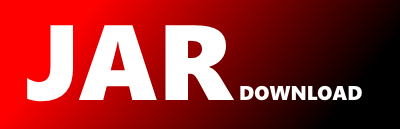
com.pulumi.aws.cloudfront.outputs.CachePolicyParametersInCacheKeyAndForwardedToOrigin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudfront.outputs;
import com.pulumi.aws.cloudfront.outputs.CachePolicyParametersInCacheKeyAndForwardedToOriginCookiesConfig;
import com.pulumi.aws.cloudfront.outputs.CachePolicyParametersInCacheKeyAndForwardedToOriginHeadersConfig;
import com.pulumi.aws.cloudfront.outputs.CachePolicyParametersInCacheKeyAndForwardedToOriginQueryStringsConfig;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CachePolicyParametersInCacheKeyAndForwardedToOrigin {
/**
* @return Whether any cookies in viewer requests are included in the cache key and automatically included in requests that CloudFront sends to the origin. See Cookies Config for more information.
*
*/
private CachePolicyParametersInCacheKeyAndForwardedToOriginCookiesConfig cookiesConfig;
/**
* @return Flag determines whether the Accept-Encoding HTTP header is included in the cache key and in requests that CloudFront sends to the origin.
*
*/
private @Nullable Boolean enableAcceptEncodingBrotli;
/**
* @return Whether the Accept-Encoding HTTP header is included in the cache key and in requests sent to the origin by CloudFront.
*
*/
private @Nullable Boolean enableAcceptEncodingGzip;
/**
* @return Whether any HTTP headers are included in the cache key and automatically included in requests that CloudFront sends to the origin. See Headers Config for more information.
*
*/
private CachePolicyParametersInCacheKeyAndForwardedToOriginHeadersConfig headersConfig;
/**
* @return Whether any URL query strings in viewer requests are included in the cache key. It also automatically includes these query strings in requests that CloudFront sends to the origin. Please refer to the Query String Config for more information.
*
*/
private CachePolicyParametersInCacheKeyAndForwardedToOriginQueryStringsConfig queryStringsConfig;
private CachePolicyParametersInCacheKeyAndForwardedToOrigin() {}
/**
* @return Whether any cookies in viewer requests are included in the cache key and automatically included in requests that CloudFront sends to the origin. See Cookies Config for more information.
*
*/
public CachePolicyParametersInCacheKeyAndForwardedToOriginCookiesConfig cookiesConfig() {
return this.cookiesConfig;
}
/**
* @return Flag determines whether the Accept-Encoding HTTP header is included in the cache key and in requests that CloudFront sends to the origin.
*
*/
public Optional enableAcceptEncodingBrotli() {
return Optional.ofNullable(this.enableAcceptEncodingBrotli);
}
/**
* @return Whether the Accept-Encoding HTTP header is included in the cache key and in requests sent to the origin by CloudFront.
*
*/
public Optional enableAcceptEncodingGzip() {
return Optional.ofNullable(this.enableAcceptEncodingGzip);
}
/**
* @return Whether any HTTP headers are included in the cache key and automatically included in requests that CloudFront sends to the origin. See Headers Config for more information.
*
*/
public CachePolicyParametersInCacheKeyAndForwardedToOriginHeadersConfig headersConfig() {
return this.headersConfig;
}
/**
* @return Whether any URL query strings in viewer requests are included in the cache key. It also automatically includes these query strings in requests that CloudFront sends to the origin. Please refer to the Query String Config for more information.
*
*/
public CachePolicyParametersInCacheKeyAndForwardedToOriginQueryStringsConfig queryStringsConfig() {
return this.queryStringsConfig;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CachePolicyParametersInCacheKeyAndForwardedToOrigin defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private CachePolicyParametersInCacheKeyAndForwardedToOriginCookiesConfig cookiesConfig;
private @Nullable Boolean enableAcceptEncodingBrotli;
private @Nullable Boolean enableAcceptEncodingGzip;
private CachePolicyParametersInCacheKeyAndForwardedToOriginHeadersConfig headersConfig;
private CachePolicyParametersInCacheKeyAndForwardedToOriginQueryStringsConfig queryStringsConfig;
public Builder() {}
public Builder(CachePolicyParametersInCacheKeyAndForwardedToOrigin defaults) {
Objects.requireNonNull(defaults);
this.cookiesConfig = defaults.cookiesConfig;
this.enableAcceptEncodingBrotli = defaults.enableAcceptEncodingBrotli;
this.enableAcceptEncodingGzip = defaults.enableAcceptEncodingGzip;
this.headersConfig = defaults.headersConfig;
this.queryStringsConfig = defaults.queryStringsConfig;
}
@CustomType.Setter
public Builder cookiesConfig(CachePolicyParametersInCacheKeyAndForwardedToOriginCookiesConfig cookiesConfig) {
if (cookiesConfig == null) {
throw new MissingRequiredPropertyException("CachePolicyParametersInCacheKeyAndForwardedToOrigin", "cookiesConfig");
}
this.cookiesConfig = cookiesConfig;
return this;
}
@CustomType.Setter
public Builder enableAcceptEncodingBrotli(@Nullable Boolean enableAcceptEncodingBrotli) {
this.enableAcceptEncodingBrotli = enableAcceptEncodingBrotli;
return this;
}
@CustomType.Setter
public Builder enableAcceptEncodingGzip(@Nullable Boolean enableAcceptEncodingGzip) {
this.enableAcceptEncodingGzip = enableAcceptEncodingGzip;
return this;
}
@CustomType.Setter
public Builder headersConfig(CachePolicyParametersInCacheKeyAndForwardedToOriginHeadersConfig headersConfig) {
if (headersConfig == null) {
throw new MissingRequiredPropertyException("CachePolicyParametersInCacheKeyAndForwardedToOrigin", "headersConfig");
}
this.headersConfig = headersConfig;
return this;
}
@CustomType.Setter
public Builder queryStringsConfig(CachePolicyParametersInCacheKeyAndForwardedToOriginQueryStringsConfig queryStringsConfig) {
if (queryStringsConfig == null) {
throw new MissingRequiredPropertyException("CachePolicyParametersInCacheKeyAndForwardedToOrigin", "queryStringsConfig");
}
this.queryStringsConfig = queryStringsConfig;
return this;
}
public CachePolicyParametersInCacheKeyAndForwardedToOrigin build() {
final var _resultValue = new CachePolicyParametersInCacheKeyAndForwardedToOrigin();
_resultValue.cookiesConfig = cookiesConfig;
_resultValue.enableAcceptEncodingBrotli = enableAcceptEncodingBrotli;
_resultValue.enableAcceptEncodingGzip = enableAcceptEncodingGzip;
_resultValue.headersConfig = headersConfig;
_resultValue.queryStringsConfig = queryStringsConfig;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy