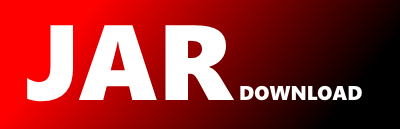
com.pulumi.aws.ec2.outputs.NetworkAclIngress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NetworkAclIngress {
/**
* @return The action to take.
*
*/
private String action;
/**
* @return The CIDR block to match. This must be a
* valid network mask.
*
*/
private @Nullable String cidrBlock;
/**
* @return The from port to match.
*
*/
private Integer fromPort;
/**
* @return The ICMP type code to be used. Default 0.
*
* > Note: For more information on ICMP types and codes, see here: https://www.iana.org/assignments/icmp-parameters/icmp-parameters.xhtml
*
*/
private @Nullable Integer icmpCode;
/**
* @return The ICMP type to be used. Default 0.
*
*/
private @Nullable Integer icmpType;
/**
* @return The IPv6 CIDR block.
*
*/
private @Nullable String ipv6CidrBlock;
/**
* @return The protocol to match. If using the -1 'all'
* protocol, you must specify a from and to port of 0.
*
*/
private String protocol;
/**
* @return The rule number. Used for ordering.
*
*/
private Integer ruleNo;
/**
* @return The to port to match.
*
*/
private Integer toPort;
private NetworkAclIngress() {}
/**
* @return The action to take.
*
*/
public String action() {
return this.action;
}
/**
* @return The CIDR block to match. This must be a
* valid network mask.
*
*/
public Optional cidrBlock() {
return Optional.ofNullable(this.cidrBlock);
}
/**
* @return The from port to match.
*
*/
public Integer fromPort() {
return this.fromPort;
}
/**
* @return The ICMP type code to be used. Default 0.
*
* > Note: For more information on ICMP types and codes, see here: https://www.iana.org/assignments/icmp-parameters/icmp-parameters.xhtml
*
*/
public Optional icmpCode() {
return Optional.ofNullable(this.icmpCode);
}
/**
* @return The ICMP type to be used. Default 0.
*
*/
public Optional icmpType() {
return Optional.ofNullable(this.icmpType);
}
/**
* @return The IPv6 CIDR block.
*
*/
public Optional ipv6CidrBlock() {
return Optional.ofNullable(this.ipv6CidrBlock);
}
/**
* @return The protocol to match. If using the -1 'all'
* protocol, you must specify a from and to port of 0.
*
*/
public String protocol() {
return this.protocol;
}
/**
* @return The rule number. Used for ordering.
*
*/
public Integer ruleNo() {
return this.ruleNo;
}
/**
* @return The to port to match.
*
*/
public Integer toPort() {
return this.toPort;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NetworkAclIngress defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String action;
private @Nullable String cidrBlock;
private Integer fromPort;
private @Nullable Integer icmpCode;
private @Nullable Integer icmpType;
private @Nullable String ipv6CidrBlock;
private String protocol;
private Integer ruleNo;
private Integer toPort;
public Builder() {}
public Builder(NetworkAclIngress defaults) {
Objects.requireNonNull(defaults);
this.action = defaults.action;
this.cidrBlock = defaults.cidrBlock;
this.fromPort = defaults.fromPort;
this.icmpCode = defaults.icmpCode;
this.icmpType = defaults.icmpType;
this.ipv6CidrBlock = defaults.ipv6CidrBlock;
this.protocol = defaults.protocol;
this.ruleNo = defaults.ruleNo;
this.toPort = defaults.toPort;
}
@CustomType.Setter
public Builder action(String action) {
if (action == null) {
throw new MissingRequiredPropertyException("NetworkAclIngress", "action");
}
this.action = action;
return this;
}
@CustomType.Setter
public Builder cidrBlock(@Nullable String cidrBlock) {
this.cidrBlock = cidrBlock;
return this;
}
@CustomType.Setter
public Builder fromPort(Integer fromPort) {
if (fromPort == null) {
throw new MissingRequiredPropertyException("NetworkAclIngress", "fromPort");
}
this.fromPort = fromPort;
return this;
}
@CustomType.Setter
public Builder icmpCode(@Nullable Integer icmpCode) {
this.icmpCode = icmpCode;
return this;
}
@CustomType.Setter
public Builder icmpType(@Nullable Integer icmpType) {
this.icmpType = icmpType;
return this;
}
@CustomType.Setter
public Builder ipv6CidrBlock(@Nullable String ipv6CidrBlock) {
this.ipv6CidrBlock = ipv6CidrBlock;
return this;
}
@CustomType.Setter
public Builder protocol(String protocol) {
if (protocol == null) {
throw new MissingRequiredPropertyException("NetworkAclIngress", "protocol");
}
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder ruleNo(Integer ruleNo) {
if (ruleNo == null) {
throw new MissingRequiredPropertyException("NetworkAclIngress", "ruleNo");
}
this.ruleNo = ruleNo;
return this;
}
@CustomType.Setter
public Builder toPort(Integer toPort) {
if (toPort == null) {
throw new MissingRequiredPropertyException("NetworkAclIngress", "toPort");
}
this.toPort = toPort;
return this;
}
public NetworkAclIngress build() {
final var _resultValue = new NetworkAclIngress();
_resultValue.action = action;
_resultValue.cidrBlock = cidrBlock;
_resultValue.fromPort = fromPort;
_resultValue.icmpCode = icmpCode;
_resultValue.icmpType = icmpType;
_resultValue.ipv6CidrBlock = ipv6CidrBlock;
_resultValue.protocol = protocol;
_resultValue.ruleNo = ruleNo;
_resultValue.toPort = toPort;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy