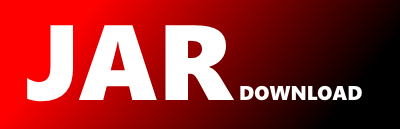
com.pulumi.aws.batch.JobQueueArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.batch;
import com.pulumi.aws.batch.inputs.JobQueueComputeEnvironmentOrderArgs;
import com.pulumi.aws.batch.inputs.JobQueueJobStateTimeLimitActionArgs;
import com.pulumi.aws.batch.inputs.JobQueueTimeoutsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class JobQueueArgs extends com.pulumi.resources.ResourceArgs {
public static final JobQueueArgs Empty = new JobQueueArgs();
/**
* The set of compute environments mapped to a job queue and their order relative to each other. The job scheduler uses this parameter to determine which compute environment runs a specific job. Compute environments must be in the VALID state before you can associate them with a job queue. You can associate up to three compute environments with a job queue.
*
*/
@Import(name="computeEnvironmentOrders")
private @Nullable Output> computeEnvironmentOrders;
/**
* @return The set of compute environments mapped to a job queue and their order relative to each other. The job scheduler uses this parameter to determine which compute environment runs a specific job. Compute environments must be in the VALID state before you can associate them with a job queue. You can associate up to three compute environments with a job queue.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy