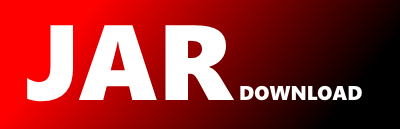
com.pulumi.aws.cloudwatch.MetricAlarm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudwatch;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
import com.pulumi.aws.cloudwatch.inputs.MetricAlarmState;
import com.pulumi.aws.cloudwatch.outputs.MetricAlarmMetricQuery;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CloudWatch Metric Alarm resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.MetricAlarm;
* import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foobar = new MetricAlarm("foobar", MetricAlarmArgs.builder()
* .name("test-foobar5")
* .comparisonOperator("GreaterThanOrEqualToThreshold")
* .evaluationPeriods(2)
* .metricName("CPUUtilization")
* .namespace("AWS/EC2")
* .period(120)
* .statistic("Average")
* .threshold(80)
* .alarmDescription("This metric monitors ec2 cpu utilization")
* .insufficientDataActions()
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Example in Conjunction with Scaling Policies
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.autoscaling.Policy;
* import com.pulumi.aws.autoscaling.PolicyArgs;
* import com.pulumi.aws.cloudwatch.MetricAlarm;
* import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var bat = new Policy("bat", PolicyArgs.builder()
* .name("foobar3-test")
* .scalingAdjustment(4)
* .adjustmentType("ChangeInCapacity")
* .cooldown(300)
* .autoscalingGroupName(bar.name())
* .build());
*
* var batMetricAlarm = new MetricAlarm("batMetricAlarm", MetricAlarmArgs.builder()
* .name("test-foobar5")
* .comparisonOperator("GreaterThanOrEqualToThreshold")
* .evaluationPeriods(2)
* .metricName("CPUUtilization")
* .namespace("AWS/EC2")
* .period(120)
* .statistic("Average")
* .threshold(80)
* .dimensions(Map.of("AutoScalingGroupName", bar.name()))
* .alarmDescription("This metric monitors ec2 cpu utilization")
* .alarmActions(bat.arn())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Example with an Expression
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.MetricAlarm;
* import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
* import com.pulumi.aws.cloudwatch.inputs.MetricAlarmMetricQueryArgs;
* import com.pulumi.aws.cloudwatch.inputs.MetricAlarmMetricQueryMetricArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foobar = new MetricAlarm("foobar", MetricAlarmArgs.builder()
* .name("test-foobar")
* .comparisonOperator("GreaterThanOrEqualToThreshold")
* .evaluationPeriods(2)
* .threshold(10)
* .alarmDescription("Request error rate has exceeded 10%")
* .insufficientDataActions()
* .metricQueries(
* MetricAlarmMetricQueryArgs.builder()
* .id("e1")
* .expression("m2/m1*100")
* .label("Error Rate")
* .returnData("true")
* .build(),
* MetricAlarmMetricQueryArgs.builder()
* .id("m1")
* .metric(MetricAlarmMetricQueryMetricArgs.builder()
* .metricName("RequestCount")
* .namespace("AWS/ApplicationELB")
* .period(120)
* .stat("Sum")
* .unit("Count")
* .dimensions(Map.of("LoadBalancer", "app/web"))
* .build())
* .build(),
* MetricAlarmMetricQueryArgs.builder()
* .id("m2")
* .metric(MetricAlarmMetricQueryMetricArgs.builder()
* .metricName("HTTPCode_ELB_5XX_Count")
* .namespace("AWS/ApplicationELB")
* .period(120)
* .stat("Sum")
* .unit("Count")
* .dimensions(Map.of("LoadBalancer", "app/web"))
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.MetricAlarm;
* import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
* import com.pulumi.aws.cloudwatch.inputs.MetricAlarmMetricQueryArgs;
* import com.pulumi.aws.cloudwatch.inputs.MetricAlarmMetricQueryMetricArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var xxAnomalyDetection = new MetricAlarm("xxAnomalyDetection", MetricAlarmArgs.builder()
* .name("test-foobar")
* .comparisonOperator("GreaterThanUpperThreshold")
* .evaluationPeriods(2)
* .thresholdMetricId("e1")
* .alarmDescription("This metric monitors ec2 cpu utilization")
* .insufficientDataActions()
* .metricQueries(
* MetricAlarmMetricQueryArgs.builder()
* .id("e1")
* .expression("ANOMALY_DETECTION_BAND(m1)")
* .label("CPUUtilization (Expected)")
* .returnData("true")
* .build(),
* MetricAlarmMetricQueryArgs.builder()
* .id("m1")
* .returnData("true")
* .metric(MetricAlarmMetricQueryMetricArgs.builder()
* .metricName("CPUUtilization")
* .namespace("AWS/EC2")
* .period(120)
* .stat("Average")
* .unit("Count")
* .dimensions(Map.of("InstanceId", "i-abc123"))
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Example of monitoring Healthy Hosts on NLB using Target Group and NLB
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.MetricAlarm;
* import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var nlbHealthyhosts = new MetricAlarm("nlbHealthyhosts", MetricAlarmArgs.builder()
* .name("alarmname")
* .comparisonOperator("LessThanThreshold")
* .evaluationPeriods(1)
* .metricName("HealthyHostCount")
* .namespace("AWS/NetworkELB")
* .period(60)
* .statistic("Average")
* .threshold(logstashServersCount)
* .alarmDescription("Number of healthy nodes in Target Group")
* .actionsEnabled("true")
* .alarmActions(sns.arn())
* .okActions(sns.arn())
* .dimensions(Map.ofEntries(
* Map.entry("TargetGroup", lb_tg.arnSuffix()),
* Map.entry("LoadBalancer", lb.arnSuffix())
* ))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* > **NOTE:** You cannot create a metric alarm consisting of both `statistic` and `extended_statistic` parameters.
* You must choose one or the other
*
* ## Import
*
* Using `pulumi import`, import CloudWatch Metric Alarm using the `alarm_name`. For example:
*
* ```sh
* $ pulumi import aws:cloudwatch/metricAlarm:MetricAlarm test alarm-12345
* ```
*
*/
@ResourceType(type="aws:cloudwatch/metricAlarm:MetricAlarm")
public class MetricAlarm extends com.pulumi.resources.CustomResource {
/**
* Indicates whether or not actions should be executed during any changes to the alarm's state. Defaults to `true`.
*
*/
@Export(name="actionsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> actionsEnabled;
/**
* @return Indicates whether or not actions should be executed during any changes to the alarm's state. Defaults to `true`.
*
*/
public Output> actionsEnabled() {
return Codegen.optional(this.actionsEnabled);
}
/**
* The list of actions to execute when this alarm transitions into an ALARM state from any other state. Each action is specified as an Amazon Resource Name (ARN).
*
*/
@Export(name="alarmActions", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> alarmActions;
/**
* @return The list of actions to execute when this alarm transitions into an ALARM state from any other state. Each action is specified as an Amazon Resource Name (ARN).
*
*/
public Output>> alarmActions() {
return Codegen.optional(this.alarmActions);
}
/**
* The description for the alarm.
*
*/
@Export(name="alarmDescription", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> alarmDescription;
/**
* @return The description for the alarm.
*
*/
public Output> alarmDescription() {
return Codegen.optional(this.alarmDescription);
}
/**
* The ARN of the CloudWatch Metric Alarm.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the CloudWatch Metric Alarm.
*
*/
public Output arn() {
return this.arn;
}
/**
* The arithmetic operation to use when comparing the specified Statistic and Threshold. The specified Statistic value is used as the first operand. Either of the following is supported: `GreaterThanOrEqualToThreshold`, `GreaterThanThreshold`, `LessThanThreshold`, `LessThanOrEqualToThreshold`. Additionally, the values `LessThanLowerOrGreaterThanUpperThreshold`, `LessThanLowerThreshold`, and `GreaterThanUpperThreshold` are used only for alarms based on anomaly detection models.
*
*/
@Export(name="comparisonOperator", refs={String.class}, tree="[0]")
private Output comparisonOperator;
/**
* @return The arithmetic operation to use when comparing the specified Statistic and Threshold. The specified Statistic value is used as the first operand. Either of the following is supported: `GreaterThanOrEqualToThreshold`, `GreaterThanThreshold`, `LessThanThreshold`, `LessThanOrEqualToThreshold`. Additionally, the values `LessThanLowerOrGreaterThanUpperThreshold`, `LessThanLowerThreshold`, and `GreaterThanUpperThreshold` are used only for alarms based on anomaly detection models.
*
*/
public Output comparisonOperator() {
return this.comparisonOperator;
}
/**
* The number of datapoints that must be breaching to trigger the alarm.
*
*/
@Export(name="datapointsToAlarm", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> datapointsToAlarm;
/**
* @return The number of datapoints that must be breaching to trigger the alarm.
*
*/
public Output> datapointsToAlarm() {
return Codegen.optional(this.datapointsToAlarm);
}
/**
* The dimensions for the alarm's associated metric. For the list of available dimensions see the AWS documentation [here](http://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/CW_Support_For_AWS.html).
*
*/
@Export(name="dimensions", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> dimensions;
/**
* @return The dimensions for the alarm's associated metric. For the list of available dimensions see the AWS documentation [here](http://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/CW_Support_For_AWS.html).
*
*/
public Output>> dimensions() {
return Codegen.optional(this.dimensions);
}
/**
* Used only for alarms based on percentiles.
* If you specify `ignore`, the alarm state will not change during periods with too few data points to be statistically significant.
* If you specify `evaluate` or omit this parameter, the alarm will always be evaluated and possibly change state no matter how many data points are available.
* The following values are supported: `ignore`, and `evaluate`.
*
*/
@Export(name="evaluateLowSampleCountPercentiles", refs={String.class}, tree="[0]")
private Output evaluateLowSampleCountPercentiles;
/**
* @return Used only for alarms based on percentiles.
* If you specify `ignore`, the alarm state will not change during periods with too few data points to be statistically significant.
* If you specify `evaluate` or omit this parameter, the alarm will always be evaluated and possibly change state no matter how many data points are available.
* The following values are supported: `ignore`, and `evaluate`.
*
*/
public Output evaluateLowSampleCountPercentiles() {
return this.evaluateLowSampleCountPercentiles;
}
/**
* The number of periods over which data is compared to the specified threshold.
*
*/
@Export(name="evaluationPeriods", refs={Integer.class}, tree="[0]")
private Output evaluationPeriods;
/**
* @return The number of periods over which data is compared to the specified threshold.
*
*/
public Output evaluationPeriods() {
return this.evaluationPeriods;
}
/**
* The percentile statistic for the metric associated with the alarm. Specify a value between p0.0 and p100.
*
*/
@Export(name="extendedStatistic", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> extendedStatistic;
/**
* @return The percentile statistic for the metric associated with the alarm. Specify a value between p0.0 and p100.
*
*/
public Output> extendedStatistic() {
return Codegen.optional(this.extendedStatistic);
}
/**
* The list of actions to execute when this alarm transitions into an INSUFFICIENT_DATA state from any other state. Each action is specified as an Amazon Resource Name (ARN).
*
*/
@Export(name="insufficientDataActions", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> insufficientDataActions;
/**
* @return The list of actions to execute when this alarm transitions into an INSUFFICIENT_DATA state from any other state. Each action is specified as an Amazon Resource Name (ARN).
*
*/
public Output>> insufficientDataActions() {
return Codegen.optional(this.insufficientDataActions);
}
/**
* The name for the alarm's associated metric.
* See docs for [supported metrics](https://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/CW_Support_For_AWS.html).
*
*/
@Export(name="metricName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> metricName;
/**
* @return The name for the alarm's associated metric.
* See docs for [supported metrics](https://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/CW_Support_For_AWS.html).
*
*/
public Output> metricName() {
return Codegen.optional(this.metricName);
}
/**
* Enables you to create an alarm based on a metric math expression. You may specify at most 20.
*
*/
@Export(name="metricQueries", refs={List.class,MetricAlarmMetricQuery.class}, tree="[0,1]")
private Output* @Nullable */ List> metricQueries;
/**
* @return Enables you to create an alarm based on a metric math expression. You may specify at most 20.
*
*/
public Output>> metricQueries() {
return Codegen.optional(this.metricQueries);
}
/**
* The descriptive name for the alarm. This name must be unique within the user's AWS account
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The descriptive name for the alarm. This name must be unique within the user's AWS account
*
*/
public Output name() {
return this.name;
}
/**
* The namespace for the alarm's associated metric. See docs for the [list of namespaces](https://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/aws-namespaces.html).
* See docs for [supported metrics](https://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/CW_Support_For_AWS.html).
*
*/
@Export(name="namespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namespace;
/**
* @return The namespace for the alarm's associated metric. See docs for the [list of namespaces](https://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/aws-namespaces.html).
* See docs for [supported metrics](https://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/CW_Support_For_AWS.html).
*
*/
public Output> namespace() {
return Codegen.optional(this.namespace);
}
/**
* The list of actions to execute when this alarm transitions into an OK state from any other state. Each action is specified as an Amazon Resource Name (ARN).
*
*/
@Export(name="okActions", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> okActions;
/**
* @return The list of actions to execute when this alarm transitions into an OK state from any other state. Each action is specified as an Amazon Resource Name (ARN).
*
*/
public Output>> okActions() {
return Codegen.optional(this.okActions);
}
/**
* The period in seconds over which the specified `statistic` is applied.
* Valid values are `10`, `30`, or any multiple of `60`.
*
*/
@Export(name="period", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> period;
/**
* @return The period in seconds over which the specified `statistic` is applied.
* Valid values are `10`, `30`, or any multiple of `60`.
*
*/
public Output> period() {
return Codegen.optional(this.period);
}
/**
* The statistic to apply to the alarm's associated metric.
* Either of the following is supported: `SampleCount`, `Average`, `Sum`, `Minimum`, `Maximum`
*
*/
@Export(name="statistic", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> statistic;
/**
* @return The statistic to apply to the alarm's associated metric.
* Either of the following is supported: `SampleCount`, `Average`, `Sum`, `Minimum`, `Maximum`
*
*/
public Output> statistic() {
return Codegen.optional(this.statistic);
}
/**
* A map of tags to assign to the resource. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
* > **NOTE:** If you specify at least one `metric_query`, you may not specify a `metric_name`, `namespace`, `period` or `statistic`. If you do not specify a `metric_query`, you must specify each of these (although you may use `extended_statistic` instead of `statistic`).
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
* > **NOTE:** If you specify at least one `metric_query`, you may not specify a `metric_name`, `namespace`, `period` or `statistic`. If you do not specify a `metric_query`, you must specify each of these (although you may use `extended_statistic` instead of `statistic`).
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy