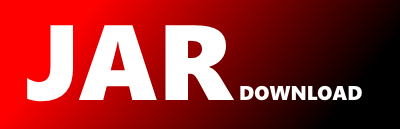
com.pulumi.aws.codebuild.Project Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.codebuild;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.codebuild.ProjectArgs;
import com.pulumi.aws.codebuild.inputs.ProjectState;
import com.pulumi.aws.codebuild.outputs.ProjectArtifacts;
import com.pulumi.aws.codebuild.outputs.ProjectBuildBatchConfig;
import com.pulumi.aws.codebuild.outputs.ProjectCache;
import com.pulumi.aws.codebuild.outputs.ProjectEnvironment;
import com.pulumi.aws.codebuild.outputs.ProjectFileSystemLocation;
import com.pulumi.aws.codebuild.outputs.ProjectLogsConfig;
import com.pulumi.aws.codebuild.outputs.ProjectSecondaryArtifact;
import com.pulumi.aws.codebuild.outputs.ProjectSecondarySource;
import com.pulumi.aws.codebuild.outputs.ProjectSecondarySourceVersion;
import com.pulumi.aws.codebuild.outputs.ProjectSource;
import com.pulumi.aws.codebuild.outputs.ProjectVpcConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CodeBuild Project resource. See also the `aws.codebuild.Webhook` resource, which manages the webhook to the source (e.g., the "rebuild every time a code change is pushed" option in the CodeBuild web console).
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import com.pulumi.aws.codebuild.Project;
* import com.pulumi.aws.codebuild.ProjectArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectArtifactsArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectCacheArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectEnvironmentArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectLogsConfigArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectLogsConfigCloudwatchLogsArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectLogsConfigS3LogsArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectSourceArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectSourceGitSubmodulesConfigArgs;
* import com.pulumi.aws.codebuild.inputs.ProjectVpcConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleBucketV2 = new BucketV2("exampleBucketV2", BucketV2Args.builder()
* .bucket("example")
* .build());
*
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(exampleBucketV2.id())
* .acl("private")
* .build());
*
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("codebuild.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .name("example")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "logs:CreateLogGroup",
* "logs:CreateLogStream",
* "logs:PutLogEvents")
* .resources("*")
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "ec2:CreateNetworkInterface",
* "ec2:DescribeDhcpOptions",
* "ec2:DescribeNetworkInterfaces",
* "ec2:DeleteNetworkInterface",
* "ec2:DescribeSubnets",
* "ec2:DescribeSecurityGroups",
* "ec2:DescribeVpcs")
* .resources("*")
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("ec2:CreateNetworkInterfacePermission")
* .resources("arn:aws:ec2:us-east-1:123456789012:network-interface/*")
* .conditions(
* GetPolicyDocumentStatementConditionArgs.builder()
* .test("StringEquals")
* .variable("ec2:Subnet")
* .values(
* example1.arn(),
* example2.arn())
* .build(),
* GetPolicyDocumentStatementConditionArgs.builder()
* .test("StringEquals")
* .variable("ec2:AuthorizedService")
* .values("codebuild.amazonaws.com")
* .build())
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("s3:*")
* .resources(
* exampleBucketV2.arn(),
* exampleBucketV2.arn().applyValue(arn -> String.format("%s/*", arn)))
* .build())
* .build());
*
* var exampleRolePolicy = new RolePolicy("exampleRolePolicy", RolePolicyArgs.builder()
* .role(exampleRole.name())
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(example -> example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
*
* var exampleProject = new Project("exampleProject", ProjectArgs.builder()
* .name("test-project")
* .description("test_codebuild_project")
* .buildTimeout(5)
* .serviceRole(exampleRole.arn())
* .artifacts(ProjectArtifactsArgs.builder()
* .type("NO_ARTIFACTS")
* .build())
* .cache(ProjectCacheArgs.builder()
* .type("S3")
* .location(exampleBucketV2.bucket())
* .build())
* .environment(ProjectEnvironmentArgs.builder()
* .computeType("BUILD_GENERAL1_SMALL")
* .image("aws/codebuild/amazonlinux2-x86_64-standard:4.0")
* .type("LINUX_CONTAINER")
* .imagePullCredentialsType("CODEBUILD")
* .environmentVariables(
* ProjectEnvironmentEnvironmentVariableArgs.builder()
* .name("SOME_KEY1")
* .value("SOME_VALUE1")
* .build(),
* ProjectEnvironmentEnvironmentVariableArgs.builder()
* .name("SOME_KEY2")
* .value("SOME_VALUE2")
* .type("PARAMETER_STORE")
* .build())
* .build())
* .logsConfig(ProjectLogsConfigArgs.builder()
* .cloudwatchLogs(ProjectLogsConfigCloudwatchLogsArgs.builder()
* .groupName("log-group")
* .streamName("log-stream")
* .build())
* .s3Logs(ProjectLogsConfigS3LogsArgs.builder()
* .status("ENABLED")
* .location(exampleBucketV2.id().applyValue(id -> String.format("%s/build-log", id)))
* .build())
* .build())
* .source(ProjectSourceArgs.builder()
* .type("GITHUB")
* .location("https://github.com/mitchellh/packer.git")
* .gitCloneDepth(1)
* .gitSubmodulesConfig(ProjectSourceGitSubmodulesConfigArgs.builder()
* .fetchSubmodules(true)
* .build())
* .build())
* .sourceVersion("master")
* .vpcConfig(ProjectVpcConfigArgs.builder()
* .vpcId(exampleAwsVpc.id())
* .subnets(
* example1.id(),
* example2.id())
* .securityGroupIds(
* example1AwsSecurityGroup.id(),
* example2AwsSecurityGroup.id())
* .build())
* .tags(Map.of("Environment", "Test"))
* .build());
*
* var project_with_cache = new Project("project-with-cache", ProjectArgs.builder()
* .name("test-project-cache")
* .description("test_codebuild_project_cache")
* .buildTimeout(5)
* .queuedTimeout(5)
* .serviceRole(exampleRole.arn())
* .artifacts(ProjectArtifactsArgs.builder()
* .type("NO_ARTIFACTS")
* .build())
* .cache(ProjectCacheArgs.builder()
* .type("LOCAL")
* .modes(
* "LOCAL_DOCKER_LAYER_CACHE",
* "LOCAL_SOURCE_CACHE")
* .build())
* .environment(ProjectEnvironmentArgs.builder()
* .computeType("BUILD_GENERAL1_SMALL")
* .image("aws/codebuild/amazonlinux2-x86_64-standard:4.0")
* .type("LINUX_CONTAINER")
* .imagePullCredentialsType("CODEBUILD")
* .environmentVariables(ProjectEnvironmentEnvironmentVariableArgs.builder()
* .name("SOME_KEY1")
* .value("SOME_VALUE1")
* .build())
* .build())
* .source(ProjectSourceArgs.builder()
* .type("GITHUB")
* .location("https://github.com/mitchellh/packer.git")
* .gitCloneDepth(1)
* .build())
* .tags(Map.of("Environment", "Test"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import CodeBuild Project using the `name`. For example:
*
* ```sh
* $ pulumi import aws:codebuild/project:Project name project-name
* ```
*
*/
@ResourceType(type="aws:codebuild/project:Project")
public class Project extends com.pulumi.resources.CustomResource {
/**
* ARN of the CodeBuild project.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the CodeBuild project.
*
*/
public Output arn() {
return this.arn;
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="artifacts", refs={ProjectArtifacts.class}, tree="[0]")
private Output artifacts;
/**
* @return Configuration block. Detailed below.
*
*/
public Output artifacts() {
return this.artifacts;
}
/**
* Generates a publicly-accessible URL for the projects build badge. Available as `badge_url` attribute when enabled.
*
*/
@Export(name="badgeEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> badgeEnabled;
/**
* @return Generates a publicly-accessible URL for the projects build badge. Available as `badge_url` attribute when enabled.
*
*/
public Output> badgeEnabled() {
return Codegen.optional(this.badgeEnabled);
}
/**
* URL of the build badge when `badge_enabled` is enabled.
*
*/
@Export(name="badgeUrl", refs={String.class}, tree="[0]")
private Output badgeUrl;
/**
* @return URL of the build badge when `badge_enabled` is enabled.
*
*/
public Output badgeUrl() {
return this.badgeUrl;
}
/**
* Defines the batch build options for the project.
*
*/
@Export(name="buildBatchConfig", refs={ProjectBuildBatchConfig.class}, tree="[0]")
private Output* @Nullable */ ProjectBuildBatchConfig> buildBatchConfig;
/**
* @return Defines the batch build options for the project.
*
*/
public Output> buildBatchConfig() {
return Codegen.optional(this.buildBatchConfig);
}
/**
* Number of minutes, from 5 to 2160 (36 hours), for AWS CodeBuild to wait until timing out any related build that does not get marked as completed. The default is 60 minutes. The `build_timeout` property is not available on the `Lambda` compute type.
*
*/
@Export(name="buildTimeout", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> buildTimeout;
/**
* @return Number of minutes, from 5 to 2160 (36 hours), for AWS CodeBuild to wait until timing out any related build that does not get marked as completed. The default is 60 minutes. The `build_timeout` property is not available on the `Lambda` compute type.
*
*/
public Output> buildTimeout() {
return Codegen.optional(this.buildTimeout);
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="cache", refs={ProjectCache.class}, tree="[0]")
private Output* @Nullable */ ProjectCache> cache;
/**
* @return Configuration block. Detailed below.
*
*/
public Output> cache() {
return Codegen.optional(this.cache);
}
/**
* Specify a maximum number of concurrent builds for the project. The value specified must be greater than 0 and less than the account concurrent running builds limit.
*
*/
@Export(name="concurrentBuildLimit", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> concurrentBuildLimit;
/**
* @return Specify a maximum number of concurrent builds for the project. The value specified must be greater than 0 and less than the account concurrent running builds limit.
*
*/
public Output> concurrentBuildLimit() {
return Codegen.optional(this.concurrentBuildLimit);
}
/**
* Short description of the project.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return Short description of the project.
*
*/
public Output description() {
return this.description;
}
/**
* AWS Key Management Service (AWS KMS) customer master key (CMK) to be used for encrypting the build project's build output artifacts.
*
*/
@Export(name="encryptionKey", refs={String.class}, tree="[0]")
private Output encryptionKey;
/**
* @return AWS Key Management Service (AWS KMS) customer master key (CMK) to be used for encrypting the build project's build output artifacts.
*
*/
public Output encryptionKey() {
return this.encryptionKey;
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="environment", refs={ProjectEnvironment.class}, tree="[0]")
private Output environment;
/**
* @return Configuration block. Detailed below.
*
*/
public Output environment() {
return this.environment;
}
/**
* A set of file system locations to mount inside the build. File system locations are documented below.
*
*/
@Export(name="fileSystemLocations", refs={List.class,ProjectFileSystemLocation.class}, tree="[0,1]")
private Output* @Nullable */ List> fileSystemLocations;
/**
* @return A set of file system locations to mount inside the build. File system locations are documented below.
*
*/
public Output>> fileSystemLocations() {
return Codegen.optional(this.fileSystemLocations);
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="logsConfig", refs={ProjectLogsConfig.class}, tree="[0]")
private Output* @Nullable */ ProjectLogsConfig> logsConfig;
/**
* @return Configuration block. Detailed below.
*
*/
public Output> logsConfig() {
return Codegen.optional(this.logsConfig);
}
/**
* Project's name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Project's name.
*
*/
public Output name() {
return this.name;
}
/**
* Specifies the visibility of the project's builds. Possible values are: `PUBLIC_READ` and `PRIVATE`. Default value is `PRIVATE`.
*
*/
@Export(name="projectVisibility", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> projectVisibility;
/**
* @return Specifies the visibility of the project's builds. Possible values are: `PUBLIC_READ` and `PRIVATE`. Default value is `PRIVATE`.
*
*/
public Output> projectVisibility() {
return Codegen.optional(this.projectVisibility);
}
/**
* The project identifier used with the public build APIs.
*
*/
@Export(name="publicProjectAlias", refs={String.class}, tree="[0]")
private Output publicProjectAlias;
/**
* @return The project identifier used with the public build APIs.
*
*/
public Output publicProjectAlias() {
return this.publicProjectAlias;
}
/**
* Number of minutes, from 5 to 480 (8 hours), a build is allowed to be queued before it times out. The default is 8 hours. The `queued_timeout` property is not available on the `Lambda` compute type.
*
*/
@Export(name="queuedTimeout", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> queuedTimeout;
/**
* @return Number of minutes, from 5 to 480 (8 hours), a build is allowed to be queued before it times out. The default is 8 hours. The `queued_timeout` property is not available on the `Lambda` compute type.
*
*/
public Output> queuedTimeout() {
return Codegen.optional(this.queuedTimeout);
}
/**
* The ARN of the IAM role that enables CodeBuild to access the CloudWatch Logs and Amazon S3 artifacts for the project's builds in order to display them publicly. Only applicable if `project_visibility` is `PUBLIC_READ`.
*
*/
@Export(name="resourceAccessRole", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> resourceAccessRole;
/**
* @return The ARN of the IAM role that enables CodeBuild to access the CloudWatch Logs and Amazon S3 artifacts for the project's builds in order to display them publicly. Only applicable if `project_visibility` is `PUBLIC_READ`.
*
*/
public Output> resourceAccessRole() {
return Codegen.optional(this.resourceAccessRole);
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="secondaryArtifacts", refs={List.class,ProjectSecondaryArtifact.class}, tree="[0,1]")
private Output* @Nullable */ List> secondaryArtifacts;
/**
* @return Configuration block. Detailed below.
*
*/
public Output>> secondaryArtifacts() {
return Codegen.optional(this.secondaryArtifacts);
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="secondarySourceVersions", refs={List.class,ProjectSecondarySourceVersion.class}, tree="[0,1]")
private Output* @Nullable */ List> secondarySourceVersions;
/**
* @return Configuration block. Detailed below.
*
*/
public Output>> secondarySourceVersions() {
return Codegen.optional(this.secondarySourceVersions);
}
/**
* Configuration block. Detailed below.
*
*/
@Export(name="secondarySources", refs={List.class,ProjectSecondarySource.class}, tree="[0,1]")
private Output* @Nullable */ List> secondarySources;
/**
* @return Configuration block. Detailed below.
*
*/
public Output>> secondarySources() {
return Codegen.optional(this.secondarySources);
}
/**
* Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to interact with dependent AWS services on behalf of the AWS account.
*
*/
@Export(name="serviceRole", refs={String.class}, tree="[0]")
private Output serviceRole;
/**
* @return Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to interact with dependent AWS services on behalf of the AWS account.
*
*/
public Output serviceRole() {
return this.serviceRole;
}
/**
* Configuration block. Detailed below.
*
* The following arguments are optional:
*
*/
@Export(name="source", refs={ProjectSource.class}, tree="[0]")
private Output source;
/**
* @return Configuration block. Detailed below.
*
* The following arguments are optional:
*
*/
public Output source() {
return this.source;
}
/**
* Version of the build input to be built for this project. If not specified, the latest version is used.
*
*/
@Export(name="sourceVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sourceVersion;
/**
* @return Version of the build input to be built for this project. If not specified, the latest version is used.
*
*/
public Output> sourceVersion() {
return Codegen.optional(this.sourceVersion);
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy