicArgs.builder()
* .name("user-updates-topic")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Example with Delivery Policy
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var userUpdates = new Topic("userUpdates", TopicArgs.builder()
* .name("user-updates-topic")
* .deliveryPolicy("""
* {
* "http": {
* "defaultHealthyRetryPolicy": {
* "minDelayTarget": 20,
* "maxDelayTarget": 20,
* "numRetries": 3,
* "numMaxDelayRetries": 0,
* "numNoDelayRetries": 0,
* "numMinDelayRetries": 0,
* "backoffFunction": "linear"
* },
* "disableSubscriptionOverrides": false,
* "defaultThrottlePolicy": {
* "maxReceivesPerSecond": 1
* }
* }
* }
* """)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Example with Server-side encryption (SSE)
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var userUpdates = new Topic("userUpdates", TopicArgs.builder()
* .name("user-updates-topic")
* .kmsMasterKeyId("alias/aws/sns")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Example with First-In-First-Out (FIFO)
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var userUpdates = new Topic("userUpdates", TopicArgs.builder()
* .name("user-updates-topic.fifo")
* .fifoTopic(true)
* .contentBasedDeduplication(true)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Message Delivery Status Arguments
*
* The `<endpoint>_success_feedback_role_arn` and `<endpoint>_failure_feedback_role_arn` arguments are used to give Amazon SNS write access to use CloudWatch Logs on your behalf. The `<endpoint>_success_feedback_sample_rate` argument is for specifying the sample rate percentage (0-100) of successfully delivered messages. After you configure the `<endpoint>_failure_feedback_role_arn` argument, then all failed message deliveries generate CloudWatch Logs.
*
* ## Import
*
* Using `pulumi import`, import SNS Topics using the topic `arn`. For example:
*
* ```sh
* $ pulumi import aws:sns/topic:Topic user_updates arn:aws:sns:us-west-2:123456789012:my-topic
* ```
*
*/
@ResourceType(type="aws:sns/topic:Topic")
public class Topic extends com.pulumi.resources.CustomResource {
/**
* IAM role for failure feedback
*
*/
@Export(name="applicationFailureFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> applicationFailureFeedbackRoleArn;
/**
* @return IAM role for failure feedback
*
*/
public Output> applicationFailureFeedbackRoleArn() {
return Codegen.optional(this.applicationFailureFeedbackRoleArn);
}
/**
* The IAM role permitted to receive success feedback for this topic
*
*/
@Export(name="applicationSuccessFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> applicationSuccessFeedbackRoleArn;
/**
* @return The IAM role permitted to receive success feedback for this topic
*
*/
public Output> applicationSuccessFeedbackRoleArn() {
return Codegen.optional(this.applicationSuccessFeedbackRoleArn);
}
/**
* Percentage of success to sample
*
*/
@Export(name="applicationSuccessFeedbackSampleRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> applicationSuccessFeedbackSampleRate;
/**
* @return Percentage of success to sample
*
*/
public Output> applicationSuccessFeedbackSampleRate() {
return Codegen.optional(this.applicationSuccessFeedbackSampleRate);
}
/**
* The message archive policy for FIFO topics. More details in the [AWS documentation](https://docs.aws.amazon.com/sns/latest/dg/message-archiving-and-replay-topic-owner.html).
*
*/
@Export(name="archivePolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> archivePolicy;
/**
* @return The message archive policy for FIFO topics. More details in the [AWS documentation](https://docs.aws.amazon.com/sns/latest/dg/message-archiving-and-replay-topic-owner.html).
*
*/
public Output> archivePolicy() {
return Codegen.optional(this.archivePolicy);
}
/**
* The ARN of the SNS topic, as a more obvious property (clone of id)
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the SNS topic, as a more obvious property (clone of id)
*
*/
public Output arn() {
return this.arn;
}
/**
* The oldest timestamp at which a FIFO topic subscriber can start a replay.
*
*/
@Export(name="beginningArchiveTime", refs={String.class}, tree="[0]")
private Output beginningArchiveTime;
/**
* @return The oldest timestamp at which a FIFO topic subscriber can start a replay.
*
*/
public Output beginningArchiveTime() {
return this.beginningArchiveTime;
}
/**
* Enables content-based deduplication for FIFO topics. For more information, see the [related documentation](https://docs.aws.amazon.com/sns/latest/dg/fifo-message-dedup.html)
*
*/
@Export(name="contentBasedDeduplication", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> contentBasedDeduplication;
/**
* @return Enables content-based deduplication for FIFO topics. For more information, see the [related documentation](https://docs.aws.amazon.com/sns/latest/dg/fifo-message-dedup.html)
*
*/
public Output> contentBasedDeduplication() {
return Codegen.optional(this.contentBasedDeduplication);
}
/**
* The SNS delivery policy. More details in the [AWS documentation](https://docs.aws.amazon.com/sns/latest/dg/DeliveryPolicies.html).
*
*/
@Export(name="deliveryPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> deliveryPolicy;
/**
* @return The SNS delivery policy. More details in the [AWS documentation](https://docs.aws.amazon.com/sns/latest/dg/DeliveryPolicies.html).
*
*/
public Output> deliveryPolicy() {
return Codegen.optional(this.deliveryPolicy);
}
/**
* The display name for the topic
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return The display name for the topic
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* Boolean indicating whether or not to create a FIFO (first-in-first-out) topic. FIFO topics can't deliver messages to customer managed endpoints, such as email addresses, mobile apps, SMS, or HTTP(S) endpoints. These endpoint types aren't guaranteed to preserve strict message ordering. Default is `false`.
*
*/
@Export(name="fifoTopic", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> fifoTopic;
/**
* @return Boolean indicating whether or not to create a FIFO (first-in-first-out) topic. FIFO topics can't deliver messages to customer managed endpoints, such as email addresses, mobile apps, SMS, or HTTP(S) endpoints. These endpoint types aren't guaranteed to preserve strict message ordering. Default is `false`.
*
*/
public Output> fifoTopic() {
return Codegen.optional(this.fifoTopic);
}
/**
* IAM role for failure feedback
*
*/
@Export(name="firehoseFailureFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> firehoseFailureFeedbackRoleArn;
/**
* @return IAM role for failure feedback
*
*/
public Output> firehoseFailureFeedbackRoleArn() {
return Codegen.optional(this.firehoseFailureFeedbackRoleArn);
}
/**
* The IAM role permitted to receive success feedback for this topic
*
*/
@Export(name="firehoseSuccessFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> firehoseSuccessFeedbackRoleArn;
/**
* @return The IAM role permitted to receive success feedback for this topic
*
*/
public Output> firehoseSuccessFeedbackRoleArn() {
return Codegen.optional(this.firehoseSuccessFeedbackRoleArn);
}
/**
* Percentage of success to sample
*
*/
@Export(name="firehoseSuccessFeedbackSampleRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> firehoseSuccessFeedbackSampleRate;
/**
* @return Percentage of success to sample
*
*/
public Output> firehoseSuccessFeedbackSampleRate() {
return Codegen.optional(this.firehoseSuccessFeedbackSampleRate);
}
/**
* IAM role for failure feedback
*
*/
@Export(name="httpFailureFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> httpFailureFeedbackRoleArn;
/**
* @return IAM role for failure feedback
*
*/
public Output> httpFailureFeedbackRoleArn() {
return Codegen.optional(this.httpFailureFeedbackRoleArn);
}
/**
* The IAM role permitted to receive success feedback for this topic
*
*/
@Export(name="httpSuccessFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> httpSuccessFeedbackRoleArn;
/**
* @return The IAM role permitted to receive success feedback for this topic
*
*/
public Output> httpSuccessFeedbackRoleArn() {
return Codegen.optional(this.httpSuccessFeedbackRoleArn);
}
/**
* Percentage of success to sample
*
*/
@Export(name="httpSuccessFeedbackSampleRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> httpSuccessFeedbackSampleRate;
/**
* @return Percentage of success to sample
*
*/
public Output> httpSuccessFeedbackSampleRate() {
return Codegen.optional(this.httpSuccessFeedbackSampleRate);
}
/**
* The ID of an AWS-managed customer master key (CMK) for Amazon SNS or a custom CMK. For more information, see [Key Terms](https://docs.aws.amazon.com/sns/latest/dg/sns-server-side-encryption.html#sse-key-terms)
*
*/
@Export(name="kmsMasterKeyId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kmsMasterKeyId;
/**
* @return The ID of an AWS-managed customer master key (CMK) for Amazon SNS or a custom CMK. For more information, see [Key Terms](https://docs.aws.amazon.com/sns/latest/dg/sns-server-side-encryption.html#sse-key-terms)
*
*/
public Output> kmsMasterKeyId() {
return Codegen.optional(this.kmsMasterKeyId);
}
/**
* IAM role for failure feedback
*
*/
@Export(name="lambdaFailureFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lambdaFailureFeedbackRoleArn;
/**
* @return IAM role for failure feedback
*
*/
public Output> lambdaFailureFeedbackRoleArn() {
return Codegen.optional(this.lambdaFailureFeedbackRoleArn);
}
/**
* The IAM role permitted to receive success feedback for this topic
*
*/
@Export(name="lambdaSuccessFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lambdaSuccessFeedbackRoleArn;
/**
* @return The IAM role permitted to receive success feedback for this topic
*
*/
public Output> lambdaSuccessFeedbackRoleArn() {
return Codegen.optional(this.lambdaSuccessFeedbackRoleArn);
}
/**
* Percentage of success to sample
*
*/
@Export(name="lambdaSuccessFeedbackSampleRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> lambdaSuccessFeedbackSampleRate;
/**
* @return Percentage of success to sample
*
*/
public Output> lambdaSuccessFeedbackSampleRate() {
return Codegen.optional(this.lambdaSuccessFeedbackSampleRate);
}
/**
* The name of the topic. Topic names must be made up of only uppercase and lowercase ASCII letters, numbers, underscores, and hyphens, and must be between 1 and 256 characters long. For a FIFO (first-in-first-out) topic, the name must end with the `.fifo` suffix. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the topic. Topic names must be made up of only uppercase and lowercase ASCII letters, numbers, underscores, and hyphens, and must be between 1 and 256 characters long. For a FIFO (first-in-first-out) topic, the name must end with the `.fifo` suffix. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`
*
*/
public Output name() {
return this.name;
}
/**
* Creates a unique name beginning with the specified prefix. Conflicts with `name`
*
*/
@Export(name="namePrefix", refs={String.class}, tree="[0]")
private Output namePrefix;
/**
* @return Creates a unique name beginning with the specified prefix. Conflicts with `name`
*
*/
public Output namePrefix() {
return this.namePrefix;
}
/**
* The AWS Account ID of the SNS topic owner
*
*/
@Export(name="owner", refs={String.class}, tree="[0]")
private Output owner;
/**
* @return The AWS Account ID of the SNS topic owner
*
*/
public Output owner() {
return this.owner;
}
/**
* The fully-formed AWS policy as JSON.
*
*/
@Export(name="policy", refs={String.class}, tree="[0]")
private Output policy;
/**
* @return The fully-formed AWS policy as JSON.
*
*/
public Output policy() {
return this.policy;
}
/**
* If `SignatureVersion` should be [1 (SHA1) or 2 (SHA256)](https://docs.aws.amazon.com/sns/latest/dg/sns-verify-signature-of-message.html). The signature version corresponds to the hashing algorithm used while creating the signature of the notifications, subscription confirmations, or unsubscribe confirmation messages sent by Amazon SNS.
*
*/
@Export(name="signatureVersion", refs={Integer.class}, tree="[0]")
private Output signatureVersion;
/**
* @return If `SignatureVersion` should be [1 (SHA1) or 2 (SHA256)](https://docs.aws.amazon.com/sns/latest/dg/sns-verify-signature-of-message.html). The signature version corresponds to the hashing algorithm used while creating the signature of the notifications, subscription confirmations, or unsubscribe confirmation messages sent by Amazon SNS.
*
*/
public Output signatureVersion() {
return this.signatureVersion;
}
/**
* IAM role for failure feedback
*
*/
@Export(name="sqsFailureFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sqsFailureFeedbackRoleArn;
/**
* @return IAM role for failure feedback
*
*/
public Output> sqsFailureFeedbackRoleArn() {
return Codegen.optional(this.sqsFailureFeedbackRoleArn);
}
/**
* The IAM role permitted to receive success feedback for this topic
*
*/
@Export(name="sqsSuccessFeedbackRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sqsSuccessFeedbackRoleArn;
/**
* @return The IAM role permitted to receive success feedback for this topic
*
*/
public Output> sqsSuccessFeedbackRoleArn() {
return Codegen.optional(this.sqsSuccessFeedbackRoleArn);
}
/**
* Percentage of success to sample
*
*/
@Export(name="sqsSuccessFeedbackSampleRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> sqsSuccessFeedbackSampleRate;
/**
* @return Percentage of success to sample
*
*/
public Output> sqsSuccessFeedbackSampleRate() {
return Codegen.optional(this.sqsSuccessFeedbackSampleRate);
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy