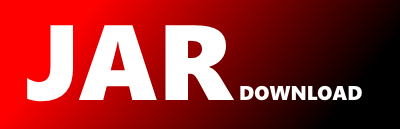
com.pulumi.aws.ec2clientvpn.outputs.GetEndpointResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2clientvpn.outputs;
import com.pulumi.aws.ec2clientvpn.outputs.GetEndpointAuthenticationOption;
import com.pulumi.aws.ec2clientvpn.outputs.GetEndpointClientConnectOption;
import com.pulumi.aws.ec2clientvpn.outputs.GetEndpointClientLoginBannerOption;
import com.pulumi.aws.ec2clientvpn.outputs.GetEndpointConnectionLogOption;
import com.pulumi.aws.ec2clientvpn.outputs.GetEndpointFilter;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetEndpointResult {
/**
* @return The ARN of the Client VPN endpoint.
*
*/
private String arn;
/**
* @return Information about the authentication method used by the Client VPN endpoint.
*
*/
private List authenticationOptions;
/**
* @return IPv4 address range, in CIDR notation, from which client IP addresses are assigned.
*
*/
private String clientCidrBlock;
/**
* @return The options for managing connection authorization for new client connections.
*
*/
private List clientConnectOptions;
/**
* @return Options for enabling a customizable text banner that will be displayed on AWS provided clients when a VPN session is established.
*
*/
private List clientLoginBannerOptions;
private String clientVpnEndpointId;
/**
* @return Information about the client connection logging options for the Client VPN endpoint.
*
*/
private List connectionLogOptions;
/**
* @return Brief description of the endpoint.
*
*/
private String description;
/**
* @return DNS name to be used by clients when connecting to the Client VPN endpoint.
*
*/
private String dnsName;
/**
* @return Information about the DNS servers to be used for DNS resolution.
*
*/
private List dnsServers;
private @Nullable List filters;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return IDs of the security groups for the target network associated with the Client VPN endpoint.
*
*/
private List securityGroupIds;
/**
* @return Whether the self-service portal for the Client VPN endpoint is enabled.
*
*/
private String selfServicePortal;
/**
* @return The URL of the self-service portal.
*
*/
private String selfServicePortalUrl;
/**
* @return The ARN of the server certificate.
*
*/
private String serverCertificateArn;
/**
* @return The maximum VPN session duration time in hours.
*
*/
private Integer sessionTimeoutHours;
/**
* @return Whether split-tunnel is enabled in the AWS Client VPN endpoint.
*
*/
private Boolean splitTunnel;
private Map tags;
/**
* @return Transport protocol used by the Client VPN endpoint.
*
*/
private String transportProtocol;
/**
* @return ID of the VPC associated with the Client VPN endpoint.
*
*/
private String vpcId;
/**
* @return Port number for the Client VPN endpoint.
*
*/
private Integer vpnPort;
private GetEndpointResult() {}
/**
* @return The ARN of the Client VPN endpoint.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Information about the authentication method used by the Client VPN endpoint.
*
*/
public List authenticationOptions() {
return this.authenticationOptions;
}
/**
* @return IPv4 address range, in CIDR notation, from which client IP addresses are assigned.
*
*/
public String clientCidrBlock() {
return this.clientCidrBlock;
}
/**
* @return The options for managing connection authorization for new client connections.
*
*/
public List clientConnectOptions() {
return this.clientConnectOptions;
}
/**
* @return Options for enabling a customizable text banner that will be displayed on AWS provided clients when a VPN session is established.
*
*/
public List clientLoginBannerOptions() {
return this.clientLoginBannerOptions;
}
public String clientVpnEndpointId() {
return this.clientVpnEndpointId;
}
/**
* @return Information about the client connection logging options for the Client VPN endpoint.
*
*/
public List connectionLogOptions() {
return this.connectionLogOptions;
}
/**
* @return Brief description of the endpoint.
*
*/
public String description() {
return this.description;
}
/**
* @return DNS name to be used by clients when connecting to the Client VPN endpoint.
*
*/
public String dnsName() {
return this.dnsName;
}
/**
* @return Information about the DNS servers to be used for DNS resolution.
*
*/
public List dnsServers() {
return this.dnsServers;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return IDs of the security groups for the target network associated with the Client VPN endpoint.
*
*/
public List securityGroupIds() {
return this.securityGroupIds;
}
/**
* @return Whether the self-service portal for the Client VPN endpoint is enabled.
*
*/
public String selfServicePortal() {
return this.selfServicePortal;
}
/**
* @return The URL of the self-service portal.
*
*/
public String selfServicePortalUrl() {
return this.selfServicePortalUrl;
}
/**
* @return The ARN of the server certificate.
*
*/
public String serverCertificateArn() {
return this.serverCertificateArn;
}
/**
* @return The maximum VPN session duration time in hours.
*
*/
public Integer sessionTimeoutHours() {
return this.sessionTimeoutHours;
}
/**
* @return Whether split-tunnel is enabled in the AWS Client VPN endpoint.
*
*/
public Boolean splitTunnel() {
return this.splitTunnel;
}
public Map tags() {
return this.tags;
}
/**
* @return Transport protocol used by the Client VPN endpoint.
*
*/
public String transportProtocol() {
return this.transportProtocol;
}
/**
* @return ID of the VPC associated with the Client VPN endpoint.
*
*/
public String vpcId() {
return this.vpcId;
}
/**
* @return Port number for the Client VPN endpoint.
*
*/
public Integer vpnPort() {
return this.vpnPort;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEndpointResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List authenticationOptions;
private String clientCidrBlock;
private List clientConnectOptions;
private List clientLoginBannerOptions;
private String clientVpnEndpointId;
private List connectionLogOptions;
private String description;
private String dnsName;
private List dnsServers;
private @Nullable List filters;
private String id;
private List securityGroupIds;
private String selfServicePortal;
private String selfServicePortalUrl;
private String serverCertificateArn;
private Integer sessionTimeoutHours;
private Boolean splitTunnel;
private Map tags;
private String transportProtocol;
private String vpcId;
private Integer vpnPort;
public Builder() {}
public Builder(GetEndpointResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.authenticationOptions = defaults.authenticationOptions;
this.clientCidrBlock = defaults.clientCidrBlock;
this.clientConnectOptions = defaults.clientConnectOptions;
this.clientLoginBannerOptions = defaults.clientLoginBannerOptions;
this.clientVpnEndpointId = defaults.clientVpnEndpointId;
this.connectionLogOptions = defaults.connectionLogOptions;
this.description = defaults.description;
this.dnsName = defaults.dnsName;
this.dnsServers = defaults.dnsServers;
this.filters = defaults.filters;
this.id = defaults.id;
this.securityGroupIds = defaults.securityGroupIds;
this.selfServicePortal = defaults.selfServicePortal;
this.selfServicePortalUrl = defaults.selfServicePortalUrl;
this.serverCertificateArn = defaults.serverCertificateArn;
this.sessionTimeoutHours = defaults.sessionTimeoutHours;
this.splitTunnel = defaults.splitTunnel;
this.tags = defaults.tags;
this.transportProtocol = defaults.transportProtocol;
this.vpcId = defaults.vpcId;
this.vpnPort = defaults.vpnPort;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder authenticationOptions(List authenticationOptions) {
if (authenticationOptions == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "authenticationOptions");
}
this.authenticationOptions = authenticationOptions;
return this;
}
public Builder authenticationOptions(GetEndpointAuthenticationOption... authenticationOptions) {
return authenticationOptions(List.of(authenticationOptions));
}
@CustomType.Setter
public Builder clientCidrBlock(String clientCidrBlock) {
if (clientCidrBlock == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "clientCidrBlock");
}
this.clientCidrBlock = clientCidrBlock;
return this;
}
@CustomType.Setter
public Builder clientConnectOptions(List clientConnectOptions) {
if (clientConnectOptions == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "clientConnectOptions");
}
this.clientConnectOptions = clientConnectOptions;
return this;
}
public Builder clientConnectOptions(GetEndpointClientConnectOption... clientConnectOptions) {
return clientConnectOptions(List.of(clientConnectOptions));
}
@CustomType.Setter
public Builder clientLoginBannerOptions(List clientLoginBannerOptions) {
if (clientLoginBannerOptions == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "clientLoginBannerOptions");
}
this.clientLoginBannerOptions = clientLoginBannerOptions;
return this;
}
public Builder clientLoginBannerOptions(GetEndpointClientLoginBannerOption... clientLoginBannerOptions) {
return clientLoginBannerOptions(List.of(clientLoginBannerOptions));
}
@CustomType.Setter
public Builder clientVpnEndpointId(String clientVpnEndpointId) {
if (clientVpnEndpointId == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "clientVpnEndpointId");
}
this.clientVpnEndpointId = clientVpnEndpointId;
return this;
}
@CustomType.Setter
public Builder connectionLogOptions(List connectionLogOptions) {
if (connectionLogOptions == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "connectionLogOptions");
}
this.connectionLogOptions = connectionLogOptions;
return this;
}
public Builder connectionLogOptions(GetEndpointConnectionLogOption... connectionLogOptions) {
return connectionLogOptions(List.of(connectionLogOptions));
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder dnsName(String dnsName) {
if (dnsName == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "dnsName");
}
this.dnsName = dnsName;
return this;
}
@CustomType.Setter
public Builder dnsServers(List dnsServers) {
if (dnsServers == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "dnsServers");
}
this.dnsServers = dnsServers;
return this;
}
public Builder dnsServers(String... dnsServers) {
return dnsServers(List.of(dnsServers));
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetEndpointFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder securityGroupIds(List securityGroupIds) {
if (securityGroupIds == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "securityGroupIds");
}
this.securityGroupIds = securityGroupIds;
return this;
}
public Builder securityGroupIds(String... securityGroupIds) {
return securityGroupIds(List.of(securityGroupIds));
}
@CustomType.Setter
public Builder selfServicePortal(String selfServicePortal) {
if (selfServicePortal == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "selfServicePortal");
}
this.selfServicePortal = selfServicePortal;
return this;
}
@CustomType.Setter
public Builder selfServicePortalUrl(String selfServicePortalUrl) {
if (selfServicePortalUrl == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "selfServicePortalUrl");
}
this.selfServicePortalUrl = selfServicePortalUrl;
return this;
}
@CustomType.Setter
public Builder serverCertificateArn(String serverCertificateArn) {
if (serverCertificateArn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "serverCertificateArn");
}
this.serverCertificateArn = serverCertificateArn;
return this;
}
@CustomType.Setter
public Builder sessionTimeoutHours(Integer sessionTimeoutHours) {
if (sessionTimeoutHours == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "sessionTimeoutHours");
}
this.sessionTimeoutHours = sessionTimeoutHours;
return this;
}
@CustomType.Setter
public Builder splitTunnel(Boolean splitTunnel) {
if (splitTunnel == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "splitTunnel");
}
this.splitTunnel = splitTunnel;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder transportProtocol(String transportProtocol) {
if (transportProtocol == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "transportProtocol");
}
this.transportProtocol = transportProtocol;
return this;
}
@CustomType.Setter
public Builder vpcId(String vpcId) {
if (vpcId == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "vpcId");
}
this.vpcId = vpcId;
return this;
}
@CustomType.Setter
public Builder vpnPort(Integer vpnPort) {
if (vpnPort == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "vpnPort");
}
this.vpnPort = vpnPort;
return this;
}
public GetEndpointResult build() {
final var _resultValue = new GetEndpointResult();
_resultValue.arn = arn;
_resultValue.authenticationOptions = authenticationOptions;
_resultValue.clientCidrBlock = clientCidrBlock;
_resultValue.clientConnectOptions = clientConnectOptions;
_resultValue.clientLoginBannerOptions = clientLoginBannerOptions;
_resultValue.clientVpnEndpointId = clientVpnEndpointId;
_resultValue.connectionLogOptions = connectionLogOptions;
_resultValue.description = description;
_resultValue.dnsName = dnsName;
_resultValue.dnsServers = dnsServers;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.securityGroupIds = securityGroupIds;
_resultValue.selfServicePortal = selfServicePortal;
_resultValue.selfServicePortalUrl = selfServicePortalUrl;
_resultValue.serverCertificateArn = serverCertificateArn;
_resultValue.sessionTimeoutHours = sessionTimeoutHours;
_resultValue.splitTunnel = splitTunnel;
_resultValue.tags = tags;
_resultValue.transportProtocol = transportProtocol;
_resultValue.vpcId = vpcId;
_resultValue.vpnPort = vpnPort;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy