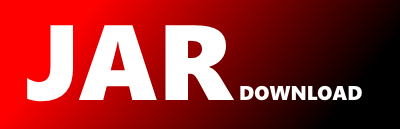
com.pulumi.aws.appsync.inputs.FunctionState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appsync.inputs;
import com.pulumi.aws.appsync.inputs.FunctionRuntimeArgs;
import com.pulumi.aws.appsync.inputs.FunctionSyncConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FunctionState extends com.pulumi.resources.ResourceArgs {
public static final FunctionState Empty = new FunctionState();
/**
* ID of the associated AppSync API.
*
*/
@Import(name="apiId")
private @Nullable Output apiId;
/**
* @return ID of the associated AppSync API.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy