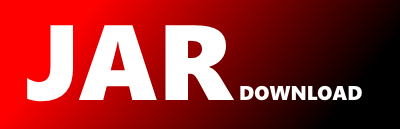
com.pulumi.aws.cloudformation.StackInstancesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudformation;
import com.pulumi.aws.cloudformation.inputs.StackInstancesDeploymentTargetsArgs;
import com.pulumi.aws.cloudformation.inputs.StackInstancesOperationPreferencesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StackInstancesArgs extends com.pulumi.resources.ResourceArgs {
public static final StackInstancesArgs Empty = new StackInstancesArgs();
/**
* Accounts where you want to create stack instances in the specified `regions`. You can specify either `accounts` or `deployment_targets`, but not both.
*
*/
@Import(name="accounts")
private @Nullable Output> accounts;
/**
* @return Accounts where you want to create stack instances in the specified `regions`. You can specify either `accounts` or `deployment_targets`, but not both.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy