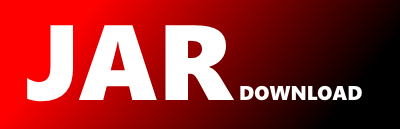
com.pulumi.aws.ec2.EipAssociationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EipAssociationArgs extends com.pulumi.resources.ResourceArgs {
public static final EipAssociationArgs Empty = new EipAssociationArgs();
/**
* The allocation ID. This is required for EC2-VPC.
*
*/
@Import(name="allocationId")
private @Nullable Output allocationId;
/**
* @return The allocation ID. This is required for EC2-VPC.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy