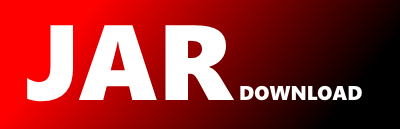
com.pulumi.aws.batch.ComputeEnvironmentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.batch;
import com.pulumi.aws.batch.inputs.ComputeEnvironmentComputeResourcesArgs;
import com.pulumi.aws.batch.inputs.ComputeEnvironmentEksConfigurationArgs;
import com.pulumi.aws.batch.inputs.ComputeEnvironmentUpdatePolicyArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ComputeEnvironmentArgs extends com.pulumi.resources.ResourceArgs {
public static final ComputeEnvironmentArgs Empty = new ComputeEnvironmentArgs();
/**
* The name for your compute environment. Up to 128 letters (uppercase and lowercase), numbers, and underscores are allowed. If omitted, the provider will assign a random, unique name.
*
*/
@Import(name="computeEnvironmentName")
private @Nullable Output computeEnvironmentName;
/**
* @return The name for your compute environment. Up to 128 letters (uppercase and lowercase), numbers, and underscores are allowed. If omitted, the provider will assign a random, unique name.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy