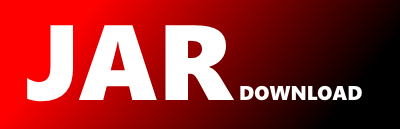
com.pulumi.aws.verifiedaccess.inputs.GroupState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.verifiedaccess.inputs;
import com.pulumi.aws.verifiedaccess.inputs.GroupSseConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GroupState extends com.pulumi.resources.ResourceArgs {
public static final GroupState Empty = new GroupState();
/**
* Timestamp when the access group was created.
*
*/
@Import(name="creationTime")
private @Nullable Output creationTime;
/**
* @return Timestamp when the access group was created.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy