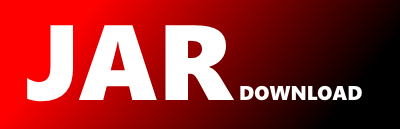
com.pulumi.aws.ec2.RouteTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.RouteTableArgs;
import com.pulumi.aws.ec2.inputs.RouteTableState;
import com.pulumi.aws.ec2.outputs.RouteTableRoute;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a resource to create a VPC routing table.
*
* > **NOTE on Route Tables and Routes:** This provider currently
* provides both a standalone Route resource and a Route Table resource with routes
* defined in-line. At this time you cannot use a Route Table with in-line routes
* in conjunction with any Route resources. Doing so will cause
* a conflict of rule settings and will overwrite rules.
*
* > **NOTE on `gateway_id` and `nat_gateway_id`:** The AWS API is very forgiving with these two
* attributes and the `aws.ec2.RouteTable` resource can be created with a NAT ID specified as a Gateway ID attribute.
* This _will_ lead to a permanent diff between your configuration and statefile, as the API returns the correct
* parameters in the returned route table. If you're experiencing constant diffs in your `aws.ec2.RouteTable` resources,
* the first thing to check is whether or not you're specifying a NAT ID instead of a Gateway ID, or vice-versa.
*
* > **NOTE on `propagating_vgws` and the `aws.ec2.VpnGatewayRoutePropagation` resource:**
* If the `propagating_vgws` argument is present, it's not supported to _also_
* define route propagations using `aws.ec2.VpnGatewayRoutePropagation`, since
* this resource will delete any propagating gateways not explicitly listed in
* `propagating_vgws`. Omit this argument when defining route propagation using
* the separate resource.
*
* ## Example Usage
*
* ### Basic example
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.RouteTable;
* import com.pulumi.aws.ec2.RouteTableArgs;
* import com.pulumi.aws.ec2.inputs.RouteTableRouteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new RouteTable("example", RouteTableArgs.builder()
* .vpcId(exampleAwsVpc.id())
* .routes(
* RouteTableRouteArgs.builder()
* .cidrBlock("10.0.1.0/24")
* .gatewayId(exampleAwsInternetGateway.id())
* .build(),
* RouteTableRouteArgs.builder()
* .ipv6CidrBlock("::/0")
* .egressOnlyGatewayId(exampleAwsEgressOnlyInternetGateway.id())
* .build())
* .tags(Map.of("Name", "example"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* To subsequently remove all managed routes:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.RouteTable;
* import com.pulumi.aws.ec2.RouteTableArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new RouteTable("example", RouteTableArgs.builder()
* .vpcId(exampleAwsVpc.id())
* .routes()
* .tags(Map.of("Name", "example"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Adopting an existing local route
*
* AWS creates certain routes that the AWS provider mostly ignores. You can manage them by importing or adopting them. See Import below for information on importing. This example shows adopting a route and then updating its target.
*
* First, adopt an existing AWS-created route:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.RouteTable;
* import com.pulumi.aws.ec2.RouteTableArgs;
* import com.pulumi.aws.ec2.inputs.RouteTableRouteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new Vpc("test", VpcArgs.builder()
* .cidrBlock("10.1.0.0/16")
* .build());
*
* var testRouteTable = new RouteTable("testRouteTable", RouteTableArgs.builder()
* .vpcId(test.id())
* .routes(RouteTableRouteArgs.builder()
* .cidrBlock("10.1.0.0/16")
* .gatewayId("local")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* Next, update the target of the route:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.Subnet;
* import com.pulumi.aws.ec2.SubnetArgs;
* import com.pulumi.aws.ec2.NetworkInterface;
* import com.pulumi.aws.ec2.NetworkInterfaceArgs;
* import com.pulumi.aws.ec2.RouteTable;
* import com.pulumi.aws.ec2.RouteTableArgs;
* import com.pulumi.aws.ec2.inputs.RouteTableRouteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new Vpc("test", VpcArgs.builder()
* .cidrBlock("10.1.0.0/16")
* .build());
*
* var testSubnet = new Subnet("testSubnet", SubnetArgs.builder()
* .cidrBlock("10.1.1.0/24")
* .vpcId(test.id())
* .build());
*
* var testNetworkInterface = new NetworkInterface("testNetworkInterface", NetworkInterfaceArgs.builder()
* .subnetId(testSubnet.id())
* .build());
*
* var testRouteTable = new RouteTable("testRouteTable", RouteTableArgs.builder()
* .vpcId(test.id())
* .routes(RouteTableRouteArgs.builder()
* .cidrBlock(test.cidrBlock())
* .networkInterfaceId(testNetworkInterface.id())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* The target could then be updated again back to `local`.
*
* ## Import
*
* Using `pulumi import`, import Route Tables using the route table `id`. For example:
*
* ```sh
* $ pulumi import aws:ec2/routeTable:RouteTable public_rt rtb-4e616f6d69
* ```
*
*/
@ResourceType(type="aws:ec2/routeTable:RouteTable")
public class RouteTable extends com.pulumi.resources.CustomResource {
/**
* The ARN of the route table.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the route table.
*
*/
public Output arn() {
return this.arn;
}
/**
* The ID of the AWS account that owns the route table.
*
*/
@Export(name="ownerId", refs={String.class}, tree="[0]")
private Output ownerId;
/**
* @return The ID of the AWS account that owns the route table.
*
*/
public Output ownerId() {
return this.ownerId;
}
/**
* A list of virtual gateways for propagation.
*
*/
@Export(name="propagatingVgws", refs={List.class,String.class}, tree="[0,1]")
private Output> propagatingVgws;
/**
* @return A list of virtual gateways for propagation.
*
*/
public Output> propagatingVgws() {
return this.propagatingVgws;
}
/**
* A list of route objects. Their keys are documented below.
* This means that omitting this argument is interpreted as ignoring any existing routes. To remove all managed routes an empty list should be specified. See the example above.
*
*/
@Export(name="routes", refs={List.class,RouteTableRoute.class}, tree="[0,1]")
private Output> routes;
/**
* @return A list of route objects. Their keys are documented below.
* This means that omitting this argument is interpreted as ignoring any existing routes. To remove all managed routes an empty list should be specified. See the example above.
*
*/
public Output> routes() {
return this.routes;
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy