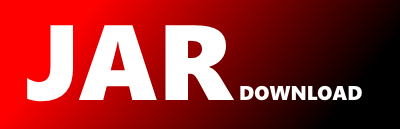
com.pulumi.aws.apigateway.Authorizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigateway;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.apigateway.AuthorizerArgs;
import com.pulumi.aws.apigateway.inputs.AuthorizerState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an API Gateway Authorizer.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigateway.RestApi;
* import com.pulumi.aws.apigateway.RestApiArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.lambda.Function;
* import com.pulumi.aws.lambda.FunctionArgs;
* import com.pulumi.aws.apigateway.Authorizer;
* import com.pulumi.aws.apigateway.AuthorizerArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import com.pulumi.asset.FileArchive;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var demoRestApi = new RestApi("demoRestApi", RestApiArgs.builder()
* .name("auth-demo")
* .build());
*
* final var invocationAssumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("apigateway.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var invocationRole = new Role("invocationRole", RoleArgs.builder()
* .name("api_gateway_auth_invocation")
* .path("/")
* .assumeRolePolicy(invocationAssumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* final var lambdaAssumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("sts:AssumeRole")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("lambda.amazonaws.com")
* .build())
* .build())
* .build());
*
* var lambda = new Role("lambda", RoleArgs.builder()
* .name("demo-lambda")
* .assumeRolePolicy(lambdaAssumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var authorizer = new Function("authorizer", FunctionArgs.builder()
* .code(new FileArchive("lambda-function.zip"))
* .name("api_gateway_authorizer")
* .role(lambda.arn())
* .handler("exports.example")
* .sourceCodeHash(StdFunctions.filebase64sha256(Filebase64sha256Args.builder()
* .input("lambda-function.zip")
* .build()).result())
* .build());
*
* var demo = new Authorizer("demo", AuthorizerArgs.builder()
* .name("demo")
* .restApi(demoRestApi.id())
* .authorizerUri(authorizer.invokeArn())
* .authorizerCredentials(invocationRole.arn())
* .build());
*
* final var invocationPolicy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("lambda:InvokeFunction")
* .resources(authorizer.arn())
* .build())
* .build());
*
* var invocationPolicyRolePolicy = new RolePolicy("invocationPolicyRolePolicy", RolePolicyArgs.builder()
* .name("default")
* .role(invocationRole.id())
* .policy(invocationPolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(invocationPolicy -> invocationPolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import AWS API Gateway Authorizer using the `REST-API-ID/AUTHORIZER-ID`. For example:
*
* ```sh
* $ pulumi import aws:apigateway/authorizer:Authorizer authorizer 12345abcde/example
* ```
*
*/
@ResourceType(type="aws:apigateway/authorizer:Authorizer")
public class Authorizer extends com.pulumi.resources.CustomResource {
/**
* ARN of the API Gateway Authorizer
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the API Gateway Authorizer
*
*/
public Output arn() {
return this.arn;
}
/**
* Credentials required for the authorizer. To specify an IAM Role for API Gateway to assume, use the IAM Role ARN.
*
*/
@Export(name="authorizerCredentials", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> authorizerCredentials;
/**
* @return Credentials required for the authorizer. To specify an IAM Role for API Gateway to assume, use the IAM Role ARN.
*
*/
public Output> authorizerCredentials() {
return Codegen.optional(this.authorizerCredentials);
}
/**
* TTL of cached authorizer results in seconds. Defaults to `300`.
*
*/
@Export(name="authorizerResultTtlInSeconds", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> authorizerResultTtlInSeconds;
/**
* @return TTL of cached authorizer results in seconds. Defaults to `300`.
*
*/
public Output> authorizerResultTtlInSeconds() {
return Codegen.optional(this.authorizerResultTtlInSeconds);
}
/**
* Authorizer's Uniform Resource Identifier (URI). This must be a well-formed Lambda function URI in the form of `arn:aws:apigateway:{region}:lambda:path/{service_api}`,
* e.g., `arn:aws:apigateway:us-west-2:lambda:path/2015-03-31/functions/arn:aws:lambda:us-west-2:012345678912:function:my-function/invocations`
*
*/
@Export(name="authorizerUri", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> authorizerUri;
/**
* @return Authorizer's Uniform Resource Identifier (URI). This must be a well-formed Lambda function URI in the form of `arn:aws:apigateway:{region}:lambda:path/{service_api}`,
* e.g., `arn:aws:apigateway:us-west-2:lambda:path/2015-03-31/functions/arn:aws:lambda:us-west-2:012345678912:function:my-function/invocations`
*
*/
public Output> authorizerUri() {
return Codegen.optional(this.authorizerUri);
}
/**
* Source of the identity in an incoming request. Defaults to `method.request.header.Authorization`. For `REQUEST` type, this may be a comma-separated list of values, including headers, query string parameters and stage variables - e.g., `"method.request.header.SomeHeaderName,method.request.querystring.SomeQueryStringName,stageVariables.SomeStageVariableName"`
*
*/
@Export(name="identitySource", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> identitySource;
/**
* @return Source of the identity in an incoming request. Defaults to `method.request.header.Authorization`. For `REQUEST` type, this may be a comma-separated list of values, including headers, query string parameters and stage variables - e.g., `"method.request.header.SomeHeaderName,method.request.querystring.SomeQueryStringName,stageVariables.SomeStageVariableName"`
*
*/
public Output> identitySource() {
return Codegen.optional(this.identitySource);
}
/**
* Validation expression for the incoming identity. For `TOKEN` type, this value should be a regular expression. The incoming token from the client is matched against this expression, and will proceed if the token matches. If the token doesn't match, the client receives a 401 Unauthorized response.
*
*/
@Export(name="identityValidationExpression", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> identityValidationExpression;
/**
* @return Validation expression for the incoming identity. For `TOKEN` type, this value should be a regular expression. The incoming token from the client is matched against this expression, and will proceed if the token matches. If the token doesn't match, the client receives a 401 Unauthorized response.
*
*/
public Output> identityValidationExpression() {
return Codegen.optional(this.identityValidationExpression);
}
/**
* Name of the authorizer
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the authorizer
*
*/
public Output name() {
return this.name;
}
/**
* List of the Amazon Cognito user pool ARNs. Each element is of this format: `arn:aws:cognito-idp:{region}:{account_id}:userpool/{user_pool_id}`.
*
*/
@Export(name="providerArns", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> providerArns;
/**
* @return List of the Amazon Cognito user pool ARNs. Each element is of this format: `arn:aws:cognito-idp:{region}:{account_id}:userpool/{user_pool_id}`.
*
*/
public Output>> providerArns() {
return Codegen.optional(this.providerArns);
}
/**
* ID of the associated REST API
*
*/
@Export(name="restApi", refs={String.class}, tree="[0]")
private Output restApi;
/**
* @return ID of the associated REST API
*
*/
public Output restApi() {
return this.restApi;
}
/**
* Type of the authorizer. Possible values are `TOKEN` for a Lambda function using a single authorization token submitted in a custom header, `REQUEST` for a Lambda function using incoming request parameters, or `COGNITO_USER_POOLS` for using an Amazon Cognito user pool. Defaults to `TOKEN`.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> type;
/**
* @return Type of the authorizer. Possible values are `TOKEN` for a Lambda function using a single authorization token submitted in a custom header, `REQUEST` for a Lambda function using incoming request parameters, or `COGNITO_USER_POOLS` for using an Amazon Cognito user pool. Defaults to `TOKEN`.
*
*/
public Output> type() {
return Codegen.optional(this.type);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Authorizer(java.lang.String name) {
this(name, AuthorizerArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Authorizer(java.lang.String name, AuthorizerArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Authorizer(java.lang.String name, AuthorizerArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:apigateway/authorizer:Authorizer", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Authorizer(java.lang.String name, Output id, @Nullable AuthorizerState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:apigateway/authorizer:Authorizer", name, state, makeResourceOptions(options, id), false);
}
private static AuthorizerArgs makeArgs(AuthorizerArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AuthorizerArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Authorizer get(java.lang.String name, Output id, @Nullable AuthorizerState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Authorizer(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy