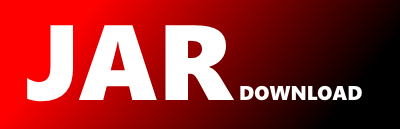
com.pulumi.aws.apigateway.ApiKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigateway;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.apigateway.ApiKeyArgs;
import com.pulumi.aws.apigateway.inputs.ApiKeyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an API Gateway API Key.
*
* > **NOTE:** Since the API Gateway usage plans feature was launched on August 11, 2016, usage plans are now **required** to associate an API key with an API stage.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigateway.ApiKey;
* import com.pulumi.aws.apigateway.ApiKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ApiKey("example", ApiKeyArgs.builder()
* .name("example")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import API Gateway Keys using the `id`. For example:
*
* ```sh
* $ pulumi import aws:apigateway/apiKey:ApiKey example 8bklk8bl1k3sB38D9B3l0enyWT8c09B30lkq0blk
* ```
*
*/
@ResourceType(type="aws:apigateway/apiKey:ApiKey")
public class ApiKey extends com.pulumi.resources.CustomResource {
/**
* ARN
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN
*
*/
public Output arn() {
return this.arn;
}
/**
* Creation date of the API key
*
*/
@Export(name="createdDate", refs={String.class}, tree="[0]")
private Output createdDate;
/**
* @return Creation date of the API key
*
*/
public Output createdDate() {
return this.createdDate;
}
/**
* An Amazon Web Services Marketplace customer identifier, when integrating with the Amazon Web Services SaaS Marketplace.
*
*/
@Export(name="customerId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customerId;
/**
* @return An Amazon Web Services Marketplace customer identifier, when integrating with the Amazon Web Services SaaS Marketplace.
*
*/
public Output> customerId() {
return Codegen.optional(this.customerId);
}
/**
* API key description. Defaults to "Managed by Pulumi".
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return API key description. Defaults to "Managed by Pulumi".
*
*/
public Output description() {
return this.description;
}
/**
* Whether the API key can be used by callers. Defaults to `true`.
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enabled;
/**
* @return Whether the API key can be used by callers. Defaults to `true`.
*
*/
public Output> enabled() {
return Codegen.optional(this.enabled);
}
/**
* Last update date of the API key
*
*/
@Export(name="lastUpdatedDate", refs={String.class}, tree="[0]")
private Output lastUpdatedDate;
/**
* @return Last update date of the API key
*
*/
public Output lastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
* Name of the API key.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the API key.
*
*/
public Output name() {
return this.name;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy