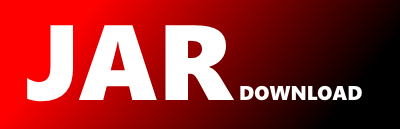
com.pulumi.aws.ec2.outputs.GetDedicatedHostResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.aws.ec2.outputs.GetDedicatedHostFilter;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetDedicatedHostResult {
/**
* @return ARN of the Dedicated Host.
*
*/
private String arn;
/**
* @return The ID of the Outpost hardware asset on which the Dedicated Host is allocated.
*
*/
private String assetId;
/**
* @return Whether auto-placement is on or off.
*
*/
private String autoPlacement;
/**
* @return Availability Zone of the Dedicated Host.
*
*/
private String availabilityZone;
/**
* @return Number of cores on the Dedicated Host.
*
*/
private Integer cores;
private @Nullable List filters;
private String hostId;
/**
* @return Whether host recovery is enabled or disabled for the Dedicated Host.
*
*/
private String hostRecovery;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Instance family supported by the Dedicated Host. For example, "m5".
*
*/
private String instanceFamily;
/**
* @return Instance type supported by the Dedicated Host. For example, "m5.large". If the host supports multiple instance types, no instanceType is returned.
*
*/
private String instanceType;
/**
* @return ARN of the AWS Outpost on which the Dedicated Host is allocated.
*
*/
private String outpostArn;
/**
* @return ID of the AWS account that owns the Dedicated Host.
*
*/
private String ownerId;
/**
* @return Number of sockets on the Dedicated Host.
*
*/
private Integer sockets;
private Map tags;
/**
* @return Total number of vCPUs on the Dedicated Host.
*
*/
private Integer totalVcpus;
private GetDedicatedHostResult() {}
/**
* @return ARN of the Dedicated Host.
*
*/
public String arn() {
return this.arn;
}
/**
* @return The ID of the Outpost hardware asset on which the Dedicated Host is allocated.
*
*/
public String assetId() {
return this.assetId;
}
/**
* @return Whether auto-placement is on or off.
*
*/
public String autoPlacement() {
return this.autoPlacement;
}
/**
* @return Availability Zone of the Dedicated Host.
*
*/
public String availabilityZone() {
return this.availabilityZone;
}
/**
* @return Number of cores on the Dedicated Host.
*
*/
public Integer cores() {
return this.cores;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
public String hostId() {
return this.hostId;
}
/**
* @return Whether host recovery is enabled or disabled for the Dedicated Host.
*
*/
public String hostRecovery() {
return this.hostRecovery;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Instance family supported by the Dedicated Host. For example, "m5".
*
*/
public String instanceFamily() {
return this.instanceFamily;
}
/**
* @return Instance type supported by the Dedicated Host. For example, "m5.large". If the host supports multiple instance types, no instanceType is returned.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return ARN of the AWS Outpost on which the Dedicated Host is allocated.
*
*/
public String outpostArn() {
return this.outpostArn;
}
/**
* @return ID of the AWS account that owns the Dedicated Host.
*
*/
public String ownerId() {
return this.ownerId;
}
/**
* @return Number of sockets on the Dedicated Host.
*
*/
public Integer sockets() {
return this.sockets;
}
public Map tags() {
return this.tags;
}
/**
* @return Total number of vCPUs on the Dedicated Host.
*
*/
public Integer totalVcpus() {
return this.totalVcpus;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDedicatedHostResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String assetId;
private String autoPlacement;
private String availabilityZone;
private Integer cores;
private @Nullable List filters;
private String hostId;
private String hostRecovery;
private String id;
private String instanceFamily;
private String instanceType;
private String outpostArn;
private String ownerId;
private Integer sockets;
private Map tags;
private Integer totalVcpus;
public Builder() {}
public Builder(GetDedicatedHostResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.assetId = defaults.assetId;
this.autoPlacement = defaults.autoPlacement;
this.availabilityZone = defaults.availabilityZone;
this.cores = defaults.cores;
this.filters = defaults.filters;
this.hostId = defaults.hostId;
this.hostRecovery = defaults.hostRecovery;
this.id = defaults.id;
this.instanceFamily = defaults.instanceFamily;
this.instanceType = defaults.instanceType;
this.outpostArn = defaults.outpostArn;
this.ownerId = defaults.ownerId;
this.sockets = defaults.sockets;
this.tags = defaults.tags;
this.totalVcpus = defaults.totalVcpus;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder assetId(String assetId) {
if (assetId == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "assetId");
}
this.assetId = assetId;
return this;
}
@CustomType.Setter
public Builder autoPlacement(String autoPlacement) {
if (autoPlacement == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "autoPlacement");
}
this.autoPlacement = autoPlacement;
return this;
}
@CustomType.Setter
public Builder availabilityZone(String availabilityZone) {
if (availabilityZone == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "availabilityZone");
}
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder cores(Integer cores) {
if (cores == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "cores");
}
this.cores = cores;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetDedicatedHostFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder hostId(String hostId) {
if (hostId == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "hostId");
}
this.hostId = hostId;
return this;
}
@CustomType.Setter
public Builder hostRecovery(String hostRecovery) {
if (hostRecovery == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "hostRecovery");
}
this.hostRecovery = hostRecovery;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder instanceFamily(String instanceFamily) {
if (instanceFamily == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "instanceFamily");
}
this.instanceFamily = instanceFamily;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder outpostArn(String outpostArn) {
if (outpostArn == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "outpostArn");
}
this.outpostArn = outpostArn;
return this;
}
@CustomType.Setter
public Builder ownerId(String ownerId) {
if (ownerId == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "ownerId");
}
this.ownerId = ownerId;
return this;
}
@CustomType.Setter
public Builder sockets(Integer sockets) {
if (sockets == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "sockets");
}
this.sockets = sockets;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder totalVcpus(Integer totalVcpus) {
if (totalVcpus == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "totalVcpus");
}
this.totalVcpus = totalVcpus;
return this;
}
public GetDedicatedHostResult build() {
final var _resultValue = new GetDedicatedHostResult();
_resultValue.arn = arn;
_resultValue.assetId = assetId;
_resultValue.autoPlacement = autoPlacement;
_resultValue.availabilityZone = availabilityZone;
_resultValue.cores = cores;
_resultValue.filters = filters;
_resultValue.hostId = hostId;
_resultValue.hostRecovery = hostRecovery;
_resultValue.id = id;
_resultValue.instanceFamily = instanceFamily;
_resultValue.instanceType = instanceType;
_resultValue.outpostArn = outpostArn;
_resultValue.ownerId = ownerId;
_resultValue.sockets = sockets;
_resultValue.tags = tags;
_resultValue.totalVcpus = totalVcpus;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy