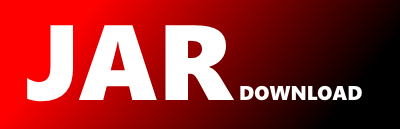
com.pulumi.aws.codedeploy.DeploymentGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.codedeploy;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.codedeploy.DeploymentGroupArgs;
import com.pulumi.aws.codedeploy.inputs.DeploymentGroupState;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupAlarmConfiguration;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupAutoRollbackConfiguration;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupBlueGreenDeploymentConfig;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupDeploymentStyle;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupEc2TagFilter;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupEc2TagSet;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupEcsService;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupLoadBalancerInfo;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupOnPremisesInstanceTagFilter;
import com.pulumi.aws.codedeploy.outputs.DeploymentGroupTriggerConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CodeDeploy Deployment Group for a CodeDeploy Application
*
* > **NOTE on blue/green deployments:** When using `green_fleet_provisioning_option` with the `COPY_AUTO_SCALING_GROUP` action, CodeDeploy will create a new ASG with a different name. This ASG is _not_ managed by this provider and will conflict with existing configuration and state. You may want to use a different approach to managing deployments that involve multiple ASG, such as `DISCOVER_EXISTING` with separate blue and green ASG.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.codedeploy.Application;
* import com.pulumi.aws.codedeploy.ApplicationArgs;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import com.pulumi.aws.codedeploy.DeploymentGroup;
* import com.pulumi.aws.codedeploy.DeploymentGroupArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupEc2TagSetArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupTriggerConfigurationArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupAutoRollbackConfigurationArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupAlarmConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("codedeploy.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var example = new Role("example", RoleArgs.builder()
* .name("example-role")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var aWSCodeDeployRole = new RolePolicyAttachment("aWSCodeDeployRole", RolePolicyAttachmentArgs.builder()
* .policyArn("arn:aws:iam::aws:policy/service-role/AWSCodeDeployRole")
* .role(example.name())
* .build());
*
* var exampleApplication = new Application("exampleApplication", ApplicationArgs.builder()
* .name("example-app")
* .build());
*
* var exampleTopic = new Topic("exampleTopic", TopicArgs.builder()
* .name("example-topic")
* .build());
*
* var exampleDeploymentGroup = new DeploymentGroup("exampleDeploymentGroup", DeploymentGroupArgs.builder()
* .appName(exampleApplication.name())
* .deploymentGroupName("example-group")
* .serviceRoleArn(example.arn())
* .ec2TagSets(DeploymentGroupEc2TagSetArgs.builder()
* .ec2TagFilters(
* DeploymentGroupEc2TagSetEc2TagFilterArgs.builder()
* .key("filterkey1")
* .type("KEY_AND_VALUE")
* .value("filtervalue")
* .build(),
* DeploymentGroupEc2TagSetEc2TagFilterArgs.builder()
* .key("filterkey2")
* .type("KEY_AND_VALUE")
* .value("filtervalue")
* .build())
* .build())
* .triggerConfigurations(DeploymentGroupTriggerConfigurationArgs.builder()
* .triggerEvents("DeploymentFailure")
* .triggerName("example-trigger")
* .triggerTargetArn(exampleTopic.arn())
* .build())
* .autoRollbackConfiguration(DeploymentGroupAutoRollbackConfigurationArgs.builder()
* .enabled(true)
* .events("DEPLOYMENT_FAILURE")
* .build())
* .alarmConfiguration(DeploymentGroupAlarmConfigurationArgs.builder()
* .alarms("my-alarm-name")
* .enabled(true)
* .build())
* .outdatedInstancesStrategy("UPDATE")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Blue Green Deployments with ECS
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.codedeploy.Application;
* import com.pulumi.aws.codedeploy.ApplicationArgs;
* import com.pulumi.aws.codedeploy.DeploymentGroup;
* import com.pulumi.aws.codedeploy.DeploymentGroupArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupAutoRollbackConfigurationArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigDeploymentReadyOptionArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigTerminateBlueInstancesOnDeploymentSuccessArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupDeploymentStyleArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupEcsServiceArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoTargetGroupPairInfoArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoTargetGroupPairInfoProdTrafficRouteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Application("example", ApplicationArgs.builder()
* .computePlatform("ECS")
* .name("example")
* .build());
*
* var exampleDeploymentGroup = new DeploymentGroup("exampleDeploymentGroup", DeploymentGroupArgs.builder()
* .appName(example.name())
* .deploymentConfigName("CodeDeployDefault.ECSAllAtOnce")
* .deploymentGroupName("example")
* .serviceRoleArn(exampleAwsIamRole.arn())
* .autoRollbackConfiguration(DeploymentGroupAutoRollbackConfigurationArgs.builder()
* .enabled(true)
* .events("DEPLOYMENT_FAILURE")
* .build())
* .blueGreenDeploymentConfig(DeploymentGroupBlueGreenDeploymentConfigArgs.builder()
* .deploymentReadyOption(DeploymentGroupBlueGreenDeploymentConfigDeploymentReadyOptionArgs.builder()
* .actionOnTimeout("CONTINUE_DEPLOYMENT")
* .build())
* .terminateBlueInstancesOnDeploymentSuccess(DeploymentGroupBlueGreenDeploymentConfigTerminateBlueInstancesOnDeploymentSuccessArgs.builder()
* .action("TERMINATE")
* .terminationWaitTimeInMinutes(5)
* .build())
* .build())
* .deploymentStyle(DeploymentGroupDeploymentStyleArgs.builder()
* .deploymentOption("WITH_TRAFFIC_CONTROL")
* .deploymentType("BLUE_GREEN")
* .build())
* .ecsService(DeploymentGroupEcsServiceArgs.builder()
* .clusterName(exampleAwsEcsCluster.name())
* .serviceName(exampleAwsEcsService.name())
* .build())
* .loadBalancerInfo(DeploymentGroupLoadBalancerInfoArgs.builder()
* .targetGroupPairInfo(DeploymentGroupLoadBalancerInfoTargetGroupPairInfoArgs.builder()
* .prodTrafficRoute(DeploymentGroupLoadBalancerInfoTargetGroupPairInfoProdTrafficRouteArgs.builder()
* .listenerArns(exampleAwsLbListener.arn())
* .build())
* .targetGroups(
* DeploymentGroupLoadBalancerInfoTargetGroupPairInfoTargetGroupArgs.builder()
* .name(blue.name())
* .build(),
* DeploymentGroupLoadBalancerInfoTargetGroupPairInfoTargetGroupArgs.builder()
* .name(green.name())
* .build())
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Blue Green Deployments with Servers and Classic ELB
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.codedeploy.Application;
* import com.pulumi.aws.codedeploy.ApplicationArgs;
* import com.pulumi.aws.codedeploy.DeploymentGroup;
* import com.pulumi.aws.codedeploy.DeploymentGroupArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupDeploymentStyleArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigDeploymentReadyOptionArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigGreenFleetProvisioningOptionArgs;
* import com.pulumi.aws.codedeploy.inputs.DeploymentGroupBlueGreenDeploymentConfigTerminateBlueInstancesOnDeploymentSuccessArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Application("example", ApplicationArgs.builder()
* .name("example-app")
* .build());
*
* var exampleDeploymentGroup = new DeploymentGroup("exampleDeploymentGroup", DeploymentGroupArgs.builder()
* .appName(example.name())
* .deploymentGroupName("example-group")
* .serviceRoleArn(exampleAwsIamRole.arn())
* .deploymentStyle(DeploymentGroupDeploymentStyleArgs.builder()
* .deploymentOption("WITH_TRAFFIC_CONTROL")
* .deploymentType("BLUE_GREEN")
* .build())
* .loadBalancerInfo(DeploymentGroupLoadBalancerInfoArgs.builder()
* .elbInfos(DeploymentGroupLoadBalancerInfoElbInfoArgs.builder()
* .name(exampleAwsElb.name())
* .build())
* .build())
* .blueGreenDeploymentConfig(DeploymentGroupBlueGreenDeploymentConfigArgs.builder()
* .deploymentReadyOption(DeploymentGroupBlueGreenDeploymentConfigDeploymentReadyOptionArgs.builder()
* .actionOnTimeout("STOP_DEPLOYMENT")
* .waitTimeInMinutes(60)
* .build())
* .greenFleetProvisioningOption(DeploymentGroupBlueGreenDeploymentConfigGreenFleetProvisioningOptionArgs.builder()
* .action("DISCOVER_EXISTING")
* .build())
* .terminateBlueInstancesOnDeploymentSuccess(DeploymentGroupBlueGreenDeploymentConfigTerminateBlueInstancesOnDeploymentSuccessArgs.builder()
* .action("KEEP_ALIVE")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import CodeDeploy Deployment Groups using `app_name`, a colon, and `deployment_group_name`. For example:
*
* ```sh
* $ pulumi import aws:codedeploy/deploymentGroup:DeploymentGroup example my-application:my-deployment-group
* ```
*
*/
@ResourceType(type="aws:codedeploy/deploymentGroup:DeploymentGroup")
public class DeploymentGroup extends com.pulumi.resources.CustomResource {
/**
* Configuration block of alarms associated with the deployment group (documented below).
*
*/
@Export(name="alarmConfiguration", refs={DeploymentGroupAlarmConfiguration.class}, tree="[0]")
private Output* @Nullable */ DeploymentGroupAlarmConfiguration> alarmConfiguration;
/**
* @return Configuration block of alarms associated with the deployment group (documented below).
*
*/
public Output> alarmConfiguration() {
return Codegen.optional(this.alarmConfiguration);
}
/**
* The name of the application.
*
*/
@Export(name="appName", refs={String.class}, tree="[0]")
private Output appName;
/**
* @return The name of the application.
*
*/
public Output appName() {
return this.appName;
}
/**
* The ARN of the CodeDeploy deployment group.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the CodeDeploy deployment group.
*
*/
public Output arn() {
return this.arn;
}
/**
* Configuration block of the automatic rollback configuration associated with the deployment group (documented below).
*
*/
@Export(name="autoRollbackConfiguration", refs={DeploymentGroupAutoRollbackConfiguration.class}, tree="[0]")
private Output* @Nullable */ DeploymentGroupAutoRollbackConfiguration> autoRollbackConfiguration;
/**
* @return Configuration block of the automatic rollback configuration associated with the deployment group (documented below).
*
*/
public Output> autoRollbackConfiguration() {
return Codegen.optional(this.autoRollbackConfiguration);
}
/**
* Autoscaling groups associated with the deployment group.
*
*/
@Export(name="autoscalingGroups", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> autoscalingGroups;
/**
* @return Autoscaling groups associated with the deployment group.
*
*/
public Output>> autoscalingGroups() {
return Codegen.optional(this.autoscalingGroups);
}
/**
* Configuration block of the blue/green deployment options for a deployment group (documented below).
*
*/
@Export(name="blueGreenDeploymentConfig", refs={DeploymentGroupBlueGreenDeploymentConfig.class}, tree="[0]")
private Output blueGreenDeploymentConfig;
/**
* @return Configuration block of the blue/green deployment options for a deployment group (documented below).
*
*/
public Output blueGreenDeploymentConfig() {
return this.blueGreenDeploymentConfig;
}
/**
* The destination platform type for the deployment.
*
*/
@Export(name="computePlatform", refs={String.class}, tree="[0]")
private Output computePlatform;
/**
* @return The destination platform type for the deployment.
*
*/
public Output computePlatform() {
return this.computePlatform;
}
/**
* The name of the group's deployment config. The default is "CodeDeployDefault.OneAtATime".
*
*/
@Export(name="deploymentConfigName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> deploymentConfigName;
/**
* @return The name of the group's deployment config. The default is "CodeDeployDefault.OneAtATime".
*
*/
public Output> deploymentConfigName() {
return Codegen.optional(this.deploymentConfigName);
}
/**
* The ID of the CodeDeploy deployment group.
*
*/
@Export(name="deploymentGroupId", refs={String.class}, tree="[0]")
private Output deploymentGroupId;
/**
* @return The ID of the CodeDeploy deployment group.
*
*/
public Output deploymentGroupId() {
return this.deploymentGroupId;
}
/**
* The name of the deployment group.
*
*/
@Export(name="deploymentGroupName", refs={String.class}, tree="[0]")
private Output deploymentGroupName;
/**
* @return The name of the deployment group.
*
*/
public Output deploymentGroupName() {
return this.deploymentGroupName;
}
/**
* Configuration block of the type of deployment, either in-place or blue/green, you want to run and whether to route deployment traffic behind a load balancer (documented below).
*
*/
@Export(name="deploymentStyle", refs={DeploymentGroupDeploymentStyle.class}, tree="[0]")
private Output* @Nullable */ DeploymentGroupDeploymentStyle> deploymentStyle;
/**
* @return Configuration block of the type of deployment, either in-place or blue/green, you want to run and whether to route deployment traffic behind a load balancer (documented below).
*
*/
public Output> deploymentStyle() {
return Codegen.optional(this.deploymentStyle);
}
/**
* Tag filters associated with the deployment group. See the AWS docs for details.
*
*/
@Export(name="ec2TagFilters", refs={List.class,DeploymentGroupEc2TagFilter.class}, tree="[0,1]")
private Output* @Nullable */ List> ec2TagFilters;
/**
* @return Tag filters associated with the deployment group. See the AWS docs for details.
*
*/
public Output>> ec2TagFilters() {
return Codegen.optional(this.ec2TagFilters);
}
/**
* Configuration block(s) of Tag filters associated with the deployment group, which are also referred to as tag groups (documented below). See the AWS docs for details.
*
*/
@Export(name="ec2TagSets", refs={List.class,DeploymentGroupEc2TagSet.class}, tree="[0,1]")
private Output* @Nullable */ List> ec2TagSets;
/**
* @return Configuration block(s) of Tag filters associated with the deployment group, which are also referred to as tag groups (documented below). See the AWS docs for details.
*
*/
public Output>> ec2TagSets() {
return Codegen.optional(this.ec2TagSets);
}
/**
* Configuration block(s) of the ECS services for a deployment group (documented below).
*
*/
@Export(name="ecsService", refs={DeploymentGroupEcsService.class}, tree="[0]")
private Output* @Nullable */ DeploymentGroupEcsService> ecsService;
/**
* @return Configuration block(s) of the ECS services for a deployment group (documented below).
*
*/
public Output> ecsService() {
return Codegen.optional(this.ecsService);
}
/**
* Single configuration block of the load balancer to use in a blue/green deployment (documented below).
*
*/
@Export(name="loadBalancerInfo", refs={DeploymentGroupLoadBalancerInfo.class}, tree="[0]")
private Output* @Nullable */ DeploymentGroupLoadBalancerInfo> loadBalancerInfo;
/**
* @return Single configuration block of the load balancer to use in a blue/green deployment (documented below).
*
*/
public Output> loadBalancerInfo() {
return Codegen.optional(this.loadBalancerInfo);
}
/**
* On premise tag filters associated with the group. See the AWS docs for details.
*
*/
@Export(name="onPremisesInstanceTagFilters", refs={List.class,DeploymentGroupOnPremisesInstanceTagFilter.class}, tree="[0,1]")
private Output* @Nullable */ List> onPremisesInstanceTagFilters;
/**
* @return On premise tag filters associated with the group. See the AWS docs for details.
*
*/
public Output>> onPremisesInstanceTagFilters() {
return Codegen.optional(this.onPremisesInstanceTagFilters);
}
/**
* Configuration block of Indicates what happens when new Amazon EC2 instances are launched mid-deployment and do not receive the deployed application revision. Valid values are `UPDATE` and `IGNORE`. Defaults to `UPDATE`.
*
*/
@Export(name="outdatedInstancesStrategy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> outdatedInstancesStrategy;
/**
* @return Configuration block of Indicates what happens when new Amazon EC2 instances are launched mid-deployment and do not receive the deployed application revision. Valid values are `UPDATE` and `IGNORE`. Defaults to `UPDATE`.
*
*/
public Output> outdatedInstancesStrategy() {
return Codegen.optional(this.outdatedInstancesStrategy);
}
/**
* The service role ARN that allows deployments.
*
*/
@Export(name="serviceRoleArn", refs={String.class}, tree="[0]")
private Output serviceRoleArn;
/**
* @return The service role ARN that allows deployments.
*
*/
public Output serviceRoleArn() {
return this.serviceRoleArn;
}
/**
* Key-value map of resource tags. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy