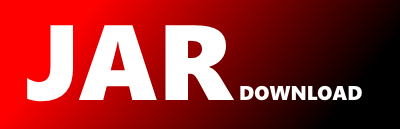
com.pulumi.aws.appstream.outputs.FleetComputeCapacity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appstream.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FleetComputeCapacity {
/**
* @return Number of currently available instances that can be used to stream sessions.
*
*/
private @Nullable Integer available;
/**
* @return Desired number of streaming instances.
*
*/
private @Nullable Integer desiredInstances;
/**
* @return Desired number of user sessions for a multi-session fleet. This is not allowed for single-session fleets.
*
*/
private @Nullable Integer desiredSessions;
/**
* @return Number of instances in use for streaming.
*
*/
private @Nullable Integer inUse;
/**
* @return Total number of simultaneous streaming instances that are running.
*
*/
private @Nullable Integer running;
private FleetComputeCapacity() {}
/**
* @return Number of currently available instances that can be used to stream sessions.
*
*/
public Optional available() {
return Optional.ofNullable(this.available);
}
/**
* @return Desired number of streaming instances.
*
*/
public Optional desiredInstances() {
return Optional.ofNullable(this.desiredInstances);
}
/**
* @return Desired number of user sessions for a multi-session fleet. This is not allowed for single-session fleets.
*
*/
public Optional desiredSessions() {
return Optional.ofNullable(this.desiredSessions);
}
/**
* @return Number of instances in use for streaming.
*
*/
public Optional inUse() {
return Optional.ofNullable(this.inUse);
}
/**
* @return Total number of simultaneous streaming instances that are running.
*
*/
public Optional running() {
return Optional.ofNullable(this.running);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FleetComputeCapacity defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer available;
private @Nullable Integer desiredInstances;
private @Nullable Integer desiredSessions;
private @Nullable Integer inUse;
private @Nullable Integer running;
public Builder() {}
public Builder(FleetComputeCapacity defaults) {
Objects.requireNonNull(defaults);
this.available = defaults.available;
this.desiredInstances = defaults.desiredInstances;
this.desiredSessions = defaults.desiredSessions;
this.inUse = defaults.inUse;
this.running = defaults.running;
}
@CustomType.Setter
public Builder available(@Nullable Integer available) {
this.available = available;
return this;
}
@CustomType.Setter
public Builder desiredInstances(@Nullable Integer desiredInstances) {
this.desiredInstances = desiredInstances;
return this;
}
@CustomType.Setter
public Builder desiredSessions(@Nullable Integer desiredSessions) {
this.desiredSessions = desiredSessions;
return this;
}
@CustomType.Setter
public Builder inUse(@Nullable Integer inUse) {
this.inUse = inUse;
return this;
}
@CustomType.Setter
public Builder running(@Nullable Integer running) {
this.running = running;
return this;
}
public FleetComputeCapacity build() {
final var _resultValue = new FleetComputeCapacity();
_resultValue.available = available;
_resultValue.desiredInstances = desiredInstances;
_resultValue.desiredSessions = desiredSessions;
_resultValue.inUse = inUse;
_resultValue.running = running;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy