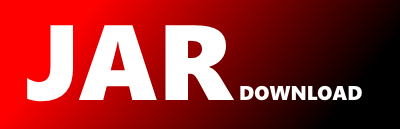
com.pulumi.aws.ec2.outputs.GetNetworkInterfaceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.aws.ec2.outputs.GetNetworkInterfaceAssociation;
import com.pulumi.aws.ec2.outputs.GetNetworkInterfaceAttachment;
import com.pulumi.aws.ec2.outputs.GetNetworkInterfaceFilter;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetNetworkInterfaceResult {
/**
* @return ARN of the network interface.
*
*/
private String arn;
/**
* @return Association information for an Elastic IP address (IPv4) associated with the network interface. See supported fields below.
*
*/
private List associations;
private List attachments;
/**
* @return Availability Zone.
*
*/
private String availabilityZone;
/**
* @return Description of the network interface.
*
*/
private String description;
private @Nullable List filters;
private String id;
/**
* @return Type of interface.
*
*/
private String interfaceType;
/**
* @return List of IPv6 addresses to assign to the ENI.
*
*/
private List ipv6Addresses;
/**
* @return MAC address.
*
*/
private String macAddress;
/**
* @return ARN of the Outpost.
*
*/
private String outpostArn;
/**
* @return AWS account ID of the owner of the network interface.
*
*/
private String ownerId;
/**
* @return Private DNS name.
*
*/
private String privateDnsName;
/**
* @return Private IPv4 address of the network interface within the subnet.
*
*/
private String privateIp;
/**
* @return Private IPv4 addresses associated with the network interface.
*
*/
private List privateIps;
/**
* @return ID of the entity that launched the instance on your behalf.
*
*/
private String requesterId;
/**
* @return List of security groups for the network interface.
*
*/
private List securityGroups;
/**
* @return ID of the subnet.
*
*/
private String subnetId;
/**
* @return Any tags assigned to the network interface.
*
*/
private Map tags;
/**
* @return ID of the VPC.
*
*/
private String vpcId;
private GetNetworkInterfaceResult() {}
/**
* @return ARN of the network interface.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Association information for an Elastic IP address (IPv4) associated with the network interface. See supported fields below.
*
*/
public List associations() {
return this.associations;
}
public List attachments() {
return this.attachments;
}
/**
* @return Availability Zone.
*
*/
public String availabilityZone() {
return this.availabilityZone;
}
/**
* @return Description of the network interface.
*
*/
public String description() {
return this.description;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
public String id() {
return this.id;
}
/**
* @return Type of interface.
*
*/
public String interfaceType() {
return this.interfaceType;
}
/**
* @return List of IPv6 addresses to assign to the ENI.
*
*/
public List ipv6Addresses() {
return this.ipv6Addresses;
}
/**
* @return MAC address.
*
*/
public String macAddress() {
return this.macAddress;
}
/**
* @return ARN of the Outpost.
*
*/
public String outpostArn() {
return this.outpostArn;
}
/**
* @return AWS account ID of the owner of the network interface.
*
*/
public String ownerId() {
return this.ownerId;
}
/**
* @return Private DNS name.
*
*/
public String privateDnsName() {
return this.privateDnsName;
}
/**
* @return Private IPv4 address of the network interface within the subnet.
*
*/
public String privateIp() {
return this.privateIp;
}
/**
* @return Private IPv4 addresses associated with the network interface.
*
*/
public List privateIps() {
return this.privateIps;
}
/**
* @return ID of the entity that launched the instance on your behalf.
*
*/
public String requesterId() {
return this.requesterId;
}
/**
* @return List of security groups for the network interface.
*
*/
public List securityGroups() {
return this.securityGroups;
}
/**
* @return ID of the subnet.
*
*/
public String subnetId() {
return this.subnetId;
}
/**
* @return Any tags assigned to the network interface.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return ID of the VPC.
*
*/
public String vpcId() {
return this.vpcId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetNetworkInterfaceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List associations;
private List attachments;
private String availabilityZone;
private String description;
private @Nullable List filters;
private String id;
private String interfaceType;
private List ipv6Addresses;
private String macAddress;
private String outpostArn;
private String ownerId;
private String privateDnsName;
private String privateIp;
private List privateIps;
private String requesterId;
private List securityGroups;
private String subnetId;
private Map tags;
private String vpcId;
public Builder() {}
public Builder(GetNetworkInterfaceResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.associations = defaults.associations;
this.attachments = defaults.attachments;
this.availabilityZone = defaults.availabilityZone;
this.description = defaults.description;
this.filters = defaults.filters;
this.id = defaults.id;
this.interfaceType = defaults.interfaceType;
this.ipv6Addresses = defaults.ipv6Addresses;
this.macAddress = defaults.macAddress;
this.outpostArn = defaults.outpostArn;
this.ownerId = defaults.ownerId;
this.privateDnsName = defaults.privateDnsName;
this.privateIp = defaults.privateIp;
this.privateIps = defaults.privateIps;
this.requesterId = defaults.requesterId;
this.securityGroups = defaults.securityGroups;
this.subnetId = defaults.subnetId;
this.tags = defaults.tags;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder associations(List associations) {
if (associations == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "associations");
}
this.associations = associations;
return this;
}
public Builder associations(GetNetworkInterfaceAssociation... associations) {
return associations(List.of(associations));
}
@CustomType.Setter
public Builder attachments(List attachments) {
if (attachments == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "attachments");
}
this.attachments = attachments;
return this;
}
public Builder attachments(GetNetworkInterfaceAttachment... attachments) {
return attachments(List.of(attachments));
}
@CustomType.Setter
public Builder availabilityZone(String availabilityZone) {
if (availabilityZone == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "availabilityZone");
}
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetNetworkInterfaceFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder interfaceType(String interfaceType) {
if (interfaceType == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "interfaceType");
}
this.interfaceType = interfaceType;
return this;
}
@CustomType.Setter
public Builder ipv6Addresses(List ipv6Addresses) {
if (ipv6Addresses == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "ipv6Addresses");
}
this.ipv6Addresses = ipv6Addresses;
return this;
}
public Builder ipv6Addresses(String... ipv6Addresses) {
return ipv6Addresses(List.of(ipv6Addresses));
}
@CustomType.Setter
public Builder macAddress(String macAddress) {
if (macAddress == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "macAddress");
}
this.macAddress = macAddress;
return this;
}
@CustomType.Setter
public Builder outpostArn(String outpostArn) {
if (outpostArn == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "outpostArn");
}
this.outpostArn = outpostArn;
return this;
}
@CustomType.Setter
public Builder ownerId(String ownerId) {
if (ownerId == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "ownerId");
}
this.ownerId = ownerId;
return this;
}
@CustomType.Setter
public Builder privateDnsName(String privateDnsName) {
if (privateDnsName == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "privateDnsName");
}
this.privateDnsName = privateDnsName;
return this;
}
@CustomType.Setter
public Builder privateIp(String privateIp) {
if (privateIp == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "privateIp");
}
this.privateIp = privateIp;
return this;
}
@CustomType.Setter
public Builder privateIps(List privateIps) {
if (privateIps == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "privateIps");
}
this.privateIps = privateIps;
return this;
}
public Builder privateIps(String... privateIps) {
return privateIps(List.of(privateIps));
}
@CustomType.Setter
public Builder requesterId(String requesterId) {
if (requesterId == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "requesterId");
}
this.requesterId = requesterId;
return this;
}
@CustomType.Setter
public Builder securityGroups(List securityGroups) {
if (securityGroups == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "securityGroups");
}
this.securityGroups = securityGroups;
return this;
}
public Builder securityGroups(String... securityGroups) {
return securityGroups(List.of(securityGroups));
}
@CustomType.Setter
public Builder subnetId(String subnetId) {
if (subnetId == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "subnetId");
}
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder vpcId(String vpcId) {
if (vpcId == null) {
throw new MissingRequiredPropertyException("GetNetworkInterfaceResult", "vpcId");
}
this.vpcId = vpcId;
return this;
}
public GetNetworkInterfaceResult build() {
final var _resultValue = new GetNetworkInterfaceResult();
_resultValue.arn = arn;
_resultValue.associations = associations;
_resultValue.attachments = attachments;
_resultValue.availabilityZone = availabilityZone;
_resultValue.description = description;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.interfaceType = interfaceType;
_resultValue.ipv6Addresses = ipv6Addresses;
_resultValue.macAddress = macAddress;
_resultValue.outpostArn = outpostArn;
_resultValue.ownerId = ownerId;
_resultValue.privateDnsName = privateDnsName;
_resultValue.privateIp = privateIp;
_resultValue.privateIps = privateIps;
_resultValue.requesterId = requesterId;
_resultValue.securityGroups = securityGroups;
_resultValue.subnetId = subnetId;
_resultValue.tags = tags;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy