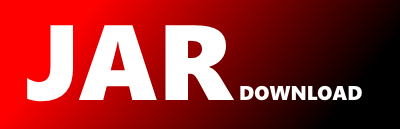
com.pulumi.aws.ec2.outputs.GetVpcIpamPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.aws.ec2.outputs.GetVpcIpamPoolFilter;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVpcIpamPoolResult {
/**
* @return IP protocol assigned to this pool.
*
*/
private String addressFamily;
/**
* @return A default netmask length for allocations added to this pool. If, for example, the CIDR assigned to this pool is `10.0.0.0/8` and you enter 16 here, new allocations will default to `10.0.0.0/16`.
*
*/
private Integer allocationDefaultNetmaskLength;
/**
* @return The maximum netmask length that will be required for CIDR allocations in this pool.
*
*/
private Integer allocationMaxNetmaskLength;
/**
* @return The minimum netmask length that will be required for CIDR allocations in this pool.
*
*/
private Integer allocationMinNetmaskLength;
/**
* @return Tags that are required to create resources in using this pool.
*
*/
private Map allocationResourceTags;
/**
* @return ARN of the pool
*
*/
private String arn;
/**
* @return If enabled, IPAM will continuously look for resources within the CIDR range of this pool and automatically import them as allocations into your IPAM.
*
*/
private Boolean autoImport;
/**
* @return Limits which service in AWS that the pool can be used in. `ec2` for example, allows users to use space for Elastic IP addresses and VPCs.
*
*/
private String awsService;
/**
* @return Description for the IPAM pool.
*
*/
private String description;
private @Nullable List filters;
/**
* @return ID of the IPAM pool.
*
*/
private @Nullable String id;
private @Nullable String ipamPoolId;
/**
* @return ID of the scope the pool belongs to.
*
*/
private String ipamScopeId;
private String ipamScopeType;
/**
* @return Locale is the Region where your pool is available for allocations. You can only create pools with locales that match the operating Regions of the IPAM. You can only create VPCs from a pool whose locale matches the VPC's Region.
*
*/
private String locale;
private Integer poolDepth;
/**
* @return Defines whether or not IPv6 pool space is publicly advertisable over the internet.
*
*/
private Boolean publiclyAdvertisable;
/**
* @return ID of the source IPAM pool.
*
*/
private String sourceIpamPoolId;
private String state;
/**
* @return Map of tags to assigned to the resource.
*
*/
private Map tags;
private GetVpcIpamPoolResult() {}
/**
* @return IP protocol assigned to this pool.
*
*/
public String addressFamily() {
return this.addressFamily;
}
/**
* @return A default netmask length for allocations added to this pool. If, for example, the CIDR assigned to this pool is `10.0.0.0/8` and you enter 16 here, new allocations will default to `10.0.0.0/16`.
*
*/
public Integer allocationDefaultNetmaskLength() {
return this.allocationDefaultNetmaskLength;
}
/**
* @return The maximum netmask length that will be required for CIDR allocations in this pool.
*
*/
public Integer allocationMaxNetmaskLength() {
return this.allocationMaxNetmaskLength;
}
/**
* @return The minimum netmask length that will be required for CIDR allocations in this pool.
*
*/
public Integer allocationMinNetmaskLength() {
return this.allocationMinNetmaskLength;
}
/**
* @return Tags that are required to create resources in using this pool.
*
*/
public Map allocationResourceTags() {
return this.allocationResourceTags;
}
/**
* @return ARN of the pool
*
*/
public String arn() {
return this.arn;
}
/**
* @return If enabled, IPAM will continuously look for resources within the CIDR range of this pool and automatically import them as allocations into your IPAM.
*
*/
public Boolean autoImport() {
return this.autoImport;
}
/**
* @return Limits which service in AWS that the pool can be used in. `ec2` for example, allows users to use space for Elastic IP addresses and VPCs.
*
*/
public String awsService() {
return this.awsService;
}
/**
* @return Description for the IPAM pool.
*
*/
public String description() {
return this.description;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
/**
* @return ID of the IPAM pool.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
public Optional ipamPoolId() {
return Optional.ofNullable(this.ipamPoolId);
}
/**
* @return ID of the scope the pool belongs to.
*
*/
public String ipamScopeId() {
return this.ipamScopeId;
}
public String ipamScopeType() {
return this.ipamScopeType;
}
/**
* @return Locale is the Region where your pool is available for allocations. You can only create pools with locales that match the operating Regions of the IPAM. You can only create VPCs from a pool whose locale matches the VPC's Region.
*
*/
public String locale() {
return this.locale;
}
public Integer poolDepth() {
return this.poolDepth;
}
/**
* @return Defines whether or not IPv6 pool space is publicly advertisable over the internet.
*
*/
public Boolean publiclyAdvertisable() {
return this.publiclyAdvertisable;
}
/**
* @return ID of the source IPAM pool.
*
*/
public String sourceIpamPoolId() {
return this.sourceIpamPoolId;
}
public String state() {
return this.state;
}
/**
* @return Map of tags to assigned to the resource.
*
*/
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVpcIpamPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String addressFamily;
private Integer allocationDefaultNetmaskLength;
private Integer allocationMaxNetmaskLength;
private Integer allocationMinNetmaskLength;
private Map allocationResourceTags;
private String arn;
private Boolean autoImport;
private String awsService;
private String description;
private @Nullable List filters;
private @Nullable String id;
private @Nullable String ipamPoolId;
private String ipamScopeId;
private String ipamScopeType;
private String locale;
private Integer poolDepth;
private Boolean publiclyAdvertisable;
private String sourceIpamPoolId;
private String state;
private Map tags;
public Builder() {}
public Builder(GetVpcIpamPoolResult defaults) {
Objects.requireNonNull(defaults);
this.addressFamily = defaults.addressFamily;
this.allocationDefaultNetmaskLength = defaults.allocationDefaultNetmaskLength;
this.allocationMaxNetmaskLength = defaults.allocationMaxNetmaskLength;
this.allocationMinNetmaskLength = defaults.allocationMinNetmaskLength;
this.allocationResourceTags = defaults.allocationResourceTags;
this.arn = defaults.arn;
this.autoImport = defaults.autoImport;
this.awsService = defaults.awsService;
this.description = defaults.description;
this.filters = defaults.filters;
this.id = defaults.id;
this.ipamPoolId = defaults.ipamPoolId;
this.ipamScopeId = defaults.ipamScopeId;
this.ipamScopeType = defaults.ipamScopeType;
this.locale = defaults.locale;
this.poolDepth = defaults.poolDepth;
this.publiclyAdvertisable = defaults.publiclyAdvertisable;
this.sourceIpamPoolId = defaults.sourceIpamPoolId;
this.state = defaults.state;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder addressFamily(String addressFamily) {
if (addressFamily == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "addressFamily");
}
this.addressFamily = addressFamily;
return this;
}
@CustomType.Setter
public Builder allocationDefaultNetmaskLength(Integer allocationDefaultNetmaskLength) {
if (allocationDefaultNetmaskLength == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "allocationDefaultNetmaskLength");
}
this.allocationDefaultNetmaskLength = allocationDefaultNetmaskLength;
return this;
}
@CustomType.Setter
public Builder allocationMaxNetmaskLength(Integer allocationMaxNetmaskLength) {
if (allocationMaxNetmaskLength == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "allocationMaxNetmaskLength");
}
this.allocationMaxNetmaskLength = allocationMaxNetmaskLength;
return this;
}
@CustomType.Setter
public Builder allocationMinNetmaskLength(Integer allocationMinNetmaskLength) {
if (allocationMinNetmaskLength == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "allocationMinNetmaskLength");
}
this.allocationMinNetmaskLength = allocationMinNetmaskLength;
return this;
}
@CustomType.Setter
public Builder allocationResourceTags(Map allocationResourceTags) {
if (allocationResourceTags == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "allocationResourceTags");
}
this.allocationResourceTags = allocationResourceTags;
return this;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder autoImport(Boolean autoImport) {
if (autoImport == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "autoImport");
}
this.autoImport = autoImport;
return this;
}
@CustomType.Setter
public Builder awsService(String awsService) {
if (awsService == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "awsService");
}
this.awsService = awsService;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetVpcIpamPoolFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipamPoolId(@Nullable String ipamPoolId) {
this.ipamPoolId = ipamPoolId;
return this;
}
@CustomType.Setter
public Builder ipamScopeId(String ipamScopeId) {
if (ipamScopeId == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "ipamScopeId");
}
this.ipamScopeId = ipamScopeId;
return this;
}
@CustomType.Setter
public Builder ipamScopeType(String ipamScopeType) {
if (ipamScopeType == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "ipamScopeType");
}
this.ipamScopeType = ipamScopeType;
return this;
}
@CustomType.Setter
public Builder locale(String locale) {
if (locale == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "locale");
}
this.locale = locale;
return this;
}
@CustomType.Setter
public Builder poolDepth(Integer poolDepth) {
if (poolDepth == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "poolDepth");
}
this.poolDepth = poolDepth;
return this;
}
@CustomType.Setter
public Builder publiclyAdvertisable(Boolean publiclyAdvertisable) {
if (publiclyAdvertisable == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "publiclyAdvertisable");
}
this.publiclyAdvertisable = publiclyAdvertisable;
return this;
}
@CustomType.Setter
public Builder sourceIpamPoolId(String sourceIpamPoolId) {
if (sourceIpamPoolId == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "sourceIpamPoolId");
}
this.sourceIpamPoolId = sourceIpamPoolId;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetVpcIpamPoolResult", "tags");
}
this.tags = tags;
return this;
}
public GetVpcIpamPoolResult build() {
final var _resultValue = new GetVpcIpamPoolResult();
_resultValue.addressFamily = addressFamily;
_resultValue.allocationDefaultNetmaskLength = allocationDefaultNetmaskLength;
_resultValue.allocationMaxNetmaskLength = allocationMaxNetmaskLength;
_resultValue.allocationMinNetmaskLength = allocationMinNetmaskLength;
_resultValue.allocationResourceTags = allocationResourceTags;
_resultValue.arn = arn;
_resultValue.autoImport = autoImport;
_resultValue.awsService = awsService;
_resultValue.description = description;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.ipamPoolId = ipamPoolId;
_resultValue.ipamScopeId = ipamScopeId;
_resultValue.ipamScopeType = ipamScopeType;
_resultValue.locale = locale;
_resultValue.poolDepth = poolDepth;
_resultValue.publiclyAdvertisable = publiclyAdvertisable;
_resultValue.sourceIpamPoolId = sourceIpamPoolId;
_resultValue.state = state;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy